Question
C++ Programming, Please help me fix errors In this program you will use the stack class you created in Assignment 1. First you will create
C++ Programming, Please help me fix errors
In this program you will use the stack class you created in Assignment 1. First you will create a class called InventoryItem. This class will have its class declaration in InventoryItem.h and its implementation in InventoryItem.cpp. It will have three private data members, an integer serialNum which holds the parts serial number, manufactDate which should be a string that holds the date the item was manufactured, then lotNum which will be an integer that holds the parts lot number. The program should then create a stack with a data type of InventoryItem (stack). The program should loop asking the user to enter in new items to the inventory stack or to remove an item from the inventory stack. The loop should continue until the user indicates they are done. This should be menu driven. When adding an item, the program should ask the user for the information it needs for the 3 data members of the InventoryItem class and add a new item to the stack. When removing an item from the stack, the program should display all of the information in the InventoryItem object that was popped off the stack. When the program ends, it should pop all of the remaining items off the stack and display the data that is in the Inventory items as it pops them off. There should be 3 utility functions that main uses.
void popItem(DynStack* stack) // pops the item off the stack and displays it.
void pushItem(DynStack* stack) // pushes the item onto the stack
int menu(); // displays the menu and returns the users choice.
This is what I have so far...
//For DynStack.h
#pragma once
/* This file contains the decleration of DynStack class */
// Declaration file for the DynStack class. This class is a template version of
// a dynamic stack class.
#ifndef DYNSTACK_H
#define DYNSTACK_H
template
class DynStack
{
private:
// Struct for stack nodes
struct StackNode {
T value; // holds the node's value
StackNode *next; // ptr to next node
};
StackNode *top; // ptr to stack top
public:
// Constructor
DynStack();
// Destructor
~DynStack();
// Stack operations
void push(T);
bool pop(T &);
bool isEmpty();
void displayStack() const;
};
#endif
//For DynStack.cpp
// This file contains Implementation for the DynStack class. This class is a template version of
// a dynamic stack class.
#include "DynStack.h"
// Default constructor
template
DynStack::DynStack()
{
top = nullptr;
}
// Destructor - deletes the stack node by node
template
DynStack::~DynStack()
{
StackNode *nodePtr;
StackNode *nextNode;
// aim nodePtr at top of stack
nodePtr = top;
// travel list and delete each node
while (nodePtr != nullptr)
{
nextNode = nodePtr->next;
delete nodePtr;
nodePtr = nextNode;
}
}
///////////////////////
// Stack operations //
/////////////////////
// push() adds the argument onto the stack
template
void DynStack::push(T item)
{
StackNode *newNode = nullptr;
// Creates a new node and stores argument there
newNode = new StackNode;
newNode->value = item;
// If the list is empty, make newNode the first node
if (isEmpty() == true)
{
top = newNode;
newNode->next = nullptr;
}
else
{
newNode->next = top;
top = newNode;
}
}
// If the stack is empty, then pop() simply returns false.
// If a node exists, pop() returns the top item, deletes it from the stack,
// and then returns true.
template
bool DynStack::pop(T &item)
{
StackNode *temp = nullptr;
bool status;
// Check that the stack isn't empty
if (isEmpty() == true)
{
status = false;
}
else // Pop value off top of stack
{
item = top->value;
temp = top->next;
delete top;
top = temp;
status = true;
}
return status;
}
// isEmpty() returns true if stack is empty; otherwise it returns false.
template
bool DynStack::isEmpty()
{
bool status;
if (!top)
status = true;
else
status = false;
return status;
}
// displayStack() simply prints each item in a given stack.
template
void DynStack::displayStack() const
{
StackNode *nodePtr;
nodePtr = top;
if (nodePtr == nullptr)
cout << "Stack is empty.";
else
{
while (nodePtr)
{
cout << nodePtr->value << endl;
nodePtr = nodePtr->next;
}
}
}
//For InventoryItem.h
#pragma once
#ifndef INVENTORYITEM_H
#define INVENTORYITEM_H
#include // Needed for strlen and strcpy
// Constant for the description's default size
const int DEFAULT_SIZE = 51;
class InventoryItem
{
private:
char *description; // The item description
double cost; // The item cost
int units; // Number of units on hand
// Private member function.
void createDescription(int size, char *value);
public:
// Constructor #1
InventoryItem();
// Constructor #2
InventoryItem(char *desc);
// Constructor #3
InventoryItem(char *desc, double c, int u);
// Destructor
~InventoryItem();
// Mutator functions
void setDescription(char *d);
void setCost(double c);
void setUnits(int u);
// Accessor functions
const char *getDescription() const;
double getCost() const;
int getUnits() const;
};
#endif
//For InventoryItem.cpp
#include "InventoryItem.h"
// Private member function.
void InventoryItem::createDescription(int size, char *value)
{
// Allocate the default amount of memory for description.
description = new char[size + 1];
// Store a value in the memory.
strcpy_s(description, size + 1, value);
}
// Constructor #1
InventoryItem::InventoryItem()
{
// Store an empty string in the description
// attribute.
createDescription(DEFAULT_SIZE, "");
// Initialize cost and units.
cost = 0.0;
units = 0;
}
// Constructor #2
InventoryItem::InventoryItem(char *desc)
{
// Allocate memory and store the description.
createDescription(strlen(desc), desc);
// Initialize cost and units.
cost = 0.0;
units = 0;
}
// Constructor #3
InventoryItem::InventoryItem(char *desc, double c, int u)
{
// Allocate memory and store the description.
createDescription(strlen(desc), desc);
// Assign values to cost and units.
cost = c;
units = u;
}
// Destructor
InventoryItem::~InventoryItem()
{
delete[] description;
}
// Mutator functions
void InventoryItem::setDescription(char *d)
{
if (strlen(description) > 0)
delete[] description;
createDescription(strlen(d), d);
}
void InventoryItem::setCost(double c)
{
cost = c;
}
void InventoryItem::setUnits(int u)
{
units = u;
}
// Accessor functions
const char *InventoryItem::getDescription() const
{
return description;
}
double InventoryItem::getCost() const
{
return cost;
}
int InventoryItem::getUnits() const
{
return units;
}
//For Main.cpp
#include "DynStack.h" #include "InventoryItem.h" #include
using namespace std;
void popItem(DynStack*); // pops the item off the stack and displays it. //calls pop() from DynStack within, snags the item info, and prints it void pushItem(DynStack*); // pushes the item onto the stack //calls push() from DynStack within, smushes item onto stack int menu(); // displays the menu and returns the users choice.
int main() { DynStack* invStack; invStack = new DynStack;
// Holds the menu selection from menu() int menuChoice = 0;
// Variable for menu loop bool valid = true;
// Values for switch statement const int ADDITEM = 1; const int REMITEM = 2; const int EXIT = 3;
// Used when printing out contents upon program exit InventoryItem temp;
while (valid) { menuChoice = menu(); switch (menuChoice) { case ADDITEM: // to add items to the stack pushItem(invStack); valid = true; break;
case REMITEM: // to remove items from the stack and display their contents popItem(invStack); valid = true; break;
case EXIT: // On exit, any inventory must be popped off the stack and // printed, in sequence. Otherwise the program simply ends.
if (invStack->isEmpty() != true) { cout << "Here are the remaining items in inventory: "; while (invStack->pop(temp) == true) // Loop runs until stack is empty { cout << "Cost: " << temp.getCost() << " "; cout << "Unit: " << temp.getUnits() << " "; cout << "Description: " << temp.getDescription() << " "; } // Display msg once all nodes are popped off if (invStack->isEmpty() == true) cout << "No further inventory. "; } else cout << "No inventory to display; exiting program. ";
return 0; } }
return 0; }
// popItem() is used to remove items from the stack and display the contents // to the user. If the stack has an item, it will use DynStack's pop() function // to populate the InventoryItem temp obj's data members, then print that data. // If the stack is empty, it returns an error and goes back to the menu screen. void popItem(DynStack* stack) { InventoryItem temp; char repeat = 'y'; // to control our loop
// While loop repeats until user is done removing items. while (repeat == 'y' || repeat == 'Y') { if (stack->pop(temp) == true) { cout << " ------------------------- "; cout << "| Remove Item | "; cout << "------------------------- "; cout << "Cost: " << temp.getCost() << " "; cout << "Units: " << temp.getUnits() << " "; cout << "Description: " << temp.getDescription() << " "; cout << "Remove and display another item y/n? "; cin >> repeat; } else // If stack is empty, display error message { cout << "No items in inventory. "; repeat = 'n'; // Set repeat to n to return to main menu } } }
// pushItem() asks the user for input for the lot number, serial number and // manufacturing date for each item. It then writes those to the temp // InventoryItem object, which is used with DynStack's push() function to // add the new item to the inventory. void pushItem(DynStack* stack) // pushes the item onto the stack { InventoryItem temp; int cost, unit; string description; char repeat = 'y'; // to control our loop
while (repeat == 'y' || repeat == 'Y') { cout << " ------------------------- "; cout << "| Add Item | "; cout << "------------------------- "; cout << "Enter Cost of the Item: ";
// Verify input so it is a non-zero number on lot num and serial num cin >> cost; while (cost < 0) { cout << "Cost should not be negative. "; cout << "Enter Cost Again: "; cin >> cost; }
cout << "Enter Unit: "; cin >> unit; while (unit < 0) { cout << "Units must not be -ve. "; cout << "Enter Unit: "; cin >> unit; }
cout << "Enter Description of the Item: "; cin >> description;
temp.setCost(cost); temp.setUnits(unit); temp.setDescription(description);
stack->push(temp);
cout << "Add another item to inventory y/n? "; cin >> repeat; } }
int menu() // displays the menu and returns the users choice. { int choice;
cout << "------------------------- "; cout << "| Inventory item Menu | "; cout << "------------------------- "; cout << "1) Add item to the Inventory "; cout << "2) Remove item from Inventory "; cout << "3) Exit program "; cout << "Selection number: "; cin >> choice;
// Check that it's a valid choice while (choice <= 0 || choice > 3) { cout << "Invalid choice. Pick 1, 2, or 3: "; cin >> choice; }
return choice; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
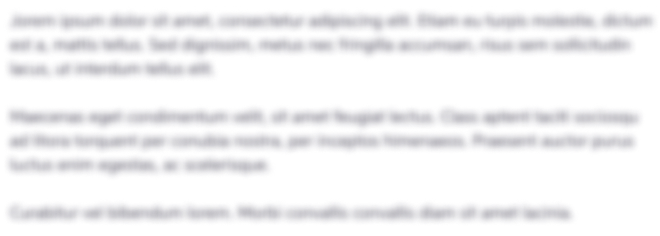
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started