Question
C Programming: Please post the test cases and output's screenshot qotd.c file #include qotd.h static qotd_t *qotd = NULL; static size_t count = 0; static
C Programming:
Please post the test cases and output's screenshot
qotd.c file
#include "qotd.h"
static qotd_t *qotd = NULL;
static size_t count = 0;
static char *buffer = NULL;
void freePointer(void *ptr)
{
/*
*
* 1. Write a short routine to clear *ptr.
* 2. Use freePointer() throughout the code
* to remove all memory leaks.
*
*/
}
buffersize_t getBufferSize(ssize_t new_size)
{ /* Calculate a new buffer size, being
* sure to prevent against integer overflow
* and/or wrap.
*/
return 0;
}
int addQuote(uint16_t pos, uint16_t len)
{
qotd = (qotd_t*)realloc(qotd, sizeof(qotd_t) * (count + 1));
if (qotd == NULL)
{
return ERROR_CONDITION;
}
qotd[count].pos = pos;
qotd[count].len = len;
count++;
return SUCCESS;
}
void quoteOfTheDay()
{
char *str = NULL;
srand((unsigned)time(NULL));
uint16_t index = ((rand() % count) + 1) - 1;
str = malloc(qotd[index].len + 1);
if (str == NULL)
{
fprintf(stderr, "quoteOfTheDay() %s", ERROR_MESSAGE);
}
else
{
strncpy(str, (char*)(buffer + qotd[index].pos), qotd[index].len);
str[qotd[index].len] = '\0';
printf("%s ", str);
}
}
int load(char *file)
{
FILE *stream;
char *line = NULL;
size_t length = 0;
ssize_t lineSize;
stream = fopen(file, "r");
if (stream == NULL)
{
return ERROR_CONDITION;
}
while ((lineSize = getline(&line, &length, stream)) != -1)
{
char* pos;
buffersize_t bufferSize;
if ((pos = strchr(line, ' ')) != NULL)
{
*pos = '\0';
}
bufferSize = getBufferSize(lineSize);
if (bufferSize == 0)
{
fclose(stream);
fprintf(stderr, "getBufferSize() %s", ERROR_MESSAGE);
return ERROR_CONDITION;
}
if ((buffer = realloc(buffer, bufferSize * sizeof(char))) == NULL)
{
fclose(stream);
fprintf(stderr, "realloc() %s", ERROR_MESSAGE);
return ERROR_CONDITION;
}
if (addQuote(strlen(buffer), lineSize - 1) == ERROR_CONDITION)
{
fclose(stream);
fprintf(stderr, "addQuote() %s", ERROR_MESSAGE);
return ERROR_CONDITION;
}
strncat(buffer, line, lineSize - 1);
}
fclose(stream);
return strlen(buffer);
}
int main (int argc, char** argv)
{
if (load(QOTD_FILE) == ERROR_CONDITION)
{
return ERROR_CONDITION;
}
quoteOfTheDay();
freePointer(buffer);
freePointer(qotd);
}
************************************************************************************************
qotd.h file
#ifndef __QOTD_H
#define __QOTD_H
#include
#include
#include
#include
#include
#include
#define QOTD_FILE "qotd.txt"
#define ERROR_MESSAGE "failed to allocate space for QOTD data. Exiting. "
#define SUCCESS 0
#define ERROR_CONDITION -1
typedef uint16_t buffersize_t;
typedef struct qotd_t
{
uint16_t pos;
uint16_t len;
} qotd_t;
#define BUFFERSIZE USHRT_MAX
#endif
***********************************************************************
qotd.txt
I did it ro I will do it. -- By myself It is better to light a candle than curse the darkness. -- Eleanor Roosevelt
Step by Step Solution
There are 3 Steps involved in it
Step: 1
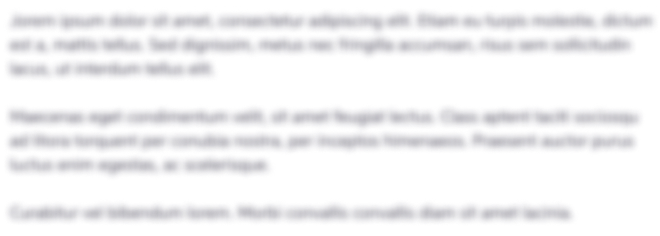
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started