Question
C PROGRAMMING: signals exercise Signals are a fairly blunt form of interprocess communication; like just a poke or a beep. But you can still encode
C PROGRAMMING: signals exercise
Signals are a fairly blunt form of interprocess communication; like just a poke or a beep. But you can still encode arbitrary data this way.
For this part of the lab you will work on two programs which communicate an arbitrary byte via signals. The "transmit" program will signal the eight bits of this byte, which is supplied on the command-line; and the "receive" program will receive these eight bits and output the single byte (plus a newline) to its standard output.
To begin, the receive program will output its process ID number (using getpid()), as part of an explanatory line of text. This makes it easier for you then to run the transmit program in another window: the transmit program takes the receive program's process ID number in argv[1], and the character to transmit in argv[2].
The transmit program will then transmit those eight bits one at a time. To transmit a zero, it signals the receive program with the signal "SIGUSR1" as defined in signal.h. To transmit a one, it signals the receive program with signal "SIGUSR2". (These two signal numbers are reserved for user-defined purposes.) To make sure that the signals arrive in order and are not combined, sleep for a tenth of a second inbetween each transmission using usleep(100000).
To assemble the eight bits in receive.c, you can start with a value of zero and keep left-shifting it by one bit, adding 1 when there's a 1 bit and not doing anything extra when there's a 0 bit (only the left-shift).
For example bit-manipulation code which disassembles the bits for use in transmit.c, see
#include
#include
#include
int main(int argc, char **argv)
{
int c, i;
if (argc != 2 || strlen(argv[1]) != 1) {
fprintf(stderr, "usage: %s character ", argv[0]);
return(1);
}
c = argv[1][0];
for (i = 0; i < 8; i++) {
if (c & 128)
printf("1 ");
else
printf("0 ");
c <<= 1;
}
return(0);
}
For an example program which waits for signals, see
/*
* This shows waiting for a signal, as you will have to do in receive.c.
* Actually, it waits for TWO signals -- you have to kill this program twice.
*/
#include
#include
#include
int signalcount = 0;
int main()
{
struct sigaction sa;
extern void myhandler();
sa.sa_handler = myhandler;
sigemptyset(&sa.sa_mask);
sa.sa_flags = 0;
sigaction(15, &sa, NULL);
printf("My pid is %ld ", (long)getpid());
while (signalcount < 2)
sleep(1234); /* will be interrupted by signal */
printf("Ack! ");
return(0);
}
void myhandler()
{
signalcount++;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
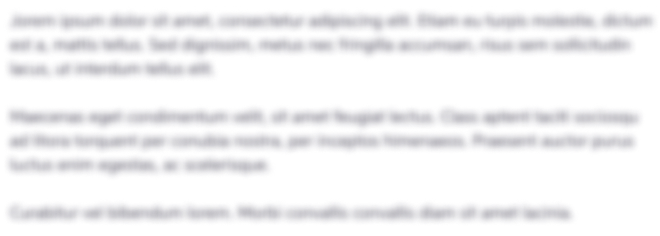
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started