Question
C Programming Take screenshot of compiled output once complete. (which should look similar to the sample one provided). Will rate positively. Problem: (1) Add PopTailSLL()
C Programming
Take screenshot of compiled output once complete. (which should look similar to the sample one provided). Will rate positively.
Problem:
(1) Add PopTailSLL() as a new member function to the SLL abstract data type. Unlink the logically-last node (tail) from the list *sll, call the DestructElement function to destruct the object pointed-to by the nodes element data member, free() the node, and decrement size. A SLL_UNDERFLOW exception occurs when size = 0.
void PopTailSLL(SLL *sll);
Hint PopTailSLL() is similar to PopHeadSLL(), but the PopTailSLL() algorithm must do a little more work than PopHeadSLL() to complete the unlinking that is required:
(2) Add IsInSLL() as a new member function to the SLL abstract data type. Return true when at least one node in *sll contains an element data member that points-to a character string that compares equal to the string pointed-to the parameter element; otherwise return false.
bool IsInSLL(const SLL *sll,const char *element);
(3) Add TraverseSLL() as a new member function to the SLL abstract data type.
void TraverseSLL(const SLL *sll,void (*ProcessElement)(char *element));
Note You will need to add the prototypes for the new member functions to SLL.h and add the new member function definitions to SLL.c.
(4)
Take screenshot of compiled output once complete. (which should look similar to the sample one provided). Will rate positively.
//--------------------------------------------------------------
// SLL ADT Problem #2
// Problem2.c
//--------------------------------------------------------------
#include
#include
#include
#include "..\Random.h"
#include ".\SLL.h"
//--------------------------------------------------------------
int main()
//--------------------------------------------------------------
{
void DestructElement(const char *element);
char *NewElement();
void DisplayElement(char *element);
int pushN;
SetRandomSeed();
printf("pushN? ");
while ( scanf("%d",&pushN) != EOF )
{
SLL sll;
int n,tries;
char *key = NewElement();
ConstructSLL(&sll,DestructElement);
for (n = 1; n
TraverseSLL(&sll,DisplayElement); printf(" ");
tries = 1;
while ( !IsInSLL(&sll,key) )
{
tries++;
free(key);
key = NewElement();
}
printf("It took %d tries to find \"%s\" ",tries,key);
free(key);
while ( !IsEmptySLL(&sll) )
{
printf("PopTailSLL()-ed \"%-7s\"\t",PeekSLL(&sll,GetSizeSLL(&sll)-1));
PopTailSLL(&sll);
if ( IsEmptySLL(&sll) )
printf("(empty) ");
else
TraverseSLL(&sll,DisplayElement), printf(" ");
}
DestructSLL(&sll);
printf(" pushN? ");
}
system("PAUSE");
return( 0 );
}
//--------------------------------------------------------------
char *NewElement()
//--------------------------------------------------------------
{
const char ALPHABET[] = "ABCDEFGHIJKLM"
"NOPQRSTUVWXYZ";
int i;
int len = RandomInteger(3,7);
char *element = (char *) malloc(sizeof(char)*(len+1));
for (i = 0; i
element[i] = RandomCharacter((char *) ALPHABET,26);
element[len] = '\0';
return( element );
}
//--------------------------------------------------------------
void DisplayElement(char *element)
//--------------------------------------------------------------
{
printf("%s ",element);
}
//--------------------------------------------------------------
void DestructElement(const char *element)
//--------------------------------------------------------------
{
free((char *) element);
}
//--------------------------------------------------------------
// Singly-linked list (SLL) abstract data type
// SLL.h
//--------------------------------------------------------------
#ifndef SLL_H
#define SLL_H
//==============================================================
// Data model definitions
//==============================================================
typedef struct SLLNODE
{
char *element;
struct SLLNODE *FLink; // (F)orward Link to logically-next node
} SLLNODE;
typedef struct SLL
{
int size;
SLLNODE *head; // pointer-to logically-first node
SLLNODE *tail; // pointer-to logically-last node
void (*DestructElement)(const char *element);
} SLL;
//==============================================================
// Public member function prototypes
//==============================================================
void ConstructSLL(SLL *sll,
void (*DestructElement)(const char *element));
void DestructSLL(SLL *sll);
void PushHeadSLL(SLL *sll,const char *element);
void PopHeadSLL(SLL *sll);
void PushTailSLL(SLL *sll,const char *element);
char *PeekSLL(const SLL *sll,const int offset);
bool IsEmptySLL(const SLL *sll);
int GetSizeSLL(const SLL *sll);
//==============================================================
// Private utility member function prototypes
//==============================================================
// (none)
#endif
//--------------------------------------------------------------
// Singly-linked list (SLL) abstract data type
// SLL.c
//--------------------------------------------------------------
#include
#include
#include
#include ".\SLL.h"
#include "..\ADTExceptions.h"
//--------------------------------------------------------------
void ConstructSLL(SLL *sll,
void (*DestructElement)(const char *element))
//--------------------------------------------------------------
{
sll->size = 0;
sll->head = NULL;
sll->tail = NULL;
sll->DestructElement = DestructElement;
}
//--------------------------------------------------------------
void DestructSLL(SLL *sll)
//--------------------------------------------------------------
{
while ( !IsEmptySLL(sll) )
{
PopHeadSLL(sll);
}
}
//--------------------------------------------------------------
void PushHeadSLL(SLL *sll,const char *element)
//--------------------------------------------------------------
{
SLLNODE *p = (SLLNODE *) malloc(sizeof(SLLNODE));
if ( p == NULL ) RaiseADTException(MALLOC_ERROR);
p->element = (char *) element;
if ( IsEmptySLL(sll) )
{
p->FLink = NULL;
sll->head = p;
sll->tail = p;
}
else
{
p->FLink = sll->head;
sll->head = p;
}
sll->size++;
}
//--------------------------------------------------------------
void PopHeadSLL(SLL *sll)
//--------------------------------------------------------------
{
SLLNODE *p = sll->head;
if ( GetSizeSLL(sll) == 0 ) RaiseADTException(SLL_UNDERFLOW);
if ( GetSizeSLL(sll) == 1 )
{
sll->head = NULL;
sll->tail = NULL;
}
else
{
sll->head = p->FLink;
}
if ( sll->DestructElement != NULL ) sll->DestructElement(p->element);
free(p);
sll->size--;
}
//--------------------------------------------------------------
void PushTailSLL(SLL *sll,const char *element)
//--------------------------------------------------------------
{
SLLNODE *p = (SLLNODE *) malloc(sizeof(SLLNODE));
if ( p == NULL ) RaiseADTException(MALLOC_ERROR);
p->element = (char *) element;
p->FLink = NULL;
if ( IsEmptySLL(sll) )
{
sll->head = p;
sll->tail = p;
}
else
{
sll->tail->FLink = p;
sll->tail = p;
}
sll->size++;
}
//--------------------------------------------------------------
char *PeekSLL(const SLL *sll,const int offset)
//--------------------------------------------------------------
{
SLLNODE *p = sll->head;
int i;
if ( !((0
for (i = 0; i
p = p->FLink;
return( p->element );
}
//--------------------------------------------------------------
bool IsEmptySLL(const SLL *sll)
//--------------------------------------------------------------
{
return( sll->size == 0 );
}
//--------------------------------------------------------------
int GetSizeSLL(const SLL *sll)
//--------------------------------------------------------------
{
return( sll->size );
}
//--------------------------------------------------------------
// ADTExceptions.h
//--------------------------------------------------------------
#ifndef ADTEXCEPTIONS_H
#define ADTEXCEPTIONS_H
// ADT exception definitions
#define MALLOC_ERROR "malloc() error"
#define DATE_ERROR "DATE error"
#define STACK_CAPACITY_ERROR "STACK capacity error"
#define STACK_UNDERFLOW "STACK underflow"
#define STACK_OVERFLOW "STACK overflow"
#define STACK_OFFSET_ERROR "STACK offset error"
// ADT exception-handler prototype
void RaiseADTException(char exception[]);
#endif
//--------------------------------------------------------------
// ADTExceptions.c
//--------------------------------------------------------------
#include
#include
#include
#include ".\ADTExceptions.h"
//--------------------------------------------------------------
void RaiseADTException(char exception[])
//--------------------------------------------------------------
{
fprintf(stderr,"\a Exception \"%s\" ",exception);
system("PAUSE");
exit( 1 );
}
/--------------------------------------------------
// Random.h
//--------------------------------------------------
#ifndef RANDOM_H
#define RANDOM_H
#include
// Initialize the "seed" used by the "rand()" function in
void SetRandomSeed(void);
// Return a uniformly and randomly chosen integer from [ LB,UB ].
int RandomInteger(int LB,int UB);
// Return a uniformly and randomly chosen real number chosen from [ 0.0,1.0 ).
double RandomReal(void);
// Return a uniformly and randomly chosen boolean from { false,true }.
bool RandomBoolean(void);
// Return a uniformly and randomly chosen character from characters[i] i in [ 0,(size-1) ].
char RandomCharacter(char characters[],int size);
#endif
//--------------------------------------------------
// Random.c
//--------------------------------------------------
#include
#include
#include
#include
#include ".\Random.h"
//--------------------------------------------------
void SetRandomSeed(void)
//--------------------------------------------------
{
srand(time(NULL));
}
//--------------------------------------------------
int RandomInteger(int LB,int UB)
//--------------------------------------------------
{
return( (int) (RandomReal()*(UB-LB+1)) + LB );
}
//--------------------------------------------------
double RandomReal()
//--------------------------------------------------
{
int R;
do
R = rand();
while ( R == RAND_MAX );
return( (double) R/RAND_MAX );
}
//--------------------------------------------------
bool RandomBoolean(void)
//--------------------------------------------------
{
return( RandomInteger(1,10000)
}
//--------------------------------------------------
char RandomCharacter(char characters[],int size)
//--------------------------------------------------
{
return( characters[RandomInteger(0,(size-1))] );
}
Sample Program Dialo E:COURSEsyCS1311Code'ADTsSLLVProblem2.exe ushN? ? PJHHMP WNXY MERLCW DSM PHDMG MNFMAS QECRET It took 154341 tries to find "DSM" PopTailsLLO-ed "QECRET PopTai1SLL-ed "MNFMAS" PopTailS LL()-ed PHDMG PopTa?1SLL()-ed ''DSM PJHHMP WNXY MERLCW DSM PHDMG MNFMAS PJHHMP WNRY MERLCW DSM PHDMG PJHHMP WNXY MERLCW DSH PJHHMP WNXY MERLCW PopTa?1SLL()-ed PopTailSLL-ed ''WNXY "PJHHMP" PJHHMPStep by Step Solution
There are 3 Steps involved in it
Step: 1
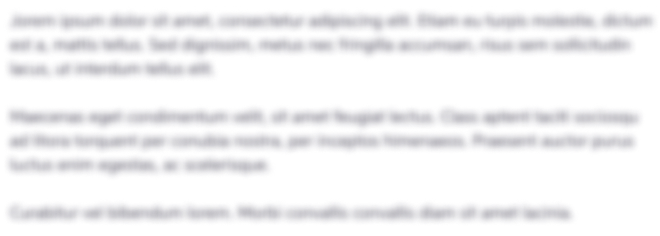
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started