Question
C programming Using the C programming language implement Heapsort in the manner described in class. Remember, you need only implement the sort algorithm, both the
C programming
Using the C programming language implement Heapsort in the manner described in class. Remember, you need only implement the sort algorithm, both the comparison and main functions have been provided.
here is the starter code
main.c:
/* * * main.c file * */
#include #include #include #include "srt.h"
static int compare(const void *, const void *);
int main(int argc, char *argv[]) {
int nelem = argc == 2 ? atoi(argv[1]) : SHRT_MAX;
TYPE *a = calloc(nelem, sizeof(TYPE));
#ifdef RAND for (int i = 0; i < nelem; ++i) {
a[i] = (TYPE)rand() / RAND_MAX; } #else for (int i = 0; i < nelem; ++i) {
a[i] = i; } #endif
#if defined BUBB srtbubb(a, nelem, sizeof(TYPE), compare); #elif defined HEAP srtheap(a, nelem, sizeof(TYPE), compare); #elif defined INSR srtinsr(a, nelem, sizeof(TYPE), compare); #elif defined MERG srtmerg(a, nelem, sizeof(TYPE), compare); #else qsort(a, nelem, sizeof(TYPE), compare); #endif
#ifdef PRNT for (int i = 0; i < nelem; ++i) {
printf("%f ", a[i]); } #else for (int i = 0; i < nelem - 1; ++i) {
if (a[i] > a[i + 1]) {
printf("fail "); goto end; } }
printf("pass "); #endif
end:
free(a);
return 0; }
static int compare(const void *p1, const void *p2) {
if (*(TYPE *)p1 < *(TYPE *)p2) {
return -5; } else if (*(TYPE *)p1 > *(TYPE *)p2) {
return +5; }
return 0; }
here is the srt.h code:
/* * * srt.h file * */
#ifndef SRT_H #define SRT_H
#include
#define MAX_BUF 256
#define swap(qx,qy,sz) \ do { \ char buf[MAX_BUF]; \ char *q1 = qx; \ char *q2 = qy; \ for (size_t m, ms = sz; ms > 0; ms -= m, q1 += m, q2 += m) { \ m = ms < sizeof(buf) ? ms : sizeof(buf); \ memcpy(buf, q1, m); \ memcpy(q1, q2, m); \ memcpy(q2, buf, m); \ } \ } while (0)
void srtbubb(void *, size_t, size_t, int (*)(const void *, const void *)); void srtheap(void *, size_t, size_t, int (*)(const void *, const void *)); void srtinsr(void *, size_t, size_t, int (*)(const void *, const void *)); void srtmerg(void *, size_t, size_t, int (*)(const void *, const void *));
#endif /* SRT_H */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
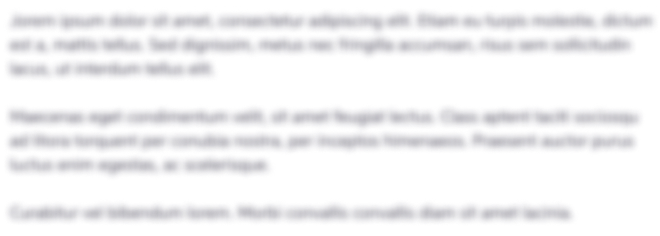
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started