Question
C Programming Write a program that reads from stdin, filters matching lines from the input, and writes the distinct lines to the standard output. More
C Programming
Write a program that reads from stdin, filters matching lines from the input, and writes the distinct lines to the standard output. More precisely, when multiple lines in the input match, only the first of these lines will be printed to the output. This program also handles command-line arguments that specify rules that determine whether two lines match. (What the program should accomplish)
For example, we have the following text file fruits.txt:
navel orange yellow banana red apple yellow banana red apple green apple navel
green apple green mango navel orange strawberry red apple Red apple apple
orange
We then run the following command:
./unique < fruits.txt
Without specifying any command-line options, we expect the program to print the following to stdout:
navel orange yellow banana red apple red apple green apple navel orange
green mango strawberry Red apple apple
Either sub-question of this question will specify the exact behavior of this program precisely. Thus the implementation and general requirements will be given before the sub-questions which will give the syntax of running the program.
Implementation Requirements: Read the input and store its distinct lines (the command-line arguments may be given to specify how to determine whether one line is distinct) in a linked list. Each distinct line is stored in one node of this linked list as a string variable. Each line of the input is expected to have at most 80 characters, excluding the terminating newline character. When one line of the input is too long, print an error message and terminate, without printing any line to stdout. This means that the string from each line can be stored in a character array of length 81.
You are expected to perform the task specified by each sub-question using this linked list.
General Requirements(Must Use And Apply All Files): Divide your source code into the following five files:
- unique.c: the file that contains the main function;
- lines.c and lines.h: the code for reading the lines from stdin and writing the lines to stdout (filtering matching lines can be done when you read the lines);
- match.c and match.h: the code for functions that determine whether two lines match, given options entered in the command-line.
In each module, divide your code into functions appropriately. In the header file, place prototypes of only those functions that are shared by difference modules. Prototypes of helper functions that are used in one module only should be placed in the corresponding .c file.
Write a makefile that will allow us to use the following command on bluenose to compile your program to generate an executable file named unique:
make unique
(i) First, implement this unique program without considering command-line arguments. In this case, two lines match each other if and only if they contain identical strings.
To run the program from a UNIX terminal using input redirection, we will enter the command
./unique < input_file
In this command line, input_file is the name of a plain text file. Each line is terminated by a trailing newline character, including the last line. You can assume that there are no empty lines in the input.
Error Handling: Your program should print an appropriate error message and terminate (without printing any lines from the input file) if there is at least one line that has more than 80 characters.
Testing: use the same input for testing
Test Case
./unique < fruits.txt > a.1
(a.1 Contents)
navel orange
yellow banana
red apple
red apple
green apple
navel orange
green mango
strawberry
Red apple
apple
Construct additional input files to fully test your program, as we will use different cases when testing your program.
Hints: You can modify the read_line function given in class for your program, so that when the end of file is reached, an empty string will be read. Recall that the getchar function returns a macro EOF when end of file is reached. Thus the following logical expression might be helpful:
(ch = getchar()) != && ch != EOF
(ii) Next implement one more feature for this program by adding an option that requires the program to skip the first f fields of any line when determining whether two lines match. If a certain line has fewer than f fields, treat this line as an empty string, i.e. a string that only stores the null character, when matching it against other strings.
For this we assume that each line of this file contains one or more fields separated by one or more space characters. To simplify your work, you can assume that other than the space characters used to separate the fields (there are no space characters after the last field of each line), and the trailing newline character, there are no other while-space characters.
Thus, to run the program from a UNIX terminal using input redirection, we will enter the command
./unique f < input_file In this command, f is a single digit between (and including) 0 and 9. Using the previous example, if we run
./unique 1 < fruits.txt
The output will be:
navel orange yellow banana red apple green mango strawberry
Note that the last line in the input file, i.e. apple, is not in the output. This is not because it matches any line that ends with apple, but because it matches strawberry! Find out why before starting the implementation.
Error Handling: In addition to the error case that you are supposed to handle in (i), your program should also print an appropriate error message and terminate (without printing any lines from the input file) if:
The user supplies illegal command-line arguments;
The number of command-line arguments supplied is incorrect (important: make sure that your program will still run without any command-line argu- ments as specified in (i)).
If there is more than one problem with user input, your program just has to detect one of them. You can assume that everything else regarding the input file is correct.
Testing: test for the three different cases.
Test Cases
./unique 0 < fruits.txt > b.0
(Contents in b.0)
navel orange
yellow banana
red apple
red apple
green apple
navel orange
green mango
strawberry
Red apple
apple
./unique 1 < fruits.txt > b.1
(Contents in b.1)
navel orange
yellow banana
red apple
green mango
strawberry
./unique 2 < fruits.txt > b.2
(Contents in b.2)
navel orange
Step by Step Solution
There are 3 Steps involved in it
Step: 1
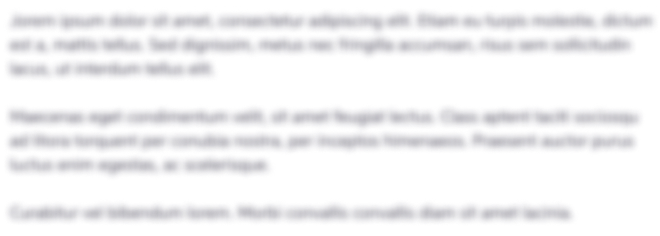
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started