Question
C++ Programming.....Please help me fix this Write your own version of a class template that will create a dynamic stack of any data type. The
C++ Programming.....Please help me fix this
Write your own version of a class template that will create a dynamic stack of any data type. The pop function must return a bool; it should return a false if it was not able to pop an item off the stack. Otherwise it returns true. The parameter to the pop function is passed by reference and should be the item on the list if it was able to pop something. Create a driver program (main) that shows that the stack works for at least two different data types.
I have done this so far. But it gives me error and does not compile.
////"DynStack.h"
#pragma once
/* This file contains the decleration of DynStack class */
// Declaration file for the DynStack class. This class is a template version of
// a dynamic stack class.
#ifndef DYNSTACK_H
#define DYNSTACK_H
template
class DynStack
{
private:
// Struct for stack nodes
struct StackNode {
T value; // holds the node's value
StackNode *next; // ptr to next node
};
StackNode *top; // ptr to stack top
public:
// Constructor
DynStack();
// Destructor
~DynStack();
// Stack operations
void push(T);
bool pop(T &);
bool isEmpty();
};
#endif
//////"DynStack.cpp"
// This file contains Implementation for the DynStack class. This class is a template version of
// a dynamic stack class.
#include "DynStack.h"
// Default constructor
template
DynStack::DynStack()
{
top = nullptr;
}
// Destructor - deletes the stack node by node
template
DynStack::~DynStack()
{
StackNode *nodePtr;
StackNode *nextNode;
// aim nodePtr at top of stack
nodePtr = top;
// travel list and delete each node
while (nodePtr != nullptr)
{
nextNode = nodePtr->next;
delete nodePtr;
nodePtr = nextNode;
}
}
///////////////////////
// Stack operations //
/////////////////////
// push() adds the argument onto the stack
template
void DynStack::push(T item)
{
StackNode *newNode ;
// Creates a new node and stores argument there
newNode = new StackNode;
newNode->value = item;
// If the list is empty, make newNode the first node
if (isEmpty() == true)
{
top = newNode;
newNode->next = nullptr;
}
else
{
newNode->next = top;
top = newNode;
}
}
// If the stack is empty, then pop() simply returns false.
// If a node exists, pop() returns the top item, deletes it from the stack,
// and then returns true.
template
bool DynStack::pop(T &item)
{
StackNode *temp ;
bool status;
// Check that the stack isn't empty
if (isEmpty() == true)
{
status = false;
}
else // Pop value off top of stack
{
item = top->value;
temp = top->next;
delete top;
top = temp;
status = true;
}
return status;
}
// isEmpty() returns true if stack is empty; otherwise it returns false.
template
bool DynStack::isEmpty()
{
bool status;
if (!top)
status = true;
else
status = false;
return status;
}
/////"Main.cpp"
#include
#include "DynStack.h"
using namespace std;
int main()
{
int var;
char ch;
DynStack stack;
cout << "Pushing 2" << endl;
stack.push(2);
cout << "Pushing 2" << endl;
stack.push(3);
cout << "Pushing 2" << endl;
stack.push(4);
cout << "Popping...." << endl;
stack.pop(var);
cout << var << endl;
stack.pop(var);
cout << var << endl;
stack.pop(var);
cout << var << endl;
//stack for characters
DynStack charstack;
cout << "Pusshing a" << endl;
charstack.push('a');
cout << "Pusshing b" << endl;
charstack.push('b');
cout << "Pusshing c" << endl;
charstack.push('c');
cout << "Popping..." << endl;
charstack.pop(ch);
cout << ch << endl;
charstack.pop(ch);
cout << ch << endl;
charstack.pop(ch);
cout << ch << endl;
system("pause");
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
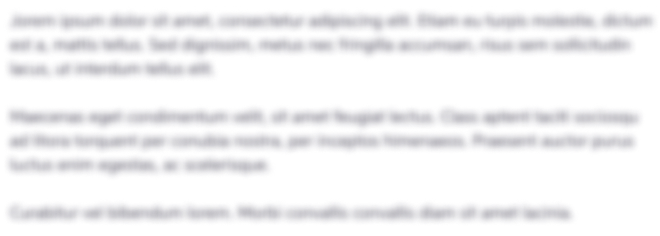
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started