Question
C++ Project Create a templated queue class with an array based variant. An example header file below is given holding elements of type class DataType.
C++ Project
Create a templated queue class with an array based variant.
An example header file below is given holding elements of type class DataType. This class should be templated
const int ARRAY_MAX = 1000;
class ArrayQueue{
public:
ArrayQueue(); //Instantiate a new Queue object with no valid data
ArrayQueue(int size, const DataType& value); // Instantiate a new Queue object which will hold int size number of elements in total, all of them initialized to be equal to parameter //value
ArrayQueue(const ArrayQueue& other); //Instantiate a new Queue object which will be a seperate copy of the data of the other Queue object which is getting copied
~ArrayQueue(); // Destroys the instance of the Queue object
ArrayQueue& operator=(const ArrayQueue& other_arrayQueue); // Will assign a new value to the calling Queue object, which will be an exact copy of the other_arrayQueue object //passed as a parameter. Returns a reference to the calling object to be used for cascading operator=
DataType& front(); //returns a reference to the front element of the queue (before calling this method ensure the queue isnt empty)
const DataType& front() const;
DataType& back(); //returns a reference to the back element of the queue (before calling this method ensure the queue isnt empty)
const DataType& back() const;
void push(const DataType& value); // Inserts at the back of the Queue an element of the given value
void pop(); //removes from the front element of the queue
int size() const; // returns the size of the current queue
bool empty() const; // will return true if the queue is empty
bool full() const; // will return true if the queue is full
void clear(); // after called the queue will be considered empty
friend std::ostream& operator<<(std::ostream& os, const ArrayQueue& arrayQueue); //will output the complete content of the calling queue object. (Not required to be templated)
private:
DataType m_array[ARRAY_MAX]; //The array that holds the data. Both parameters determined via template parameters
int m_front; // an int with the respective m_array index of the front element of the queue
int m_back; // an int with the respective m_array index of the last element of the queue
int m_size; // keeps track of how many elements are in the queue (should not exceed ARRAY_MAX)
};
/*The implementation will be a wrap around queue, meaning that,
a) pushing an element will move the back by one (m_back=(m_back+1)%ARRAY_MAXSIZE) //and increase the size by one
b) popping an element will move the front by one (m_front=(m_front+1)%ARRAY_MAXSIZE) // and decrease size by one
*/
Step by Step Solution
There are 3 Steps involved in it
Step: 1
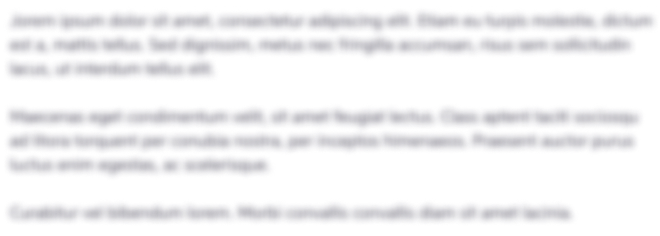
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started