Question
C++ Project with tons of information, seems long but actual project should not be bad jsut could not figure it out. Summary: This assignment is
C++ Project with tons of information, seems long but actual project should not be bad jsut could not figure it out.
Summary: This assignment is a greatly reduced version of what was originally discussed in class, in order to give you a reasonable task to complete in the time we have remaining. Rather than produce perspective images from a combination of image data and elevation data, well extract a portion of the surface of Mars and produce an image with topographical information overlaid on it. The assignment is to write a program which can draw a top-down view of a portion of the surface of Mars, and using a digital elevation map of the same portion of Mars, overlay elevation data on it. A PGM image covering the entire surface of Mars, and an elevation map of Mars are provided below in links to files that can be downloaded to accomplish this task. A complete solution will scale the image properly to correct for latitude stretching in the original PGM, and draw contour lines on resulting image using elevation data from the EGM file. Alternately, for a lower grade, the solution can simply identify the highest elevation and lowest elevation points in the image by drawing a small circle over those points. Similarly, for a lower grade, the program can skip the longitude correction, in which case the resulting image will be distorted horizontally, so that craters which are circular on the surface will appear wider horizontally because of the 1/cos(latitude) stretching that occurs in the original files.
Details: Your program should prompt the user for a rectangular latitude and longitude region to extract from the PGM and EGM files (i.e., prompt for upper left and lower right lat & lon coordinates). It should read and extract that portion of the two files and produce a PGM image from the combined data. A complete solution will overlay contour lines from the elevation data using information from the EGM elevation file, but a simpler solution can simply find the maximum and minimum elevation points and draw a circle around them on the output image. The resulting image should be written to a PGM file. The PGM and EGM files are rectangular, with constant pixels-per-degree scaling in both X (longitude) and Y (latitude) directions. Since Mars is actually a sphere, then latitudes farther from the equator are stretched in the X direction, so a proper solution will scale the pixels in the X direction by cos(latitude). A rigorous solution would require slightly different scaling for each row of the image, but it is sufficient to scale the entire image using the latitude of the midpoint of the image. The PGM image is the equivalent of a top-down satellite view of the surface. You are not required to produce a perspective image from thisjust extract the top-down view, scale it correctly, and write out the sub-image to a PGM output file.
Dynamic Memory Allocation A proper solution will use dynamic memory allocation to create the arrays needed to read in the PGM and EGM images, and for the output image as well. It should return all memory to the system before exiting. If you cant do that, then use fixed size global arrays or static arrays for a lesser grade. Binary Input and Output The Mars-wide PGM and EGM files are binary. Youll need to use C++s binary read and write functions for a full solution of this assignment. Ascii versions of a subset of the surface of Mars will also be provided, but will take much longer to read. Your output image should be a binary P5 grayscale (or optionally P6 color) image, but you may write it out as an ascii P2 or P3 image for a lesser grade if necessary. The binary PGM file format is documented on the NetPBM website, http://netpbm.sourceforge.net/doc/pgm.html . In summary, its the header information (P2 for ascii and P5 for binary), along with the number of columns, rows and maxval, followed by a single whitespace. (Note: there are no header comments, so you dont need to worry about handling comments when reading the header). Following that header is the rectangular image array, size ncols by nrows. If you open the file in binary mode, you can still read the header in with >> , then after reading the maxval, read a single character in binary mode (the white space following the maxval), then read the image array using ifstreams read() function. Youll probably want the pixels in an integer array, but the data is 8-bit unsigned char, so read it initially into an unsigned char array, then copy it into your integer array using a for loop. The binary EGM format consists of a 32-byte header, followed by a rectangular array of The binary EGM image files header is binary, rather than ascii (unlike binary PGM, and consists of the following. It is described in Lab 08s Extra Credit section. It consists of an 32-byte header (8 chars and 6 integers), followed by the pixel array stored as 2-byte short integers. The header tells you the image size (ncols & nrows), the minimum and maximum elevations in the data, and the x- and y-scaling. The scaling is importantyour elevation data might be in meters, but there might be 100 meters between pixels in the x and y directions. Then if you ignore the scaling, your mountains would be 100 times too tall! (We dont need the x and y scaling for this project, as long as we have the overall pixel scaling in pixels per degree, which is given in the filename.) Basically, you can read it the header with something like this. 1. Read the 32 bytes into an integer array (called, e.g., hdr_info) 2. Check that the 1st two bytes are E and 4 3. Use hdr_info[2] and [3] for #cols and #rows 4. Use hdr_info[4] and [5] for the minimum and maximum elevations 5. User hdr_info[6] and [7] for the x- and y-scaling. int hdr_info[8]; char *typ = hdr_info; // point a char * at beginning so we can check for E4 ifs.read(reinterpret_cast ifs.read(reinterpret_cast(p),nc*nr*sizeof(short int)); Extracting a rectangular region: The image and elevation filenames tell you the pixel scaling. Mola_16_globe.egm and mola_16_globe_illum.pgm are 16-pixels-per-degree in both x and y. The top row is + 90 degrees latitude, the middle row is the equator, and the bottom row is -90 degrees latitude. The first column is 0 degrees longitude, and the last column is 359.9375 degrees. Scaling by Latitude: The Milankovic crater is pretty far north and southern crater far south), and because the image and elevation files are rectangular grids of a spherical surface, the points nearer the poles are stretched out in the x-direction relative to the y direction. The stretching is by 1/cos(theta). The scaling actually changes from top to bottom, but well assume a fixed scaling based on the middle latitude of the region. So, to draw it properly, you want an output image that is narrower than it is tall. Milankovic should be cos(54.7) * pgm width, by full pgm high. So for a 160x160 rectangle around Milankovic Crater, rewrite the extracted pixels into a 92 x 160 image. You can simply subsample to get the resulting image (take Dr. Sarrafs course on image processing for algorithms for resampling images efficiently). Creating contour lines: This is really quite simple, once you have the elevation data in a matrix. Assume that you have elevations ranging from -200 meters to +1800 meters (2000 meters total), and you want a contour line every 250 meters (8 contours over the entire range). Subtract the minimum elevation from all pixels ( i.e., add 200), so you have elevations between 0 and 2000. Then, using integer arithmetic, if you divide all elevations by 25, then youll have integer values between 8 and 8. Now, if you go through the array pixel by pixel, any pixel whose value is greater than any of its neighbors is a on a contour line. So, create a second array the same size, set it all to zeros, and set any contour pixel to, say, 64. If you write this array out to a PGM by itself, youll have dark gray contours on a black background. Vaguely interesting. But if you add this pixel array, point by point, to the PGM of the Mars surface at that location, then youll end up with light lines drawn over top of the original image. For any really bright pixel (above 192), just wrap the value around (modulus 256!) and youll have a dark line over the bright pixels. Terraforming Mars Mars is currently a dusty red, but if Elon Musk has his way, well start terraforming it once we get humans living there. (Captain Kirk and the Enterprise managed to do it in a matter of minutes to a planet on Star Trek, and thereby revived Mr. Spock who had died) So for a little extra credit, instead of writing out a P2 or P5 gray scale PGM image, you could colorize it and write out a P3 or P6 PPM color image. Colormap_mars.txt and Colormap_mountains.txt are two different 256-row by 3-column RGB colormaps, with green for low values (valleys), yellow (quaking aspen trees) for middle elevations, and brown for the high elevations. You could write out PPMs (typ=P6) with three values (red, green and blue) for each pixel, based on the elevation, and have a terraformed Mars. This would be extra credit.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
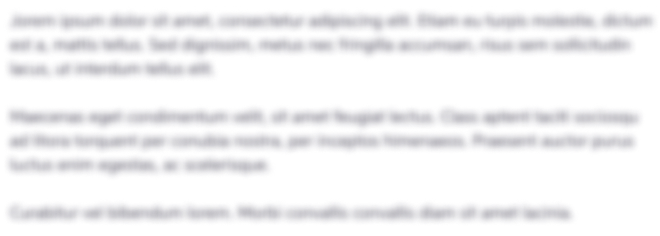
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started