C++ Q2. Write a class CircularLinkedList that will able to run with the following main function. void main() { CircularLinkedList myList; myList.InsertAtFront(10); myList.InsertAtFront(20); myList.InsertAtFront(30); myList.InsertAtFront(40);
C++
Q2. Write a class CircularLinkedList that will
able to run with the following main function.
void main()
{
CircularLinkedList myList;
myList.InsertAtFront(10);
myList.InsertAtFront(20);
myList.InsertAtFront(30);
myList.InsertAtFront(40);
myList.InsertAtEnd(60);
myList.InsertAtEnd(70);
myList.InsertAtEnd(80);
myList.InsertAfter(60,65);
myList.InsertBefore(30,35);
myList.Print(); /*print all the elements
in the circular linked list. It must be:
40 35 30 20 10 60 65 70 80 */
myList.Delete(30);
myList.Delete(300);
myList.DeleteAfter(20);
myList.DeleteBefore(20);
myList.Delete(40);
myList.DeleteAfter(80);
myList.DeleteBefore(60);
myList.Print(); /*print all the elements
in the circular linked list. It must be:
60 65 70 */
myList.DeleteAll();
myList.Print(); /*print all the elements
in the circular linked list. It must be:
*/
myList.SortedInsert(10);
myList.SortedInsert(20);
myList.SortedInsert(30);
myList.SortedInsert(40);
myList.SortedInsert(60);
myList.SortedInsert(70);
myList.SortedInsert(80);
myList.Print(); /*print all the elements
in the circular linked list. It must be:
10 20 30 40 60 70 80 */
myList.InsertAtFront(80);
myList.Print(); /*print all the elements
in the circular linked list. It must be:
80 10 20 30 40 60 70 80 */
myList.DeleteDuplicate(80);
myList.Print(); /*print all the elements
in the circular linked list. It must be:
10 20 30 40 60 70 */
myList.DeleteFirst();
myList.DeleteLast();
myList.Print(); /*print all the elements
in the circular linked list. It must be:
20 30 40 60 */
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
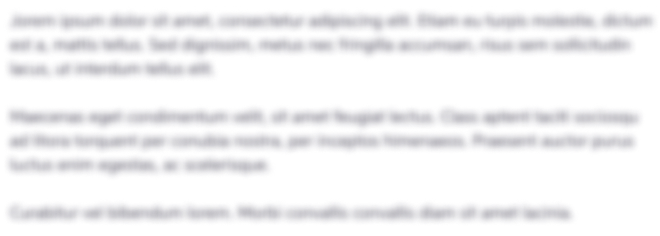
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started