Question
C++ Rewrite Lab 10 using the doublyLinkedList instead of an array (Note: You dont have to limit the size to 100) #ifndef H_doublyLinkedList #define H_doublyLinkedList
C++ Rewrite Lab 10 using the doublyLinkedList instead of an array (Note: You dont have to limit the size to 100)
#ifndef H_doublyLinkedList
#define H_doublyLinkedList
#include
#include
using namespace std;
//Definition of the node
template
struct nodeType
{
Type info;
nodeType
nodeType
};
template
class doublyLinkedList
{
public:
const doublyLinkedList
(const doublyLinkedList
//Overload the assignment operator.
void initializeList();
//Function to initialize the list to an empty state.
//Postcondition: first = nullptr; last = nullptr; count = 0;
bool isEmptyList() const;
//Function to determine whether the list is empty.
//Postcondition: Returns true if the list is empty,
// otherwise returns false.
void destroy();
//Function to delete all the nodes from the list.
//Postcondition: first = nullptr; last = nullptr; count = 0;
void print() const;
//Function to output the info contained in each node.
void reversePrint() const;
//Function to output the info contained in each node
//in reverse order.
int length() const;
//Function to return the number of nodes in the list.
//Postcondition: The value of count is returned.
Type front() const;
//Function to return the first element of the list.
//Precondition: The list must exist and must not be empty.
//Postcondition: If the list is empty, the program
// terminates; otherwise, the first
// element of the list is returned.
Type back() const;
//Function to return the last element of the list.
//Precondition: The list must exist and must not be empty.
//Postcondition: If the list is empty, the program
// terminates; otherwise, the last
// element of the list is returned.
bool search(const Type& searchItem) const;
//Function to determine whether searchItem is in the list.
//Postcondition: Returns true if searchItem is found in
// the list, otherwise returns false.
void insert(const Type& insertItem);
//Function to insert insertItem in the list.
//Precondition: If the list is nonempty, it must be in
// order.
//Postcondition: insertItem is inserted at the proper place
// in the list, first points to the first
// node, last points to the last node of the
// new list, and count is incremented by 1.
void deleteNode(const Type& deleteItem);
//Function to delete deleteItem from the list.
//Postcondition: If found, the node containing deleteItem
// is deleted from the list; first points
// to the first node of the new list, last
// points to the last node of the new list,
// and count is decremented by 1; otherwise,
// an appropriate message is printed.
doublyLinkedList();
//default constructor
//Initializes the list to an empty state.
//Postcondition: first = nullptr; last = nullptr; count = 0;
doublyLinkedList(const doublyLinkedList
//copy constructor
~doublyLinkedList();
//destructor
//Postcondition: The list object is destroyed.
protected:
int count;
nodeType
nodeType
private:
void copyList(const doublyLinkedList
//Function to make a copy of otherList.
//Postcondition: A copy of otherList is created and
// assigned to this list.
};
template
doublyLinkedList
{
first= nullptr;
last = nullptr;
count = 0;
}
template
bool doublyLinkedList
{
return (first == nullptr);
}
template
void doublyLinkedList
{
nodeType
while (first != nullptr)
{
temp = first;
first = first->next;
delete temp;
}
last = nullptr;
count = 0;
}
template
void doublyLinkedList
{
destroy();
}
template
int doublyLinkedList
{
return count;
}
template
void doublyLinkedList
{
nodeType
current = first; //set current to point to the first node
while (current != nullptr)
{
cout << current->info << " "; //output info
current = current->next;
}//end while
}//end print
template
void doublyLinkedList
{
nodeType
//the list
current = last; //set current to point to the
//last node
while (current != nullptr)
{
cout << current->info << " ";
current = current->back;
}//end while
}//end reversePrint
template
bool doublyLinkedList
search(const Type& searchItem) const
{
bool found = false;
nodeType
current = first;
while (current != nullptr && !found)
if (current->info >= searchItem)
found = true;
else
current = current->next;
if (found)
found = (current->info == searchItem); //test for
//equality
return found;
}//end search
template
Type doublyLinkedList
{
assert(first != nullptr);
return first->info;
}
template
Type doublyLinkedList
{
assert(last != nullptr);
return last->info;
}
template
void doublyLinkedList
{
nodeType
nodeType
nodeType
bool found;
newNode = new nodeType
newNode->info = insertItem; //store the new item in the node
newNode->next = nullptr;
newNode->back = nullptr;
if(first == nullptr) //if the list is empty, newNode is
//the only node
{
first = newNode;
last = newNode;
count++;
}
else
{
found = false;
current = first;
while (current != nullptr && !found) //search the list
if (current->info >= insertItem)
found = true;
else
{
trailCurrent = current;
current = current->next;
}
if (current == first) //insert newNode before first
{
first->back = newNode;
newNode->next = first;
first = newNode;
count++;
}
else
{
//insert newNode between trailCurrent and current
if (current != nullptr)
{
trailCurrent->next = newNode;
newNode->back = trailCurrent;
newNode->next = current;
current->back = newNode;
}
else
{
trailCurrent->next = newNode;
newNode->back = trailCurrent;
last = newNode;
}
count++;
}//end else
}//end else
}//end insert
template
void doublyLinkedList
{
nodeType
nodeType
bool found;
if (first == nullptr)
cout << "Cannot delete from an empty list." << endl;
else if (first->info == deleteItem) //node to be deleted is
//the first node
{
current = first;
first = first->next;
if (first != nullptr)
first->back = nullptr;
else
last = nullptr;
count--;
delete current;
}
else
{
found = false;
current = first;
while (current != nullptr && !found) //search the list
if (current->info >= deleteItem)
found = true;
else
current = current->next;
if (current == nullptr)
cout << "The item to be deleted is not in "
<< "the list." << endl;
else if (current->info == deleteItem) //check for
//equality
{
trailCurrent = current->back;
trailCurrent->next = current->next;
if (current->next != nullptr)
current->next->back = trailCurrent;
if (current == last)
last = trailCurrent;
count--;
delete current;
}
else
cout << "The item to be deleted is not in list."
<< endl;
}//end else
}//end deleteNode
template
void doublyLinkedList
{
//ENTER CODE HERE (20%)
}//end copyList
template
doublyLinkedList
{
//ENTER CODE HERE (20%)
}
template
const doublyLinkedList
(const doublyLinkedList
{
//ENTER CODE HERE (20%)
}
template
doublyLinkedList
{
cout << "Definition of the destructor is left as an exercise." << endl;
cout << "See Programming Execrise 9." << endl;
}
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
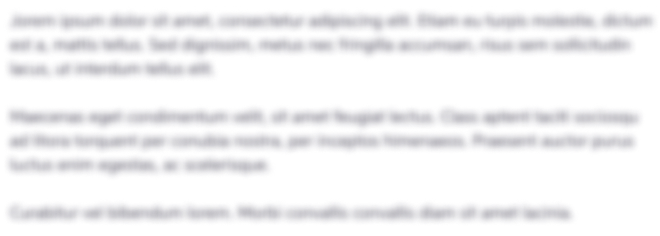
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started