Question
C++, simple programming, produce a two classes that work together to simulate a MegaMillions lottery. In an effort to help you, the design of these
C++, simple programming, produce a two classes that work together to simulate a MegaMillions lottery. In an effort to help you, the design of these two classes will be discussed here. In addition, some sample code has been provided to assist you with this task. Various UML diagrams are shown below to communicate the code you need to create. Please follow the steps outlined below and don't jump ahead until you have finished the earlier step.
First, you will create the class MegaMillionsTicket. This class represents a player's ticket and stores the values of 5 balls plus the megaball. As described in the rules of the game, balls 1-5 should each come a value between 1 to 75 and the megaball should come from a value between 1 to 25. Balls 1-5 must each be different but the megaball is allowed to match the value of the first five balls, if that should occur. Please review the class diagram shown here:
As designed, the class MegaMillionsTicket stores 6 different ints and provides accessor methods for each one. Create a .h and .cpp for this class. Write some sample driver code and assertions to verify that your accessor methods are all working properly.
Next, acquire the RandomNumber class. This is code I am providing to you to complete this programming project. Click on the links for the .h and .cpp file for the RandomNumber class. Add it to your solution or project. This class provides you with a way to acquire a random int value within a specified range. Please review the class diagram shown here:
As described in the rules of the game, each ticket may potentially win a prize. You need to create an enumeration called WinningPossibility that will list the different possible winning outcomes. This enumeration will need to be a public part of the class MegaMillionsLottery which I am about to explain next. Please review the enumeration shown here:
The second class you will create is called MegaMillionsLottery. This class represents the winning combination for one particular MegaMillions game. Similar to a MegaMillionsTicket, the MegaMillionsLottery stores the values of 5 balls plus the megaball. Please review the class diagram shown here:
When the constructor is called with no arguments, use the RandomNumber class to generate all the ball values. When the constructor is called with 6 ints, use those values for the balls. The WinningPossibility enumeration needs to be a public part of the MegaMillionsLottery class. Create a .h and .cpp for this class. Write some sample driver code and assertions to verify that your accessor methods are all working properly.
Once you get to this point, there are three remaining class methods to implement. Each are described below.
1. MegaMillionsTicket MegaMillionsLottery::quickPick( );
This method should return a MegaMillionsTicket correctly filled with random ball values for the driver code to inspect as diagrammed below.
2. MegaMillionsLottery::WinningPossibility MegaMillionsLottery::checkTicket( MegaMillionsTicket ticket );
This method should check the values found in the ticket parameter against the lottery's values and return the WinningPossibility represented by this ticket. (Because of how hard it is to win, much of the time, your code will report NOTWINNING, unless you cheat..)
3. string MegaMillionsLottery::printWwhatHappened( MegaMillionsTicket ticket );
This method should check the values found in the ticket parameter against the lottery's values and return a string that describes what happened so you can textually read whether you won or not. Yes, I am expecting this method to call the checkTicket method you created in Step 2. You should print one of the following messages, depending on the ticket passed:
You didn't win anything at all!
You matched the megaball!
You matched 1 ball plus the megaball!
You matched 2 balls plus the megaball!
You matched 3 balls plus the megaball!
You matched 3 balls!
You matched 4 balls plus the megaball!
You matched 4 balls!
You matched 5 balls!
You matched 5 balls plus the megaball!
You are free to create additional public and private methods and data members as you see fit. However, the test cases will only be driving the public methods of the two classes diagrammed here.
The source files you turn in will these classes and a main routine. You can have the main routine do whatever you want, because we will rename it to something harmless, never call it, and append our own main routine to your file. Our main routine will thoroughly test your functions. You'll probably want your main routine to do the same. If you wish, you may write functions in addition to those required here. We will not directly call any such additional functions. If you wish, your implementation of a function required here may call other functions required here.
The program you turn in must build successfully, and during execution, no method may read anything from cin. If you want to print things out for debugging purposes, write to cerr instead of cout. When we test your program, we will cause everything written to cerr to be discarded instead we will never see that output, so you may leave those debugging output statements in your program if you wish.
Your program must not use any function templates from the algorithms portion of the Standard C++ library. If you don't know what the previous sentence is talking about, you have nothing to worry about. If you do know, and you violate this requirement, you will be required to take an oral examination to test your understanding of the concepts and architecture of the STL.
Your implementations must not use any global variables whose values may be changed during execution.
Your program must build successfully under both Visual C++ and either clang++ or g++.
The correctness of your program must not depend on undefined program behavior.
The text files named MegaMillionsTicket.h and MegaMillionsTicket.cpp that implement the MegaMillionsTicket class diagrammed above, the text files named MegaMillionsLottery.h and MegaMillionsLottery.cpp that implement the MegaMillionsLottery class diagrammed above, the text files named RandomNumber.h and RandomNumber.cpp that implement the RandomNumber class diagrammed above (yes, these are the ones you downloaded for free...) and the text file named main.cpp which will hold your main program. Your source code should have helpful comments that explain any non-obvious code.
Tests:
#include#include #include #include "MegaMillionsLottery.h" #include "MegaMillionsTicket.h" #include "RandomNumber.h" using namespace std; int main() { // sample test code // test code MegaMillionsTicket ticket( 1, 2, 3, 4, 5, 6 ); assert( ticket.getBall1() == 1); assert( ticket.getBall2() == 2); assert( ticket.getBall3() == 3); assert( ticket.getBall4() == 4); assert( ticket.getBall5() == 5); assert( ticket.getMegaBall() == 6); MegaMillionsLottery lottery( 1, 2, 3, 4, 5, 6 ); assert( lottery.getBall1() == 1); assert( lottery.getBall2() == 2); assert( lottery.getBall3() == 3); assert( lottery.getBall4() == 4); assert( lottery.getBall5() == 5); assert( lottery.getMegaBall() == 6); assert( lottery.checkTicket(ticket) == MegaMillionsLottery::WinningPossibility::FIVEPLUSMEGABALL); assert( lottery.whatHappened( ticket ) == "You matched 5 balls plus the megaball!" ); ticket = MegaMillionsTicket( 1, 2, 3, 4, 5, 12 ); assert( lottery.checkTicket(ticket) == MegaMillionsLottery::WinningPossibility::FIVE); assert( lottery.whatHappened( ticket ) == "You matched 5 balls!" ); ticket = MegaMillionsTicket( 1, 2, 3, 4, 15, 12 ); MegaMillionsTicket quickPickTicket( 1, 2, 3, 4, 5, 6); for (int i = 0; i Mega MillionsTicket -mBall1 : int -mBall2 : int -mBall3 : int -mBall4 : int -mBall5 : int -mMegaBall : int +Mega MillionsTicket(ball1 : int, ball2 : int, ball3 : int, ball4 : int, ball5 : int, megaball : int) +getBall1(): int +getBall2(): int +getBall3() : int +getBall4(): int +getBall50) : int +getMegaBall(: int RandomNumber -mMinimum : int -mMaximum : int +RandomNumber( min : int, max: int, minInclusive : bool = true, maxInclusive : bool = true) +random() : int WinningPossibility MEGABALL ONEPLUSMEGABALL TWOPLUSMEGABALL THREE THREEPLUSMEGABALL FOUR FOURPLUSMEGABALL FIVE FIVEPLUSMEGABALL NOTWINNING Mega MillionsLottery -mBall1 : int -mBall2 : int -mBall3: int -mBall4 : int -mBall5 : int -mMegaBall : int +Mega MillionsLottery(). +MegaMillionsLottery(ball1 : int, ball2: int, ball3 : int, ball4: int, ball5 : int, megaball : int ) +getBall10 : int +getBall2(): int +getBall3(): int +getBall4(: int +getBall50) : int +getMegaBall( ) : int +quickPick(): Mega MillionsTicket +checkTicket( ticket : Mega MillionsTicket ) : Winning Possibility +whatHappened( ticket: Mega MillionsTicket): string Mega MillionsLottery MegaMillionsLottery creates creates > Mega MillionsTicket MegaMillionsTicket Mega MillionsTicket -mBall1 : int -mBall2 : int -mBall3 : int -mBall4 : int -mBall5 : int -mMegaBall : int +Mega MillionsTicket(ball1 : int, ball2 : int, ball3 : int, ball4 : int, ball5 : int, megaball : int) +getBall1(): int +getBall2(): int +getBall3() : int +getBall4(): int +getBall50) : int +getMegaBall(: int RandomNumber -mMinimum : int -mMaximum : int +RandomNumber( min : int, max: int, minInclusive : bool = true, maxInclusive : bool = true) +random() : int WinningPossibility MEGABALL ONEPLUSMEGABALL TWOPLUSMEGABALL THREE THREEPLUSMEGABALL FOUR FOURPLUSMEGABALL FIVE FIVEPLUSMEGABALL NOTWINNING Mega MillionsLottery -mBall1 : int -mBall2 : int -mBall3: int -mBall4 : int -mBall5 : int -mMegaBall : int +Mega MillionsLottery(). +MegaMillionsLottery(ball1 : int, ball2: int, ball3 : int, ball4: int, ball5 : int, megaball : int ) +getBall10 : int +getBall2(): int +getBall3(): int +getBall4(: int +getBall50) : int +getMegaBall( ) : int +quickPick(): Mega MillionsTicket +checkTicket( ticket : Mega MillionsTicket ) : Winning Possibility +whatHappened( ticket: Mega MillionsTicket): string Mega MillionsLottery MegaMillionsLottery creates creates > Mega MillionsTicket MegaMillionsTicket
Step by Step Solution
There are 3 Steps involved in it
Step: 1
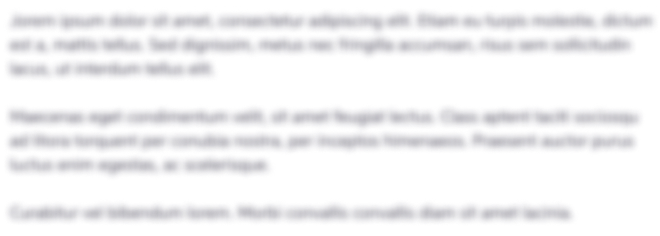
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started