Question
C++ use the .cpp, .hpp, and main.cpp as shown below. Program Tasks (What do I have to do exactly?): In this homework, you will extend
C++ use the .cpp, .hpp, and main.cpp as shown below.
Program Tasks (What do I have to do exactly?): In this homework, you will extend your book database in the following way: 1. Add a feature to delete a record from the book database. The book to be deleted can be searched for by any attribute of the book. This means, the user may want to search for the book to be deleted by ID, title, author name, edition, year or ISBN number. Only one attribute will be used to search for the book. 2. The search to find the book to be deleted will be conducted by the binary search algorithm 3. These two features will be added to the programs below Input/output specifications: The input file specification is the same as the one from the code below. You can use your old bookinput.dat files. Program Operation: It will be identical to that of ones below. In addition, an extra menu option will be added for deleting records.
To get started with this, a fully-working code for the book class that solves the original problem below will be provided. You have to modify this code to address the requirements of this homework. Your solution must add the features asked for, and not remove any existing features from the program.
Example Run : bookinput.dat contains are below: 000001, Bjarne Stroustrup, The C++ Programming Language, 4, 2013, 0321563840 000002, Stephen Colbert, Stephen Colbert's Midnight Confessions, 1, 2017, 1501169009 000003, Tom Clancy, The Hunt for Red October, 2, 1984, 0425240339 000004, Gregory Dudek, Computational Principles of Mobile Robotics, 2, 2010, 0521692121 Program Execution (user input underlined): Database has 4 records. (A) Add Record (F) Find Record by author name (P) Print All Records (S) Sort Records (D) Delete Record (Q) Quit Program d Search by: 1.ID 2.Author Name 3.Title 4.Edition 5.Publication Year 6.ISBN 3 Enter title: The C++ Programming Language Record found and deleted! 000002, Stephen Colbert, Stephen Colbert's Midnight Confessions, 1, 2017, 1501169009 000004, Gregory Dudek, Computational Principles of Mobile Robotics, 2, 2010, 0521692121 000003, Tom Clancy, The Hunt for Red October, 2, 1984, 0425240339 Database has 3 records. (A) Add Record (F) Find Record by author name (P) Print All Records (S) Sort Records (D) Delete Record (Q) Quit Program d Search by: 1.ID 2.Author Name 3.Title 4.Edition 5.Publication Year 6.ISBN 2 Enter author name: Tom Clonker Record not found, not deleted! 000002, Stephen Colbert, Stephen Colbert's Midnight Confessions, 1, 2017, 1501169009 000004, Gregory Dudek, Computational Principles of Mobile Robotics, 2, 2010, 0521692121 000003, Tom Clancy, The Hunt for Red October, 2, 1984, 0425240339 Database has 3 records. For this problem, you have to submit three files: one HPP file containing the class declaration, a CPP file containing the class definition, and a driver file that includes everything.
Cpp, Hpp, and Main.cpp given
~~~~ Books.cpp
#include "books.hpp"
#include
#include
using std::string;
using std::ostream;
using std::istream;
using std::cout;
using std::cin;
// Getters and setters for each of the private fields.
int Book::getEdition() const {
return edition;
}
void Book::setEdition( int edition ) {
this->edition = edition;
}
string Book::getAuthor() const {
return authorName;
}
void Book::setAuthor( string aName ) {
authorName = aName;
}
string Book::getTitle() const {
return title;
}
void Book::setTitle( string tName ) {
title = tName;
}
string Book::getID() const {
return id;
}
void Book::setID( string sID ) {
if( id.length() == 6 ) id = sID;
}
string Book::getISBN() const {
return isbn;
}
void Book::setISBN( string sIsbn ) {
isbn = sIsbn;
}
ostream& operator<<( ostream &os, Book s ) {
os << s.id << "," << s.authorName << "," << s.title << "," << s.edition << "," << s.year << ","<< s.isbn ;
return os;
}
istream& operator>>( istream &is, Book &s ) {
string str="";
getline( is, str );
if( is.fail() ) {
return is;
}
std::stringstream jStream( str );
string id="", author="", title="", isbn="";
string edition="", year="";
getline( jStream, id, ',' );
getline( jStream, author, ',' );
getline( jStream, title, ',' );
getline( jStream, edition, ',' );
getline( jStream, year, ',' );
getline( jStream, isbn );
Book newBook( id, author, title, stoi(edition), stoi(year), isbn );
s = newBook;
return is;
}
Book::Book() {
id=authorName=title=isbn="";
edition=1;
year=1970;
}
Book::Book( string sid, string fName, string lName, int edition, int year, string isbn ) {
id=sid;
authorName=fName;
title=lName;
this->isbn=isbn;
this->edition=edition;
this->year = year;
}
~~~~Books.hpp
#ifndef __BOOKS_HPP_
#define __BOOKS_HPP_
#include
#include
using std::string;
using std::ostream;
using std::istream;
class Book {
string id;
string authorName; // Author name
string title; // book title
int edition; // edition
int year; // year published
string isbn; // ISBN 10-digit
public:
Book();
Book( string sid, string fName, string lName, int edition, int year, string isbn );
int getEdition() const;
void setEdition( int edition );
string getAuthor() const;
void setAuthor( string aName );
string getTitle() const;
void setTitle( string title );
string getID() const;
void setID( string sID );
int getYear() const;
void setYear( int year );
string getISBN() const;
void setISBN( string sIsbn );
friend ostream& operator<<( ostream &os, Book s );
friend istream& operator>>( istream &is, Book &s );
friend void Sort( Book bookList[], int size, short field );
};
#endif
~~~~Books_main.cpp
#include "books.hpp"
#include
using std::cerr;
using std::cout;
using std::cin;
using std::endl;
template
void Swap( T &a, T &b ) {
T temp =a;
a=b;
b=temp;
}
void Sort( Book bookList[], int size, short field ) {
for( int i = 0; i < size-1; i++ ) {
for( int j = i+1; j < size; j++ ) {
switch( field ) {
case 1:
if( bookList[i].id > bookList[j].id )
Swap( bookList[i], bookList[j] );
break;
case 2:
if( bookList[i].authorName > bookList[j].authorName )
Swap( bookList[i], bookList[j] );
break;
case 3:
if( bookList[i].title > bookList[j].title )
Swap( bookList[i], bookList[j] );
break;
case 4:
if( bookList[i].edition > bookList[j].edition )
Swap( bookList[i], bookList[j] );
break;
case 5:
if( bookList[i].year > bookList[j].year )
Swap( bookList[i], bookList[j] );
break;
case 6:
if( bookList[i].isbn > bookList[j].isbn )
Swap( bookList[i], bookList[j] );
break; }
}
}
}
int LoadDatabase( Book *books) {
std::ifstream infile("bookinput.dat");
if( infile.fail() )
return -1;
string iString="";
Book newBook;
int bookNum=0; //number of books in the input file.
while( infile >> newBook ) {
//Book newBook = BookMaker( iString );
books[bookNum++] = newBook;
}
if (bookNum==0 ) { // Empty file
cout << "File was empty! Exiting. ";
return 0;
}
infile.close();
return bookNum;
}
bool FlushToDatabase( Book *books, int size ) {
std::ofstream outFile("bookinput.dat");
if( !outFile ) {
cout << "Error writing to file! ";
return false;
}
for( int i=0; i < size; i++ ) {
outFile << books[i] << endl;
}
outFile.close();
return true;
}
int main() {
std::ofstream outfile("bookoutput.dat");
if( outfile.fail() ) {
cerr << "File I/O error! Exiting." << endl;
return 1;
}
char choice = 'y';
Book library[200]; //library that can hold 200 books.
int bookNum = LoadDatabase( library );
while( toupper( choice ) != 'Q' ) {
cout << "(A) Add Record\t (F) Find Record by author name (P) Print All Records\t(S) Sort Records (Q) Quit Program ";
cin >> choice;
cin.ignore();
switch( toupper(choice) ) {
case 'A': /* Add record */
{ cout << "Enter the record, with items seperated by a single comma: ";
Book newBook;
cin >> newBook;
library[bookNum++]=newBook;
cout << "Current database size: " << bookNum << " records." << endl;
break;
}
case 'F': /* Find and print record */
{
cout << "Enter author name: ";
string lName;
cin >> lName;
int i=0;
bool found=false;
for( i=0; i if( library[i].getAuthor().find( lName ) != std::string::npos ) { cout << library[i] < found = true; } } if( !found ) cout << "Record not found. "; break; } case 'P': /* Print all records */ { for( int i=0; i < bookNum; i++ ) { cout << library[i] < } break; } case 'S': /* Sort and print records, also send to output file */ { Book *sortedBooks = new Book[bookNum]; for( int i=0; i sortedBooks[i] = library[i]; char sortChoice =' '; cout << "Sort by: 1.ID 2.Author Name 3.Title 4.Edition 5.Publication Year 6.ISBN "; cin >> sortChoice; cin.ignore(); switch( sortChoice ) { case '1': Sort( sortedBooks, bookNum, 1 ); break; case '2': Sort( sortedBooks, bookNum, 2 ); break; case '3': Sort( sortedBooks, bookNum, 3 ); break; case '4': Sort( sortedBooks, bookNum, 4 ); break; case '5': Sort( sortedBooks, bookNum, 5 ); break; case '6': Sort( sortedBooks, bookNum, 6 ); break; default: break; } for( int i=0; i < bookNum; i++ ) { outfile << sortedBooks[i] << endl; cout << sortedBooks[i] << endl; } delete [] sortedBooks; break; } default: break; } } FlushToDatabase(library, bookNum ); outfile.close(); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
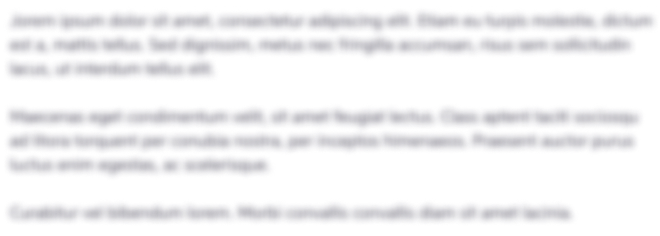
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started