Question
[c++] Useing code below. modify the code to solve the problem. Your code must include exception handling. implement a program that checks for balanced braces
[c++]
Useing code below. modify the code to solve the problem. Your code must include exception handling.
implement a program that checks for balanced braces using the ADT stack.
Your program will receive a string from the user and display whether the given string has balanced or unbalanced braces. For example, a string, abc{def}{ghik{lm, is unbalanced. A string abcd{efg{hijk}lmn}op is balanced. How can we check balance? The braces are balanced if
- Each time you encounter a "}", it matches an already encountered "{"
- When you reach the end of the string, you have matched each "{".
//main.cpp
#include
int main() {
const int SIZE = 5; IntFiniteStack s1(SIZE); // Stack empty test if (s1.isEmpty()) cout << "s1 is empty at the beginning." << endl; else cout << "s1 must be empty. Something's wrong!" << endl; for(int i = 0; i < SIZE; i++) s1.push(i); // Stack full test if (s1.isFull()) cout << "s1 is full after five push() calls." << endl; else cout << "s1 must be full. Something's wrong!" << endl; // top() and pop() test: reverses the items cout << "Expected: 4 3 2 1 0 -->" << endl; for (int i = 0; i < SIZE; i++) { cout << s1.top() << endl; s1.pop(); } // Stack empty test if (s1.isEmpty()) cout << "s1 is empty after five pop() calls." << endl; else cout << "s1 must be full. Something's wrong!" << endl;
// StackFullException test try { cout << "now, push() 6 times..." << endl; for(int i = 0; i < SIZE + 1; i++) s1.push(i); } catch (IntFiniteStack::StackEmptyException) { cout << "Exception: cannot pop, stack is empty" << endl; } catch (IntFiniteStack::StackFullException) { cout << "Exception: cannot push, stack is full" << endl; } // StackEmptyException test try { cout << "now, pop() 6 times..." << endl; for(int i = 0; i < SIZE + 1; i++) s1.pop();
} catch (IntFiniteStack::StackEmptyException) { cout << "Exception: cannot pop, stack is empty" << endl; } catch (IntFiniteStack::StackFullException) { cout << "Exception: cannot push, stack is full" << endl; }
return 0;
}
----------------------
//INTFINITESTACK.H
#ifndef INTFINITESTACK_H #define INTFINITESTACK_H
class IntFiniteStack { private: int* items; int size; // array size (capacity of stack) int p_index; // array index where a new data will be pushed public: class StackEmptyException{ }; // empty inner class for exception handling class StackFullException{ }; // empty inner class for exception handling IntFiniteStack(); IntFiniteStack(int size); virtual ~IntFiniteStack(); void push(int) ; void pop(); int top(); bool isEmpty(); bool isFull(); };
#endif
---------------
//INTFINITESTACK.cpp
#include "IntFiniteStack.h"
IntFiniteStack::IntFiniteStack() { p_index = 0; size = 10; items = new int[10]; //default size }
IntFiniteStack::IntFiniteStack(int size) { p_index = 0; this->size = size; items = new int[size]; }
// destructor: free all the memory allocated for the stack IntFiniteStack::~IntFiniteStack() { delete [] items; }
// push a data onto the stack void IntFiniteStack::push(int data) { if (isFull()) throw StackFullException();
items[p_index] = data; p_index++; // to combine two lines ==> items[p_index++] = data }
// pop the data from the stack void IntFiniteStack::pop() { if (isEmpty()) throw StackEmptyException();
--p_index; //don't have to delete data but just adjust the push index }
// read the data on the top int IntFiniteStack::top() { if (isEmpty()) throw StackEmptyException(); return items[p_index - 1]; // note: items[p_index] is for next push }
// is stack empty? bool IntFiniteStack::isEmpty() { return p_index == 0; }
// is stack full? bool IntFiniteStack::isFull() { return p_index == size; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
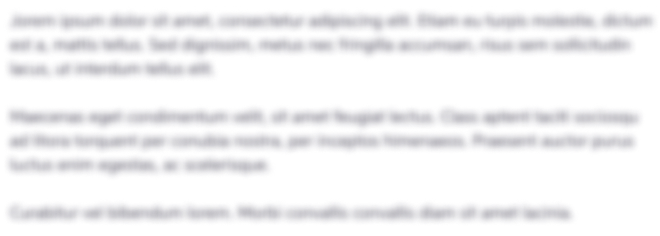
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started