Question
C# Visual Studio 2015 Windows Form, PLEASE READ BEFORE POSTING YOUR SOLUTION, IM TIRED OF WASTING QUESTIONS BECAUSE PEOPLE FAIL TO READ. I'm needing some
C# Visual Studio 2015 Windows Form, PLEASE READ BEFORE POSTING YOUR SOLUTION, IM TIRED OF WASTING QUESTIONS BECAUSE PEOPLE FAIL TO READ. I'm needing some help with my code, I have written the code for my Tic-Tac-Toe in Windows For, everything seems to work except for making the computer move. Whenever i run my application the MakeComputerMove Method is not working. Can someone please help me? I only need help with that method, i dont need help re-writing the whole thing. Once again please dont just copy and paste a generic solotuion since my methods work except fo that one. I have made the area where i need help in bold, if you do decide to write a generic code i will flag it as bad and report it to Chegg. I'm needing help but all my questions are being answered by people that dont even bother to read with what i need help with. Thank you
namespace TicTacToe { public partial class TTTForm : Form { public TTTForm() { InitializeComponent(); }
const string USER_SYMBOL = "X"; const string COMPUTER_SYMBOL = "O"; const string EMPTY = "";
const int SIZE = 5;
// constants for the 2 diagonals const int TOP_LEFT_TO_BOTTOM_RIGHT = 1; const int TOP_RIGHT_TO_BOTTOM_LEFT = 2;
// constants for IsWinner const int NONE = -1; const int ROW = 1; const int COLUMN = 2; const int DIAGONAL = 3;
// This method takes a row and column as parameters and // returns a reference to a label on the form in that position private Label GetSquare(int row, int column) { int labelNumber = row * SIZE + column + 1; return (Label)(this.Controls["label" + labelNumber.ToString()]); }
// This method does the "reverse" process of GetSquare // It takes a label on the form as it's parameter and // returns the row and column of that square as output parameters private void GetRowAndColumn(Label l, out int row, out int column) { int position = int.Parse(l.Name.Substring(5)); row = (position - 1) / SIZE; column = (position - 1) % SIZE; }
// This method takes a row (in the range of 0 - 4) and returns true if // the row on the form contains 5 Xs or 5 Os. // Use it as a model for writing IsColumnWinner private bool IsRowWinner(int row) { Label square = GetSquare(row, 0); string symbol = square.Text; for (int col = 1; col
//* TODO: finish all of these that return true private bool IsAnyRowWinner() { //UPDATED CODE for (int row = 1; row
private bool IsColumnWinner(int col) { //UPDATED CODE Label square = GetSquare(0, col); string symbol = square.Text; for (int row = 1; row
for (int diag = 1; diag
private bool IsAnyDiagonalWinner() { //UPDATED CODE Label square = GetSquare(0, (SIZE - 1)); string symbol = square.Text; for (int row = 1, col = SIZE - 2; row
// This method determines if any row, column or diagonal on the board is a winner. // It returns true or false and the output parameters will contain appropriate values // when the method returns true. See constant definitions at top of form. private bool IsWinner(out int whichDimension, out int whichOne) { // rows for (int row = 0; row
// I wrote this method to show you how to call IsWinner private bool IsTie() { int winningDimension, winningValue; return (IsFull() && !IsWinner(out winningDimension, out winningValue)); }
// This method takes an integer in the range 0 - 4 that represents a column // as it's parameter and changes the font color of that cell to red. private void HighlightColumn(int col) { for (int row = 0; row
// This method changes the font color of the top right to bottom left diagonal to red // I did this diagonal because it's harder than the other one private void HighlightDiagonal2() { for (int row = 0, col = SIZE - 1; row
// This method will highlight either diagonal, depending on the parameter that you pass private void HighlightDiagonal(int whichDiagonal) { if (whichDiagonal == TOP_LEFT_TO_BOTTOM_RIGHT) HighlightDiagonal1(); else HighlightDiagonal2();
}
//* TODO: finish these 2 private void HighlightRow(int row) { //UPDATED CODE 1 for (int col = 0; col
{ Label square = GetSquare(row, col); square.Enabled = true; square.ForeColor = Color.Red; } }
private void HighlightDiagonal1() { //UPDATED CODE 2 for (int row = SIZE - 1, col = 0; row
//* TODO: finish this private void HighlightWinner(string player, int winningDimension, int winningValue) { switch (winningDimension) { //UPDATED CODE case ROW: HighlightRow(winningValue); resultLabel.Text = (player + " wins!");
break; //UPDATED CODE case COLUMN: HighlightColumn(winningValue); resultLabel.Text = (player + " wins!");
break; case DIAGONAL: HighlightDiagonal(winningValue); resultLabel.Text = (player + " wins!"); break; } }
//* TODO: finish these 2 private void ResetSquares() { //UPDATED CODE for (int row = 0; row
private void MakeComputerMove() { Random gen = new Random(); int row; int column; Label square; do { row = gen.Next(0, SIZE); column = gen.Next(0, SIZE); square = GetSquare(row, column);
} while (square.Text != EMPTY); square.Text = COMPUTER_SYMBOL; DisableSquare(square); }
// Setting the enabled property changes the look and feel of the cell. // Instead, this code removes the event handler from each square. // Use it when someone wins or the board is full to prevent clicking a square. private void DisableAllSquares() { for (int row = 0; row
// Inside the click event handler you have a reference to the label that was clicked // Use this method (and pass that label as a parameter) to disable just that one square private void DisableSquare(Label square) { square.Click -= new System.EventHandler(this.label_Click); }
// You'll need this method to allow the user to start a new game private void EnableAllSquares() { for (int row = 0; row
//* TODO: finish the event handlers private void label_Click(object sender, EventArgs e) { //UPDATED CODE int winningDimension = NONE; int winningValue = NONE;
Label clickedLabel = (Label)sender; clickedLabel.Text = USER_SYMBOL; DisableSquare(clickedLabel); if (IsWinner(out winningDimension, out winningValue)) { HighlightWinner("The User", winningDimension, winningValue);
}
} private void button1_Click(object sender, EventArgs e) { }
private void button2_Click(object sender, EventArgs e) { this.Close(); } } }
303 305 306 307 308 310 311 312 313 315 317 318 319 private void MakeComputerMove() Random gen new Random int row; int column Label square now gen Next (0, SIZE) column gen Next (0 SIZE) square Getsquare (row, column while square. Text EMPTY) square Text COMPUTER SYMBOL Disable Square (square); 303 305 306 307 308 310 311 312 313 315 317 318 319 private void MakeComputerMove() Random gen new Random int row; int column Label square now gen Next (0, SIZE) column gen Next (0 SIZE) square Getsquare (row, column while square. Text EMPTY) square Text COMPUTER SYMBOL Disable Square (square)Step by Step Solution
There are 3 Steps involved in it
Step: 1
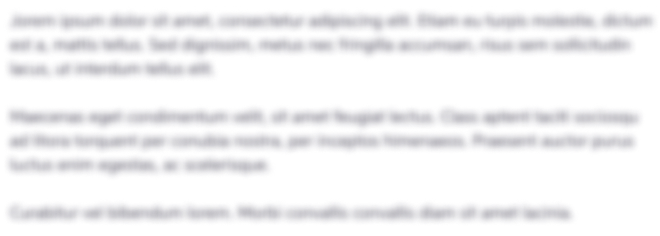
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started