Question
C++ Visual Studio Project 7: String Selection Sort Concepts tested by the program: Working with arrays Using file operations Using a selection sort to sort
C++ Visual Studio Project 7: String Selection Sort
Concepts tested by the program:
Working with arrays
Using file operations
Using a selection sort to sort string arrays
Using a function to display arrays
Using a function to save arrays to file
Implementing functions besides function main()
Project Description
Write a C++ program that processes a string data file. The program will 1) read the names from a string data file and place them in an array, and 2) sort the names in the array in ascending order using selection sort. The program will use a function to display the array before it is sorted and AFTER it is sorted. A separate function should be implemented to save the sorted names to a data file called sortedNames.txt.
Project Specifications
Input for this project: you may use the attached data file names.txt for testing, or a string data file of your own. Assume the file contains at least 20 names, each written on a separate line. The user must enter the number of names to process and names of the input file and output file.
Output: If the input file does not open, an error message should appear on the console. The program should have the follow output:
Display the names in the array before sorting (unsorted list)
Display the names in the array after sorting (sorted list)
Save the sorted array to a data file
Processing Requirements
Define all functions after main function and prototype them properly. Assume that the data file contains 20 names on separate lines and is constructed correctly.
Create functions for the following:
1. Read the names from the data file: Prompt for and get the input file name and try to open the file. If the file opens, read the names from the file, store them into the array. If the file does NOT open, print an error message on the console. If the file is not found, do NOT process the names.
2. Display the unsorted array. The string array should be a parameter.
3. Display the sorted array. The string array should be a parameter.
4. Save the sorted array to a data file. Prompt the user for data file name. The string array should be a parameter.
Sample Input File:
Collins, Bill
Smith, Bart
Allen, Jim,
Griffin, Jim
Stamey, Marty
Rose, Geri,
Taylor, Terri
Johnson, Jill,
Allison, Jeff
Looney, Joe
Wolfe, Bill,
James, Jean
Weaver, Jim
Pore, Bob,
Rutherford, Greg
Javens, Renee,
Harrison, Rose
Setzer, Cathy,
Pike, Gordon
Holland, Beth
Sample Output File:
Names in Ascending Order:
Allen, Jim,
Allison, Jeff
Collins, Bill
Griffin, Jim
Harrison, Rose
Holland, Beth
James, Jean
Javens, Renee,
Johnson, Jill,
Looney, Joe
Pike, Gordon
Pore, Bob,
Rose, Geri,
Rutherford, Greg
Setzer, Cathy,
Smith, Bart
Stamey, Marty
Taylor, Terri
Weaver, Jim
Wolfe, Bill,
Sample Screen Output:
I need my code to display the same output as the example of the screen outputs shown above.
#include
#include
#include
#include
using namespace std;
// function declarations
void sort(string names[], int count);
void display(string names[], int count);
void saveToFile(ofstream& dataOut, string names[], int count);
int main()
{
// declaring variables
string name;
int count = 0;
// defines an input stream for the data file
ifstream dataIn;
// Defines an output stream for the data file
ofstream dataOut;
// Opening the input file
dataIn.open("namesFile.txt");
// checking whether the file name is valid or not
if (dataIn.fail())
{
cout
return 1;
}
else
{
while (getline(dataIn, name))
{
count++;
}
dataIn.close();
// Creating array dynamically
string* names = new string[count];
// Opening the input file
dataIn.open("namesFile.txt");
for (int i = 0; i
{
getline(dataIn, name);
names[i] = name;
}
dataIn.close();
cout
cout
// calling the functions
display(names, count);
sort(names, count);
cout
cout
display(names, count);
// creating and Opening the output file
dataOut.open("sortedNames.txt");
saveToFile(dataOut, names, count);
// Closing the output file.
dataOut.close();
}
system("pause");
return 0;
}
// This function will sort the String array
void sort(string names[], int count)
{
int start;
string temp;
for (int i = count - 1; i > 0; --i)
{
start = 0;
for (int j = 1; j
{
if (names[j].compare(names[start]) > 0)
{
start = j;
}
temp = names[start];
names[start] = names[i];
names[i] = temp;
}
}
}
// This function will display the string array
void display(string names[], int count)
{
for (int i = 0; i
{
cout
}
}
// This function will save the sorted array to outfile
void saveToFile(ofstream& dataOut, string names[], int count)
{
dataOut
for (int i = 0; i
{
dataOut
}
}
? X C:Windows system32\cmd.exe Please enter the name of the file to read names from: names. txt Enter number of names to read 20 Here are the unsor ted names: Collins, Bill Smith, Bart Allen, Jim, Griffin, Jim Stamey, Marty Rose, Geri, Taylor, Terri Johnson, Jill, Allison, Jeff Looney, Joe Wolfe, Bill, James, Jean Weaver, Jim Pore, BobStep by Step Solution
There are 3 Steps involved in it
Step: 1
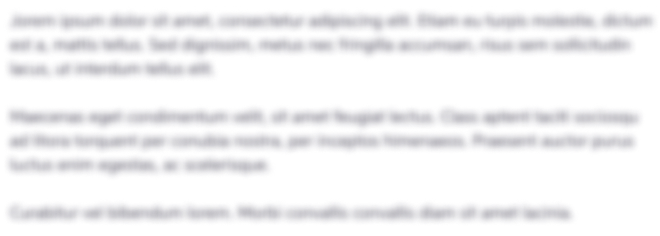
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started