Question
C++ War Card game program: Lab Instructions Create 3 files: lab9.cpp, Card.cpp, Card.h. Your goal is to design and implement the card game of War.
C++ War Card game program:
Lab Instructions Create 3 files: lab9.cpp, Card.cpp, Card.h.
Your goal is to design and implement the card game of War. Here are the requirements:
Card.cpp + Card.h
In the header file, create a new CPP class Card. Make sure to use header guards and best CPP practices while writing your class. The Card class should make use of the following enum provided for you:
public: enum class Suit { HEARTS=0, DIAMONDS=1, SPADES=2, CLUBS=3 };
Place this code inside the class definition as a public member. You have covered enums in class; in this case we will use them as a handy way for describing card suits. Notice that we explicitly give each enum an integer value. This is not strictly necessary, but useful if we wanted to do something like sort cards by suit using std::sort. Your card class should have a Suit data member suit whose type is the enum above. To access members of this enum, we must use class level scope. For example, to get the enum value of hearts we would use the code:
Card::Suit::HEARTS
The card class should also have an integer value. Your cards will run aces high. This means the lowest valued card is a 2 and the highest (ace) is a 14. Your card class should define 2 constructors. The first is a default constructor, which creates a default card 2 of hearts. The second constructor takes an integer value and a Suit enum as arguments to create the card. Your card class should define getters and setters for both data members. You will also write a print method which returns a string representation of a card. How you print the cards is up to you, but you could include a switch statement like this:
switch (this->suit) {
case Card::Suit::HEARTS: out << "\u2665"; break;
case Card::Suit::DIAMONDS: out << "\u2666"; break;
case Card::Suit::SPADES: out << "\u2660"; break;
case Card::Suit::CLUBS: out << "\u2663"; break;
}
To print out the Unicode characters for card suit. https://en.wikipedia.org/wiki/Playing_cards_in_Unicode
All implementation of the Card class should be done in Card.cpp. Card.h is only for defining the class.
lab9.cpp
Your main method should create two arrays of type Card, each with 52 elements. You must populate these arrays with a standard deck of cards, i.e. 2-Ace of hearts, spades, clubs, and diamonds. Once youve created the decks, make two calls to random_shuffle from the standard library. This will randomly shuffle your decks. This function is used in much the same way as std::sort, refer to the link above for more info. Then, after your decks are shuffled, make a game loop. This loop should iterate 52 times, comparing the card from the first deck against the card in the second. High value wins, same values draw. Keep track of 3 integer variables, which represent the number of times the first deck wins, the number of times the second deck wins, and the number of draws.
Output
Your program should output the cards played and the result of each hand to the console. You should also output the values from the game loops (number of wins and draws). Output should look something like this:
J J Draw
10 5 P1 Wins
Q 8 P1 Wins
9 J P2 Wins
. . . (skip some lines)
10 K P2 Wins
3 Q P2 Wins
Q 5 P1 Wins
7 9 P2 Wins
2 2 Draw
9 6 P1 Wins
4 3 P1 Wins
J 9 P1 Wins
A A Draw
P1 Total Wins = 23
P2 Total Wins = 21
Draws = 8
Step by Step Solution
There are 3 Steps involved in it
Step: 1
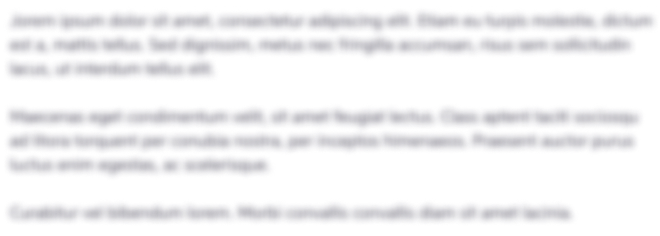
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started