Answered step by step
Verified Expert Solution
Question
1 Approved Answer
C++ What is the problem with the code snippet below? void cost(int price, int reps) { for (int i = 0; i < reps; i++)
C++
- What is the problem with the code snippet below?
void cost(int price, int reps)
{
for (int i = 0; i < reps; i++)
{
cout << price;
}
return;
}
int main()
{
cout << cost(10, 4) << endl;
return 0;
}
- The function cost is invoked with the wrong parameters
- The function cost uses uninitialized variables
- The function cost returns void and therefore cannot be used in a cout statement
- The function cost must return an integer value
- Which of the following is true about functions?
- Functions can have only one parameter and can return only one return value.
- Functions can have multiple parameters and can return multiple return values.
- Function can have multiple parameters and can return one return value.
- Functions can have one parameter and can return multiple return values.
- Consider a function named calc, which accepts two numbers as integers and returns their sum as an integer. Which of the following is the correct statement to call the function calc?
- calc(2, 3.14);
- int sum = calc(2, 3);
- calc();
- int sum = calc("2", "3");
- How are variables passed as input to a function?
- By using the return statement
- By using arguments
- By using comments
- By using the function name
- Consider the function shown below.
int do_it(int x, int y)
{
int val = 0;
if (x < y)
{
val = x + 1;
}
else
{
val = y - 1;
}
// the last statement goes here
}
What should be the last statement of this function?
- An assignment statement
- A return statement
- An increment statement
- A comparison statement
- Which of the following is true about function return statements?
- A function can hold multiple return statements, but only one return statement executes in one function call.
- A function can hold only one return statement.
- A function can hold multiple return statements, and multiple return statements can execute in one function call.
- A function can have maximum of two return statements.
- Which of the following is the best choice for a return type from a function that prompts users to enter their password?
- char
- int
- string
- void
- In the following code snippet, what is the scope of variable b?
void func1()
{
int i = 0;
double b = 0;
}
void func2()
{
}
int main()
{
func1();
func2();
return 0;
}
- It can be used only in func1().
- It can be used in user-defined functions, func1() and func2().
- It can be used anywhere in this program.
- It can be used in many programs.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
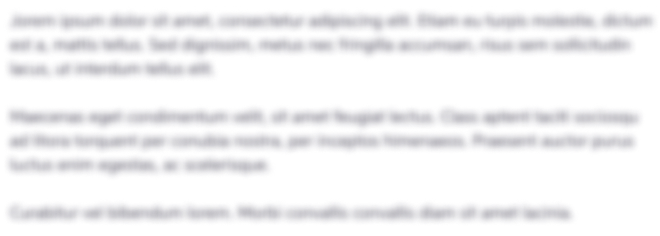
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started