Question
C++ Why Doesnt this run? Please hurry its due tonight the problem lies on binSTree.h! Thank You! //binSTree.h #ifndef BINSTREE_H #define BINSTREE_H #include #include binTree.h
C++
Why Doesnt this run? Please hurry its due tonight the problem lies on binSTree.h!
Thank You!
//binSTree.h
#ifndef BINSTREE_H
#define BINSTREE_H
#include
#include "binTree.h"
#include "Node.h"
#include
#include
#define N=100
using namespace std;
template
class binSTree : public binTree
public:
void insert(const T& x); // inserts node with value x
bool search(const T& x); // searches leaf with value x
bool remove(const T& x); // removes leaf with value x
private:
void insert(Node < T >*&, const T&); // private version of insert
bool search(Node < T >*, const T&);// private version of search
void remove(Node < T >*&, const T&); // private version of remove
bool leaf(Node < T >* node) const; // checks if node is leaf
};
//public functions
//public insert calls private insert
template
void binSTree
//public search calls private search
template
bool binSTree
//public remove removes leaf with the value of x
template
bool binSTree
if (this->size() == 1)
{
this->root = NULL;
return false;
}
if (this->size() > 1)
{
if (search(x)) this->root = remove(this->root, x);
return true;
}
else if (this->size() == 1) { return false; }
else { return false; }
}
//private functions
//private version of insert
template
void binSTree
if (p == NULL) { p = new binTreeNode
else if (v < p->data) { insert(p->left, v); }
else if (v > p->data) { insert(p->right, v); }
else { return; }
}
//private search
template
bool binSTree
if (p == NULL) return false;
else
{
if (v == p->data)
{
if (leaf(p)) return true;
else return false;
}
else if (v < p->data)
return search(p->left, v);
else
return search(p->right, v);
}
}
//private remove
template
void binSTree
binTreeNode
binTreeNode
curr = p;
while (curr != NULL)
{
if (curr->data == v) { break; }
else
{
parent = curr;
//if the value is more, curr will point to the right node
if (v > curr->data) { curr = curr->right; }
//if not, curr will point to the left one
else { curr = curr->left; }
}
}
if (curr != NULL)
{
if (parent->right == curr) parent->right = NULL;
else parent->left = NULL;
delete curr;
curr = NULL;
}
if (this->size() >= 1) { return this->root; }
return this->root;
}
//private leaf
template
bool binSTree
//if the node is a leaf
if (p->left == NULL && p->right == NULL) { return true; }
//if the node is not a leaf
else { return false; }
}
#endif
//binTree.h
#ifndef H_PROG6
#define H_PROG6
#include "Node.h"
#include
using namespace std;
template < class T > class binTree {
public:
binTree(); // default constructor
unsigned height() const; // returns height of tree
virtual void insert(const T&v); // inserts node in tree
void inorder(void(*fn)(T&)); // inorder traversal of tree
protected:
Node < T >* root; // root of tree
private:
unsigned height(Node < T >*) const; // private version of height ( )
void insert(Node < T >*&p, const T&v); // private version of insert ( )
void inorder(Node < T >*p, void(*fn) (T&));// private version of inorder ( )
};
//type definition for side
typedef enum { left_side, right_side } SIDE;
SIDE rnd() { return rand() % 2 ? right_side : left_side; }
//default consturctor definition
//initializes root
template
binTree
{
root = 0;
}
//public function definitions
//returns the height of the tree
template
unsigned binTree
{
return height(root);
}
//calls private insert function
template
void binTree
{
insert(root, v);
}
//calls private inorder function
template
void binTree
{
inorder(root, fn);
}
//privae function definitions
template
unsigned binTree
{
//the tree is empty
if (p == 0)
{
return 0;
}
else
{
//left side
int lHeight = height(p->left);
//right side
int rHeight = height(p->right);
//determines which is side has the greater height
if (lHeight > rHeight)
{
return 1 + lHeight;
}
else
{
return 1 + rHeight;
}
}
}
//inserts a value into a node
template
void binTree
if (p == nullptr)
{
//sets p to a new node
p = new Node
}
else
{
//randomizes the side variable
SIDE s = rnd();
//condition to put value into either left or right
if (s == left_side)
{
insert(p->left, v);
}
else
{
insert(p->right, v);
}
}
}
//prints the tree inorder
template
void binTree
if (p != NULL)
{
inorder(p->left, fn);
fn(p->data);
inorder(p->right, fn);
}
}
#endif
//Node.h
#ifndef H_NODE
#define H_NODE
// definition for class of nodes in bin tree
template < class T > class binTree; // forward declaration
template < class T > class binSTree; // forward declaration
template < class T >
class Node {
friend class binTree < T >; // binTree is friend
friend class binSTree < T >; // binSTree is friend
public:
// default constructor
Node(const T& x = T(), Node < T >* l = 0, Node < T >* r = 0) :
data(x), left(l), right(r) { }
private:
T data; // data component
Node < T > *left, *right; // left and right links
};
#endif
//prog7.h
#include
#include
#include
#include
using namespace std;
#ifndef H_PROG7
#define H_PROG7
#define SEED 1 // seed for RNG
#define N 100 // num of rand ints
// class to generate rand ints
class RND {
private:
int seed;
public:
RND(const int& s = SEED) : seed(s) { srand(seed); }
int operator ( ) () { return rand() % N + 1; }
};
#define NO_ITEMS 16 // max num of elems printed in line
#define ITEM_W 3 // size of each elem on printout
unsigned sz; // size of vector
// macro to print size
#define COUT_SZ cout << "size = " << setw ( ITEM_W ) << sz << " :"
// function to print elems on stdout
template < class T > void print(T& x)
{
static unsigned cnt = 0;
const string sp(12, ' ');
cout << setw(ITEM_W) << x << ' '; cnt++;
if (cnt % NO_ITEMS == 0 || cnt == sz) cout << endl << sp;
if (cnt == sz) cnt = 0;
}
#endif
//prog7.cc
#include "prog7.h"
#include "BinSTree.h"
// This program generates bunch of rand ints in given range
// and stores them in vector, and it also inserts them in
// bin search tree. Then, removes all leaves in tree and
// repeat this process until tree becomes empty.
int main()
{
vector < int > v(N); // holds rand ints
binSTree < int > t; // bin search tree ( BST )
// generate rand ints
generate(v.begin(), v.end(), RND());
// printout contents of vector
sz = v.size(); COUT_SZ;
for_each(v.begin(), v.end(), print < int >); cout << endl;
// insert ints in vector into BST
for (unsigned i = 0; i < v.size(); i++) t.insert(v[i]);
// remove leaves of BST until it becomes empty
bool flag = true; // to check if BST empty
while (flag)
{
// printout contents of BST
sz = t.size(); COUT_SZ;
t.inorder(print < int >); cout << endl;
// remove all leaves of BST
flag = false;
for (unsigned i = 0; i < v.size(); i++)
if (t.remove(v[i])) flag = true;
}
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
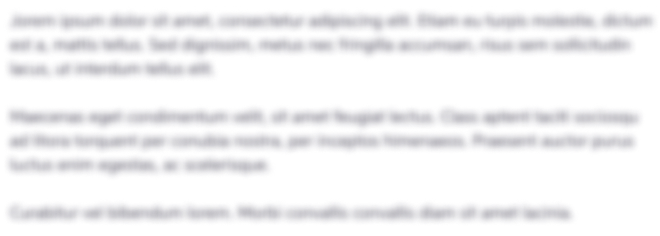
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started