Question
C++ , Write a program which simulates a lottery, allowing any number of players to participate in the lottery game. The program should declare, in
C++, Write a program which simulates a lottery, allowing any number of players to participate in the lottery game.
The program should declare, in main, an array of five integers named lottery and a vector of integers named user.
Then have the user enter 5 different digits in an overloaded function to fill the lottery array. Any number of players can now play the lottery.
In main have the user enter a lottery player's full name, and have the user enter 5 different integers in a second version of the overloaded function to fill the vector. Validate that all digits for the array and vector are neither negative nor greater than 9.
In main have your program match the numbers in the array to those in the vector to determine which numbers in the user vector match any of the numbers in the lottery array (i.e., not just the numbers in "corresponding" positions).
Display in main the player's name, the user vector, the number of matching digits, and the digits which match, and display a congratulatory "Grand Prize" message, with the user's name, if all digits match. Then have another player try the lottery, or quit the game.
You may not sort the array nor the vector, and must use loops to process both.
Test data: -the lottery array consists of: 7, 4, 9, 1, 3 -name: Iam A. Gambler, with user vector: 0, 2, 9, 6, 3 -name: Help Imhooked, with user vector: 1, 9, 7, 3, 4
Provide two screen prints:
I need assistance with the last part of this problem. It is bolded in the last paragraph. I am not sure how to iterate through an array and vector to see matching digits.
================================================== My current code:
#include
#include
#include
#include
using namespace std;
void fill(int arr[], const int SIZE);
void fill(vector &user, const int SIZE);
void Display_All(int arr[], vector );
int main() {
//initialize variables
string playerName;
const int SIZE = 5;
int num, lottery[SIZE], temp;
vector user;
char playerNew = 'y';
//enter 5 digits in an overloaded funtion to fill lottery array
fill(lottery, SIZE);
while (playerNew != 'n' && playerNew != 'N') {
//enter players name
cin.ignore();
cout << " Please enter players full name: ";
getline(cin, playerName);
cout << endl;
//enter 5 integers in second Overloaded Function to fill vector (user selected numbers)
fill(user, SIZE);
//calculations to match numbers in array to those in vector
//OUTPUT
//display user name
cout << " Player name is: " << playerName;
//display user vector
cout << " Players number selections were: ";
for (int i = 0; i < SIZE; i++) {
cout << "choice " << i + 1 <<": " << user[i] << endl;
}
//# of matching digits
//the digits that match are:
//if ALL match output grand prize / else sorry you lost
//Play again or quite? End the loop
cout << " Want to continue: (y/n) ";
cin >> playerNew;
if (playerNew == 'y') {
cout << " Welcome again! ";
}
else {
cout << " QUITING...";
}
}
cout << endl << endl;
system("pause");
return 0;
}
void fill(int arr[], const int size)
{
int lotteryNum;
cout << "Enter 5 digits into the lottery array." << endl << endl;
for (int i = 0; i < size; i++)
{
cout << "Enter number " << i + 1 << ": ";
cin >> lotteryNum;
arr[i] = lotteryNum;
//validation
while (lotteryNum < 0 || lotteryNum > 9) {
cout << "Invalid number, enter a digit between 0 and 9";
cout << " Please re-enter " << i + 1 << ": ";
cin >> lotteryNum;
}
}
}
void fill(vector &user, const int SZ)
{
int userNum;
cout << "Enter your selected numbers" << endl;
for (int i = 0; i < SZ; i++)
{
cout << "Enter selection #" << i + 1 << ": ";
cin >> userNum;
user.push_back (userNum);
//validation
while (user[i] < 0 || user[i] > 9) {
cout << "Invalid number, enter a digit between 0 and 9";
cout << " Please re-enter " << i + 1 << ": ";
cin >> user[i];
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
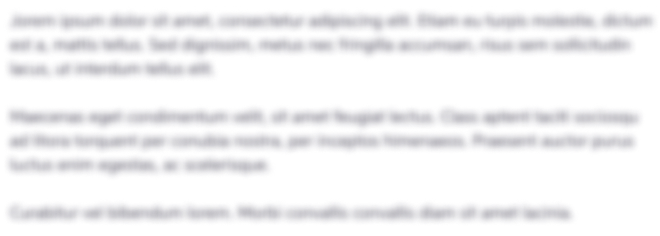
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started