Question
C++ Write a recursive function called void reverse ( ) that reverses a sentence. You need to create a class called Sentence that includes the
C++ Write a recursive function called void reverse ( ) that reverses a sentence. You need to create a class called Sentence that includes the recursive function called reverse along with a display function and a default constructor for the class. It is up to you as the programmer to determine the private member variables. Your program should create an Object in the main function and call reverse string function. For example:
Sentence greeting = new Sentence ("Hello!"); greeting.reverse ( ); cout << greeting.get_text() << " ";
Display on the Screen: !olleH
Note: Make sure the reverse string function does implement a recursive solution and does not include a for or while loop.
Modify the Object Oriented program you just completed so that your program is able to read several different sentences from a file then make a call using the greeting object so that each sentence will display in reverse order. Use your own test case sentences to test your program.
#include #include #include #include
using namespace std;
const int SIZE = 1000;
class Sentence { public: char* arrayOne;
Sentence(char* arrayTwo) { arrayOne = (char*)malloc(sizeof(char)*(strlen(arrayTwo) + 1)); strcpy_s(arrayOne, strlen(arrayOne), arrayTwo); }
char* get_text() { return arrayOne; }
void reverse() { reverseArray(arrayOne, 0, strlen(arrayOne) - 1); }
void reverseArray(char* array, int x, int y) { if (x < y) { char temp = array[x]; array[x] = array[y]; array[y] = temp; reverseArray(array, x + 1, y - 1); } } };
int main() { char *arrayStringPointer = new char[SIZE];
ifstream inFile; inFile.open("data.txt");
while (inFile >> arrayStringPointer) { Sentence greeting(arrayStringPointer); greeting.reverse(); cout << greeting.get_text() << " "; }
delete[] arrayStringPointer; arrayStringPointer = NULL;
inFile.close();
system("pause"); return 0; }
It compiles the first time, but the second time I get a .exe has triggered a breakpoint.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
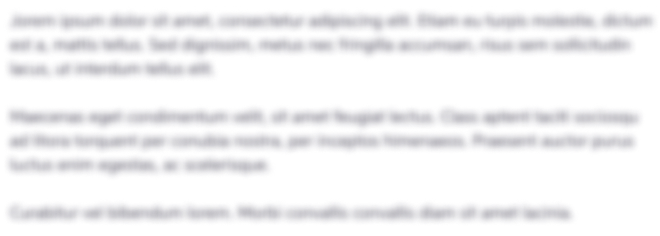
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started