Question
C++ Write a two-player tic-tac-toe program that asks users to input the row and column for each move they want to make and prints the
C++
Write a two-player tic-tac-toe program that asks users to input the row and column for each move they want to make and prints the board after each move. Initialize a 3 by 3 two dimensional char array with a space character as the default. Your program should perform input validation to make sure the move is not out of bounds and that the move has not already been used. Include userInput to take the users move, and checkWinner to check if a player has won, and printBoard to display the current gameboard. If a player wins, print a message stating who won and exit the program. If nine moves are made and there is no winner, announce a tie and exit the program.
Use classes and constructors. Header, implementation, and driver file.
Example Outputs:
[ ][ ][ ]
[ ][ ][ ]
[ ][ ][ ]
Player X, enter the row youd like to play: 1
Enter the column youd like to play: 1
[ ][ ][ ]
[ ][X][ ]
[ ][ ][ ]
Player O, enter the row youd like to play: 1
Enter the column youd like to play: 1
That moves been taken, please enter a valid move. . . . (game continues until ends)
Some scenario during the game
[O][ ][ ]
[O][X][ ]
[ ][X][ ]
Player X, enter the row youd like to play: 0
Enter the column youd like to play: 1
[O][X][ ]
[O][X][ ]
[ ][X][ ]
Player X wins!
This is what I have so far:
#include
//header file
class TicTacToe { private: char arr[3][3];
public: void DrawBoard(char **arr); void PrintBoard(); void GetMove(char **arr, char ch); void TogglePlayer(char player); bool DetermineDraw(); char checkWinner(char **arr); };
//implementation file void TicTacToe::DrawBoard(char **arr) { arr = new char*[3]; for(int i = 0; i < 3; ++i){ arr[i] = new char[3]; for(int j = 0; j < 3; ++j){ arr[i][j] = ' '; } } }
void TicTacToe::GetMove(char **arr, char ch)
{ int row; int col; while(true){ while(true){ cout << "Player " << ch << ", enter the row youd like to play: "; cin >> row; if(row < 0 || row > 2){ cout << "Invalid input. Try again" << endl; } else break; } while(true){ cout << "Enter the column youd like to play: "; cin >> col; if(col < 0 || col > 2){ cout << "Invalid input. Try again" << endl; } else break; } if(arr[row][col] != ' ') cout << "Invalid input. Try again" << endl; else break; } arr[row][col] = ch; }
char TicTacToe :: checkWinner(char **arr) { // check rows for(int i = 0; i < 3; ++i){ char ch = arr[i][0]; bool equal = true; for(int j = 0; j < 3; ++j){ if(arr[i][j] != ch){ equal = false; break; } } if(equal && ch != ' ') return ch; } // check columns for(int i = 0; i < 3; ++i){ char ch = arr[0][i]; bool equal = true; for(int j = 0; j < 3; ++j){ if(arr[j][i] != ch){ equal = false; break; } } if(equal && ch != ' ') return ch; } if(arr[0][0] == arr[1][1] && arr[1][1] == arr[2][2] && arr[0][0] != ' ') return arr[0][0]; if(arr[0][2] == arr[1][1] && arr[1][1] == arr[2][0] && arr[0][2] != ' ') return arr[0][2]; return ' '; }
void TicTacToe::TogglePlayer(char player) { if (player == 'X') player = 'O'; else if(player == 'O') player = 'X'; }
void TicTacToe:: PrintBoard() { for(int i = 0; i < 3; ++i){ for(int j = 0; j < 3; ++j){ cout << "[" << arr[i][j] << "]"; } cout << endl; } cout << endl; }
//driver file int main() { TicTacToe game; char player = 'X'; while(game.DetermineDraw() == false) { TicTacToe.DrawBoard(); TicTacToe.GetMove(player); TicTacToe.TogglePlayer(player); }
system("pause"); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
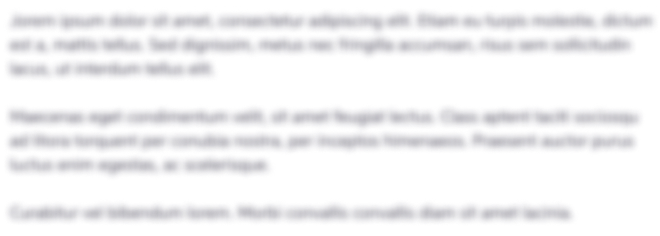
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started