Question
(C++) Write the single linked list using the following information. LINKED_LIST.H #ifndef LINKED_LIST_H #define LINKED_LIST_H #include node.h #include namespace lab0 { class linked_list { node
(C++) Write the single linked list using the following information.
LINKED_LIST.H
#ifndef LINKED_LIST_H
#define LINKED_LIST_H
#include "node.h"
#include
namespace lab0 {
class linked_list {
node *head, *tail;
public:
linked_list();
explicit linked_list(std::string &data);
linked_list(const linked_list &original);
virtual ~linked_list();
linked_list &operator=(const linked_list &RHS);
friend std::ostream& operator<<(std::ostream& stream, linked_list& RHS);
friend std::istream& operator>>(std::istream& stream, linked_list& RHS);
bool isEmpty() const;
unsigned listSize() const; //Note that you do **not** have a size variable in your linked list. You *MUST* count the nodes.
std::string get_value_at(unsigned location);
void insert(const std::string input, unsigned location = 0 ); //create a node from the input string and put it into the linked list at the given location
void append(const std::string input); //create a new node and put it at the end/tail of the linked list
void remove(unsigned location = 0); //Remove the node at the given location
void sort(); //Perform selection sort on the linked list
};
}
#endif
LINKED_LIST.CPP
#include
namespace lab0 {
linked_list::linked_list() { }
linked_list::linked_list(std::string &data) { }
linked_list::linked_list(const linked_list &original) { }
linked_list::~linked_list() { }
linked_list &lab5::linked_list::operator=(const linked_list &RHS) { }
bool linked_list::isEmpty() const { return false; }
unsigned linked_list::listSize() const { return 0; } //Note that you do **not** have a size variable in your linked list. You *MUST* count the nodes.
void linked_list::insert(const std::string input, unsigned int location) { //create a node from the input string and put it into the linked list at the given location
node *prev; node *current; node *temp = new node (input); current = head; }
void linked_list::append(const std::string input) { } //create a new node and put it at the end/tail of the linked list
void linked_list::remove(unsigned location) { } //Remove the node at the given location
std::ostream& operator<<(std::ostream &stream, linked_list &RHS) { return stream; }
std::istream& operator>>(std::istream &stream, linked_list &RHS) { return stream; }
void linked_list::sort() { } //Perform selection sort on the linked list
std::string linked_list::get_value_at(unsigned location) { }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
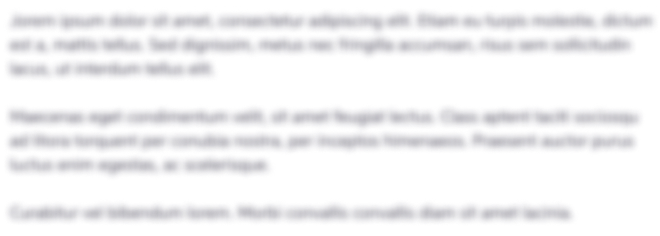
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started