Question
C++ You are creating a program for a JellyBelly factory to keep up with jellybean inventory. Your program will load current inventory from a text
C++ You are creating a program for a JellyBelly factory to keep up with jellybean inventory. Your program will load current inventory from a text file and then allow the user to choose from a menu. The user may print the inventory, add to inventory, delete from inventory, or save the inventory and exit the program.
You will create one structure named Jellybean that will hold the following information about a kind of jellybean:
Flavor (Example: A&W Root Beer)
Color: (Example: brown)
Star rating from consumers (Example: 4.5)
Price per pound of this kind (Example: 8.99)
Quantity (in pounds) in stock (Example: 480)
Below you will find details of what should happen in each function. Make sure to follow the specifications!
FUNCTION: MAIN
Create a 50-element Jellybean array.
You will need an integer variable that will keep track of how many jellybean kinds are in inventory at the current time.
Load the inventory. All jellybean kinds that are currently in inventory (in stock) are written in jellybellyinventory.txt Your program will read the text file within a loop, placing each piece of data in the correct element of the structure array. [provided for you]
Call the displayMenuGetChoice function to display the menu and get the users choice. Your program should continue to do this until the user chooses #5.
Call the appropriate function based on the users choice.
If they chose 1, call the printAllInventory function.
IF they chose 2, call the printColor function
If they chose 3, call the addBeans function
If they chose 4, call the deleteBeans function
Save all data in the Jellybean array to the text file jellybellyInventory.txt. Each piece of data should be separated by a * character so that it can then be read again by the program at a later date.
This function is provided for you and aids in reading the data from the file by converting strings to numbers so that the numbers can be placed in the structure member that has a number data type.
FUNCTION: DISPLAYMENUGETCHOICE
This function should print the menu, get the users choice, validate the users choice, and then return the users choice. Here is what the menu should look like:
This function should print all data currently stored in the Jellybean structure in a readable way.
This function should first ask the user what color jellybeans they want to print from inventory. It should then print out all the possible colors: beige, black, brown, blue, green, multi-colored, orange, pink, purple, red, white, and yellow. Then, read in the users color choice. Then, print all jellybean kinds from the Jellybean array that are of that color. If there were no jellybean types in inventory of that color then print Sorry, there were no jellybean types in inventory with that color.
This function will allow the user to add a new type of jellybean. Allow the user to enter in the flavor, color, rating, price per pound, and quantity in pounds. You should make sure this function adds one to the current number of jellybean kinds in inventory and return the updated number of kinds from this function.
This function will allow the user to delete or remove a kind of jellybean from inventory. If a kind was successfully removed, then make sure this function subtracts one from the current number of jellybean kinds in inventory. Return the updated number of kinds from this function.
jellybelly.h
jellybelly.cpp
functions.cpp
jellybellyinventory.txt
#ifndef JELLYBELLY_H
#define JELLYBELLY_H
#include
#include
#include
#include
#include
using namespace std;
struct Jellybean
{
//FIXME add memebers
};
const int NUM_FLAVORS = 50;
float stringToFloat(string);
int deleteBeans(Jellybean[], int);
//FIXME - add other function prototypes
#endif
#include "jellybelly.h"
int main()
{
//FIXME - create a 50-element Jellybean array (USE THE PROVIDED GLOBAL CONSTANT!)
ifstream inFile;
string data;
int currentNum = 0; //keeps track of how many kinds of jellybeans are in inventory right now
cout << " Welcome to JellyBelly Factory! ";
//Load the inventory
inFile.open("jellybellyInventory.txt");
if(inFile.good())
{
getline(inFile, data, '*');
while(!inFile.eof() && currentNum != NUM_FLAVORS)
{
jellybeanArray[currentNum].flavor = data;
getline(inFile, jellybeanArray[currentNum].color, '*');
getline(inFile, data, '*');
jellybeanArray[currentNum].starRating = stringToFloat(data);
getline(inFile, data, '*');
jellybeanArray[currentNum].pricePerLb = stringToFloat(data);
getline(inFile, data, '*');
jellybeanArray[currentNum].quantityLbs = stringToFloat(data);
currentNum++;
getline(inFile, data, '*');
}
cout << " There are currently " << currentNum << " items in inventory. ";
inFile.close();
}
else{
cout << " There are currently no items in inventory. ";
}
do
{
//FIXME - call displayMenuGetChoice
//FIXME - based on choice, call correct function!
} while(choice != 5);
cout << " Saving inventory.... ";
//FIXME - open inventory text file and print all data from array, separated by a *
cout << "Goodbye! ";
return 0;
}
#include "jellybelly.h"
//FIXME - add all other programmer-defined function definitions in this file!!!
/*
Function: stringToFloat
Purpose: This funciton accepts a string, converts the string to
a c-string, and then converts the c-string to a float.
Then, returns this float from the function.
*/
float stringToFloat(string str)
{
float number;
char convertStr[1000];
strcpy(convertStr, str.c_str()); //convert the string to c-string
number = atof(convertStr);
return number;
}
/*
Function: deleteBeans
Purpose: This funciton will allow the user to delete or remove a kind
of jellybean from inventory. If kind was successfully removed
then this function subtracts one from the current number of
kinds in inventory and returns this integer from the function.
*/
int deleteBeans(Jellybean jellybelly[], int num)
{
string flavorChoice;
bool removed = false;
cout << " Choose a jellybelly kind to remove from inventory! ";
for(int x=0; x { cout << jellybelly[x].flavor << " "; } cout << " FLAVOR TO DELETE: "; cin.ignore(); getline(cin, flavorChoice); for(int x=0; x { if(flavorChoice == jellybelly[x].flavor) { removed = true; for(int y=x; y<(num-1); y++) { jellybelly[y] = jellybelly[y+1]; } } } if(removed == true) { num--; cout << flavorChoice << " was removed from the inventory. "; } else cout << flavorChoice << " does not exist in the inventory. "; return num; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
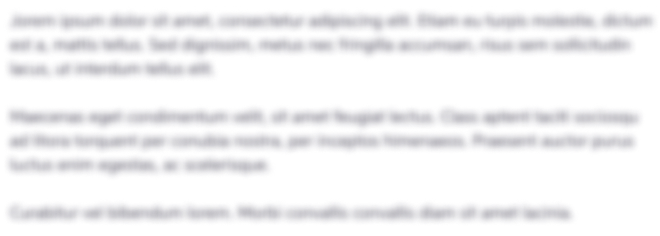
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started