Question
C++ You are to compare two sorting algorithms and compare two searching algorithms by running the algorithms and collecting data on each. Your data for
C++
You are to compare two sorting algorithms and compare two searching algorithms by running the algorithms and collecting data on each. Your data for sorting and searching will be strings of 20 characters in length. Have your program run, compute and print all the results in one run one execution, not multiple runs. (You are to run your program one time for all data sets.) In order to test sort algorithm, we will need to run each sort algorithms on different size data sets.
First Part:
The two sorts to compare are the Bubble Sort and the Selection Sort. You are to test your sorts against different sets of strings. Each sort will sort six sets of data, one set has 1000 strings, then a set with 3000 strings, 5000, 7000, 9000 and 11000 strings. You will compare how well each sort did with the different data sizes and show results. I would like to see a plot of the results. A table format showing results will do. Use the clock function shown in the handout for timing. Show answers with at least 4 places to right of decimal point. (Note: you need only one array of 11000 using only part of it for the smaller sort/search tasks.)
Second Part:
The two searches to use for comparisons are the Linear Search and the Binary Search. You will search for 5000 random strings in the array of 11000 strings array only and compute the average number of probes (not time) needed to find a match. The target string will be a randomly selected string from the 11000 strings data set. By selecting a string out of the 11000, you will always find the string in a search. You will select randomly 5000 strings for testing each search algorithm. Output the average number of probes needed for each search algorithm.
Third Part:
How does your two search algorithms compare with the theoretical search values?
You are to generate random strings of 20 characters in each string for your string data sets. Below is code to build one (1) random string.
Hint:
char charset[52]; a b c A B C Z;
string randomstr;
for ( i = 1; i <=20; i++ )
{ randomndx = rand() % 56; // random index into array charset.
randomstr = randomstr + charset[ randomndx ]; //concat a char to the string being built
// you are building a string one char at a time.
// The code above builds only one string.
}
To select a random string form the 11000. How? You will generate a random number between 0 to 10999, an index into the 11000 string array. Then use the selected string to do a Search. You will search for the random string using the Linear Search. Now generate a new random number as an index into the 11000 array again and do a search again. You will do the same action for the Binary Search. Do this for 5000 strings for the Linear Search and 5000 strings for the Binary Search.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
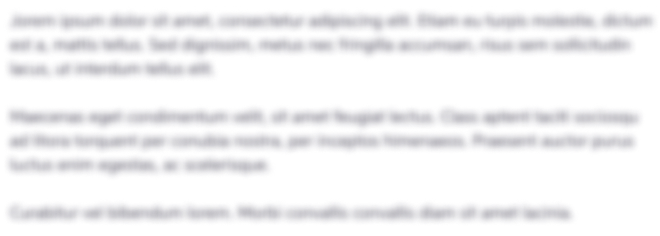
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started