Question
C# You will be implementing a simple class library containing a list of classes. These classes have relationships between them and each of these relationships
C#
You will be implementing a simple class library containing a list of classes. These classes have relationships between them and each of these relationships should be implemented in your code. After creating the class library, you will create a project that uses these classes from this class library.
Your task is to create a class library based on the following UML class diagram.
The attributes of this classes are to be created as properties. Create constructors for each of the classes. The constructor for each class should at minimum accept the name property value of the object, you can add other parameters as you see fit. The constructor for the person class should have the first name, last-name and date of birth for the individual.
Follow these steps to create your class library:
1. Create a new solution
2. Add a new project of type Class Library to your newly created solution.
3. Give your class library project a meaningful name and save it.
4. Add the relevant classes as they appear in the class diagram above, indicating the relationships shown.
5. Build you class library project.
Use the class library you have just created:
1. Add a new project that will be using the class library you have just created to the solution explorer
2. Right-click on the References for the newly created project and add your class library to the references for this project.
Your project should now have access to all the classes in your class library.
To use the classes in the library, add the name space containing the classes in the class library to your code.
We will look at how this classes that have been referenced in your new project will be used
In this new project, create a form that create a school, add departments to the school, add students to the school and display this add students to each department and print out the number of students in each department in the school you have just created
Create a console-based application within the solution in which you have your class library.
1. Using your class library, create an array of 100 Student objects (you can use a random number generator to assign them names e.g. An example student object will have full name as FirstName1 SecondName1 where the numbers are generated using a random number generator. Also assign them a GPA that is between 0 and 4 randomly. Use array segments to increase the GPA of students in the 60th to the 90th index by 0.05 and graduate all students from index 30 through 55. Use your implemented class library based on Lab 4.
Hint: Use the Random class which is in the System namespace to generate your random numbers.
2. Write a program that calls a method with a type Person argument. The method should promote the person if they are a Lecturer, increase their GPA 0.07 by if it is a student object, or print out an error message if the passed argument is neither a student nor a lecture. You may choose to use the nameof or typeof operators.
3. Write a function in C# that accepts two numbers and returns the sum, product, modulus as a tuple of three elements.
4. Using the 100 student objects created in question 2, write a function that stores the first 20 student objects in a single Tuple.
Person +firstName: String +lastName: String +dateOfBirth: DateTime +SSN: String +getFullName0 +getYearOfBirth0 +getAge0 Lecturer Student +program: Program +enrollmentDate: DateTime +currentLevel: EnrollmentLevel +currentGPA: double +graduationDate: DateTime +appointmentDate: DateTime +tenured: bool +lastPromotionDate: DateTime +initialAppointmentRole: String +homeDepartment: Department +currentRole: string +enroll(Program prog) +graduate(Date graduationDate) +promote(String newRole, DateTime promotionDate) +demote(String newRole, DateTime demotionDate) +has Department +lecturersD +name: String +head: Lecturer +address: String +telephoneNumber +programsl +mySchool: School Program +name: String +homeDepartment: Department +minimumDuration: int +relocate(Department newDepartment) +assignHead(Lecturer head) +addLecturer(Lecturer newLecturer) Course enumeration EnrollmentLevel +name: String +code: String +credits: int +description: String Freshman Sophomore Junior Senior School +name: String +departmentsl
Step by Step Solution
There are 3 Steps involved in it
Step: 1
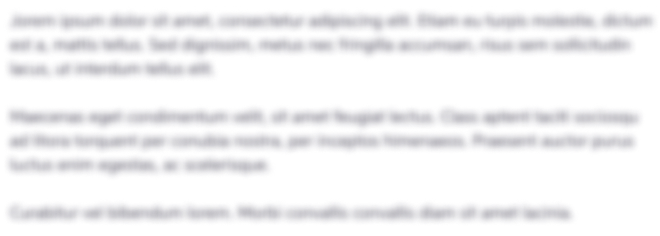
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started