Question
c++ You will start with the Math Break option, which gives the user a slightly fun break from managing their CD collection. For this first
c++
You will start with the Math Break option, which gives the user a slightly fun break from managing their CD collection.
For this first lab, your menu will look like this:
1.Math Break
2.Quit
The Math Break option will have the following sub-menu:
1.Even or Odd
2.Prime Number
3.End my Math Break
Your program will continue to display the Math Break menu until they choose the option to end their Math Break. When they choose to end their Math Break, your program should display the main menu. When they want to quite the whole program they will choose Quit from the menu.
Be sure to use a data validation loop to make sure each option the user enters is a valid option for the menu displayed.
Each Math Break menu option should invoke a function to play the chosen game:
evenOdd
primeNumber
Function evenOdd() does the following:
1.Prompt user for an integer (0 or larger)
2.Validate the user input, checking for negative integers, and not move forward until the user has entered an integer 0 or greater
3.Use a sentinel-controlled while loop to decide if the user wants to quit this game or not use 0 as your sentinel value
4.Call a function, isEven(), that returns a value; true if the number is even and false if the number is odd
5.Display a message telling the user whether the number they entered is even or odd
6.Repeat these steps until the user enters 0, then the Math Break menu is re-displayed
Function primeNumber() does the following:
1.Prompt user for an integer (0 or larger)
2.Validate the user input, checking for negative integers, and not move forward until the user has entered an integer 0 or greater
3.Use a sentinel-controlled while loop to decide if the user wants to quit this game or not use 0 as your sentinel value
4.Call a function, isPrime(), that returns a value; true if the number is prime and false if the number not prime
5.Display a message telling the user whether the number they entered is prime or not
6.Repeat these steps until the user enters 0, then the Math Break menu is re-displayed
Your main() controls the program. Your main() will never just call another function to do all the work.
You will NEVER call main() more than once.
You will NEVER use break, exit, return, or anything to leave a loop, function, or other construct prematurely, unless it is part of the structure as in a case statement.
You will NEVER have a function call itself, unless it is intentional recursion.
You will NEVER use global variables. However, you may use global constants if it is appropriate and they are used properly.
You will have only one return statement in a function.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
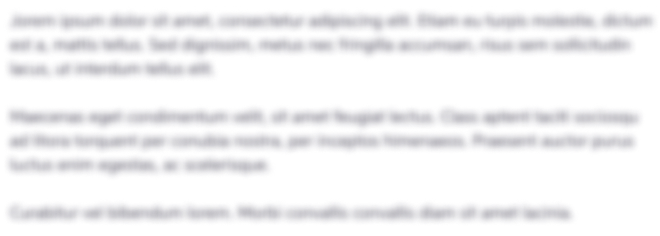
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started