Question
Can anyone help fix my memset function? i dont know what is wrong with it... it should be printing 13 dots. .data # The data
Can anyone help fix my memset function? i dont know what is wrong with it... it should be printing 13 dots.
.data # The data segment.
welc1: .asciiz "Welcome to the test. "
welc2: .asciiz "First, strcpy. This should print 'Hello, world.' twice. "
welc3: .asciiz "Now, strlen. Should be 13: "
welc4: .asciiz "Now, memset. Should print 13 dots: "
welc5: .asciiz "Now, strchr. Should be a number, that +3, then zero. "
welc6: .asciiz "Now, strcat. Should be dots then 'Hello, world.'. "
welc7: .asciiz "Finally, strcmp. Should be 0, then 1, then -1. "
hello: .asciiz "Hello, world."
alice: .asciiz "alice"
bob: .asciiz "bob"
crlf: .asciiz " "
dot: .byte 46
stch: .byte 'l'
buf: .space 128
.text # The code segment.
main:
# --- WELCOME ---
li $v0, 4 # System call to print.
la $a0, welc1 # Load up our message.
syscall # Fire the system call.
# --- strcpy ---
li $v0, 4 # System call to print.
la $a0, welc2 # Load up our message.
syscall # Fire the system call.
li $v0, 4 # System call to print.
la $a0, hello # Load up our message.
syscall # Fire the system call.
li $v0, 4 # System call to print.
la $a0, crlf # Load up our message.
syscall # Fire the system call.
la $a0, buf
la $a1, hello
jal strcpy # strcpy(buf, hello)
move $a0, $v0 # Load up the returned message.
li $v0, 4 # System call to print.
syscall # Fire the system call.
li $v0, 4 # System call to print.
la $a0, crlf # Load up our message.
syscall # Fire the system call.
# --- strlen ---
li $v0, 4 # System call to print.
la $a0, welc3 # Load up our message.
syscall # Fire the system call.
la $a0, buf
jal strlen
move $s0, $v0
move $a0, $v0
li $v0, 1
syscall
li $v0, 4 # System call to print.
la $a0, crlf # Load up our message.
syscall # Fire the system call.
# --- memset ---
li $v0, 4 # System call to print.
la $a0, welc4 # Load up our message.
syscall # Fire the system call.
la $a0, buf
lb $a1, dot
move $a2, $s0
jal memset
move $a0, $v0 # Load up the returned message.
li $v0, 4 # System call to print.
syscall # Fire the system call.
li $v0, 4 # System call to print.
la $a0, crlf # Load up our message.
syscall # Fire the system call.
# --- strchr ---
li $v0, 4 # System call to print.
la $a0, welc5 # Load up our message.
syscall # Fire the system call.
la $a0, hello
li $v0, 1
syscall
li $v0, 4 # System call to print.
la $a0, crlf # Load up our message.
syscall # Fire the system call.
la $a0, hello
lb $a1, stch
jal strchr
move $a0, $v0
li $v0, 1
syscall
li $v0, 4 # System call to print.
la $a0, crlf # Load up our message.
syscall # Fire the system call.
la $a0, buf
lb $a1, stch
jal strchr
move $a0, $v0
li $v0, 1
syscall
li $v0, 4 # System call to print.
la $a0, crlf # Load up our message.
syscall # Fire the system call.
# --- strcat ---
li $v0, 4 # System call to print.
la $a0, welc6 # Load up our message.
syscall # Fire the system call.
la $a0, buf
la $a1, hello
jal strcat
move $a0, $v0 # Load up the returned message.
li $v0, 4 # System call to print.
syscall # Fire the system call.
li $v0, 4 # System call to print.
la $a0, crlf # Load up our message.
syscall # Fire the system call.
# --- strcmp ---
li $v0, 4 # System call to print.
la $a0, welc7 # Load up our message.
syscall # Fire the system call.
la $a0, alice
la $a1, alice
jal strcmp
move $a0, $v0
li $v0, 1
syscall
li $v0, 4 # System call to print.
la $a0, crlf # Load up our message.
syscall # Fire the system call.
la $a0, bob
la $a1, alice
jal strcmp
move $a0, $v0
li $v0, 1
syscall
li $v0, 4 # System call to print.
la $a0, crlf # Load up our message.
syscall # Fire the system call.
la $a0, alice
la $a1, bob
jal strcmp
move $a0, $v0
li $v0, 1
syscall
li $v0, 4 # System call to print.
la $a0, crlf # Load up our message.
syscall # Fire the system call.
# --- finished ---
li $v0, 10 # System call to exit.
syscall # Fire the system call.
strcpy:
# strcpy(dest, src) copies src into dest and returns dest.
# dest = $a0, src = $a1, return $a0 in $v0.
addi $sp, $sp, -4
sw $ra, 0($sp)
move $v0, $a0 # Do this now so we don't have to save $a0
strcpy_next:
lb $t0, 0($a1) # Load a byte
sb $t0, 0($a0) # Save a byte
addi $a0, $a0, 1 # Increment dest
addi $a1, $a1, 1 # Increment src
bne $t0, $zero, strcpy_next # Done?
lw $ra, 0($sp)
addi $sp, $sp, 4
jr $ra
# Copy your code here.
strlen:
li $t0, 0 # initialize the count to zero
loop:
lb $t1, 0($a0) # load the next character into t1
move $v0, $t0
beqz $t1, exit # check for the null character
addi $a0, $a0, 1 # increment the string pointer
addi $t0, $t0, 1 # increment the count
j loop # return to the top of the loop
memset:
lb $t0, 0($a0) #load a0 to t0
sb $zero, ($a0) #store 0 to a0
beqz $t0, exit # check for the null character
addi $a0, $a0, 1 # increment the string pointer
j memset # return to the top of the loop
exit:
jr $ra
Step by Step Solution
There are 3 Steps involved in it
Step: 1
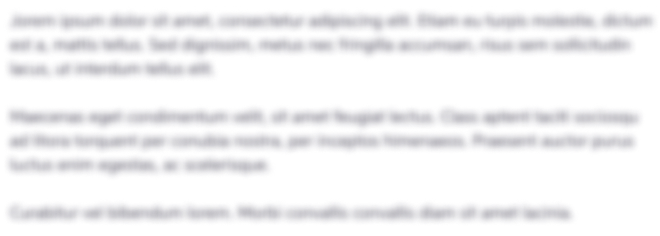
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started