Question
Can anyone help me with this assignment? please Overview: Write a multithreaded C++ program using open MP threads. I will provide a text file containing
Can anyone help me with this assignment? please
Overview: Write a multithreaded C++ program using open MP threads. I will provide a text file containing numbers that must be read into a two dimensional array. The first line of the file will have 2 integers, the number of rows in the array followed by the by the number of columns in the array. The rest of the file will contain integers that the array must be initialized to. Your program must also utilize a random number generator. I will provide source code.
Description: Your program will indicate the cell address (the row and column) of the cell with the highest neighborhood average. If there is a tie, your program must report only a single cell that ties the maximum value. I will post a solution consisting of all cells that tie the maximum value so you can be sure your solution is correct. The neighborhood of a cell is all cells that immediately border the cell, including the cell itself. For each cell, you must compute the average of numbers in the neighborhood.
For example, in the following array: unsigned int M[10000][10000]; the neighborhood of cell M[2][8] consists of the following cells:
M[1][7] M[1][8] M[1][9]
M[2][7] M[2][8] M[2][9]
M[3][7] M[3][8] M[3][9]
Because the array will be large, you will need to use dynamic memory allocation (keyword new) on the heap.
Be careful not to go out of bounds on the array when computing neighborhoods
Your program should take a command line argument indicating the number of threads that will be used. here's the source code :
#include#include #include #include #include #include #include #include
using namespace std; // a class to get more accurate time class stopwatch{ private: double elapsedTime; double startedTime; bool timing; //returns current time in seconds double current_time( ) { timeval tv; gettimeofday(&tv, NULL); double rtn_value = (double) tv.tv_usec; rtn_value /= 1e6; rtn_value += (double) tv.tv_sec; return rtn_value; } public: stopwatch( ): elapsedTime( 0 ), startedTime( 0 ), timing( false ) { } void start( ) { if( !timing ) { timing = true; startedTime = current_time( ); } } void stop( ) { if( timing ) { elapsedTime += current_time( )-startedTime; timing = false; } } void resume( ) { start( ); } void reset( ) { elapsedTime = 0; startedTime = 0; timing = false; } double getTime( ) { return elapsedTime; } }; // function takes an array pointer, and the number of rows and cols in the array, and // allocates and intializes the two dimensional array to a bunch of random numbers void makeRandArray( unsigned int **& data, unsigned int rows, unsigned int cols, unsigned int seed ) { // allocate the array data = new unsigned int*[ rows ]; for( unsigned int i = 0; i < rows; i++ ) { data[i] = new unsigned int[ cols ]; } // seed the number generator // you should change the seed to get different values srand( seed ); // populate the array for( unsigned int i = 0; i < rows; i++ ) for( unsigned int j = 0; j < cols; j++ ) { data[i][j] = rand() % 10000 + 1; // number between 1 and 10000 } } void getDataFromFile( unsigned int **& data, char fileName[], unsigned int &rows, unsigned int &cols ) { ifstream in; in.open( fileName ); if( !in ) { cerr << "error opening file: " << fileName << endl; exit( -1 ); } in >> rows >> cols; data = new unsigned int*[ rows ]; for( unsigned int i = 0; i < rows; i++ ) { data[i] = new unsigned int[ cols ]; } // now read in the data for( unsigned int i = 0; i < rows; i++ ) for( unsigned int j = 0; j < cols; j++ ) { in >> data[i][j]; } } int main( int argc, char* argv[] ) { if( argc < 3 ) { cerr<<" usage: exe [input data file] [num of threads to use] " << endl; cerr<<"or usage: exe rand [num of threads to use] [num rows] [num cols] [seed value]" << endl; } // read in the file unsigned int rows, cols, seed; unsigned int numThreads; unsigned int ** data; // convert numThreads to int { stringstream ss1; ss1 << argv[2]; ss1 >> numThreads; } string fName( argv[1] ); if( fName == "rand" ) { { stringstream ss1; ss1 << argv[3]; ss1 >> rows; } { stringstream ss1; ss1 << argv[4]; ss1 >> cols; } { stringstream ss1; ss1 << argv[5]; ss1 >> seed; } makeRandArray( data, rows, cols, seed ); } else { getDataFromFile( data, argv[1], rows, cols ); } cerr << "data: " << endl; for( unsigned int i = 0; i < rows; i++ ) { for( unsigned int j = 0; j < cols; j++ ) { cerr << "i,j,data " << i << ", " << j << ", "; cerr << data[i][j] << " "; } cerr << endl; } cerr<< endl; // tell omp how many threads to use omp_set_num_threads( numThreads ); #pragma omp parallel { } stopwatch S1; S1.start(); ///////////////////////////////////////////////////////////////////// /////////////////////// YOUR CODE HERE /////////////////////// ///////////////////////////////////////////////////////////////////// S1.stop(); // print out the max value here cerr << "elapsed time: " << S1.getTime( ) << endl; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
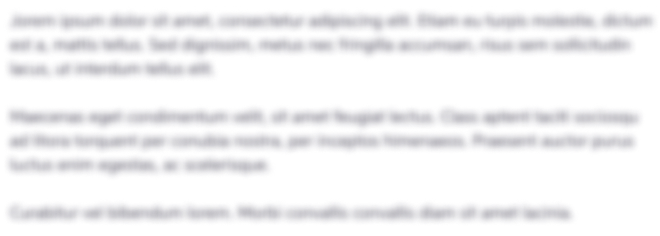
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started