Question
Can anyone help me with this? just Activity 1 2 and anything worth up to 7 points from activity 3. Thank you so much. Here
Can anyone help me with this?
just Activity 1 2 and anything worth up to 7 points from activity 3.
Thank you so much.
Here is my demo.
import java.awt.Image;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Scanner;
import javax.imageio.ImageIO;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JLabel;
public class Demo {
public static void main(String args[]){
Scanner f=null;
//Activity 1
/** This code should compute the factorial of 10 */
//TODO: Debug this code using print statements
int y = 0;
for (int x = 1; x <= 10; x++) {
y *= x;
}
System.out.println("The value of 10! is: "+y);
//Activity 2
/** This code should compute the total wages owed to workers in a cell phone store.
* It reads the hours worked and amount in sales for each employee from a file, then
* calculates total wage as follows:
* - If total hours worked if over 40, pay them $9 for each of the first 40 hours ($360
* total) and $12 for each hour worked past 40 hours. Furthermore, if amount in sales
* is over $300, pay them a bonus of 8% the amount in sales, or if amount in sales is
* between $100 and $300, pay them a bonus of 5% the amount in sales.
* - If total hours worked is under 40, pay them $9 for each hour worked. Furthermore,
* if amount in sales is over $300, pay them a bonus of 10% the amount in sales, or if
* amount in sales is between $100 and $300, pay them a bonus of 5% the amount in sales.
*/
/*
//TODO: Debug this code using the Eclipse debugger
try{
f = new Scanner(new File("data/hours.txt"));
}catch(FileNotFoundException e){
e.printStackTrace();
}
double hourlyWage = 9.00; // paying $9 per hour
double overtimeWage = 12.00; // $12 per hour for overtime work
double wage = 0;
while(f.hasNext()){
String line[] = f.nextLine().split(" "); //split on spaces
String name = line[0]; //employee name
double hours = Double.parseDouble(line[1]);
double sales = Double.parseDouble(line[2]);
if(hours>40){
wage += ( (40 * hourlyWage) + ((hours-40) * overtimeWage) );
if(sales>300){
wage += sales*.08;
}else if(sales>100);
wage += sales*.05;
}else{
wage += hours*hourlyWage;
if(sales>300){
wage += sales*.1;
}else if(sales>100);
wage += sales*.05;
}
System.out.println(name+": $" + wage);
}
*/
//Activity 3 -- choose at least 7 points worth of the following sections to debug and
// describe your debugging process on the worksheet.
/** (A) Fixing this code is worth 2 points.
* This code should find and print the minimum element in the num array.
*/
/*
int[] num = {111, 36, 51, 25, 64, 30, 83, 22, 47, 98};
int min = 0;
for(int i=0; i if(num[i] min = num[i]; } } System.out.println("Minimum element is "+min); */ /** (B) Fixing this code is worth 7 points. * This code should shrink an image by averaging the red, green, and blue values of * each square of pixels into a single pixels in the smaller image. */ /* int[][][] pixels = get3DMatrix("data/Braver.jpg"); //use any image file you want showImage(pixels); //display the original image //declare a matrix with half the height and width to hold the smaller image int[][][] smaller = new int[pixels.length/2][pixels[0].length/2][4]; //use for loops to loop through each 2x2 pixel square for(int i=0; i for(int j=0; j //loop through red, green, and blue components for(int k=0; k<3; k++){ //average the color values from the four pixels in the square and store the result in the smaller matrix smaller[i][j][k] = (pixels[i][j][k]+pixels[i+1][j][k]+pixels[i][j+1][k]+pixels[i+1][j+1][k])/4; } smaller[i][j][3]=255; //set opaqueness to full for each pixel } } showImage(smaller); //display the smaller image */ /** (C) Fixing this code is worth 2 points. * This code reads from a file the number of people at a group activity and outputs * the number of cookies each person gets and how many cookies will be left over. */ /* try { f = new Scanner(new File("data/groups.txt")); } catch (FileNotFoundException e) { e.printStackTrace(); } int numCookies = 36; // number of cookies in a standard package of Oreos while (f.hasNext()) { int numPeople = f.nextInt(); int cookiesPerPerson = numCookies / numPeople; int cookiesLeft = numCookies - numPeople; System.out.println("Each of " + numPeople + " people gets " + cookiesPerPerson + " cookies. There will be " + cookiesLeft + " cookies left."); } */ /** (D) Fixing this code is worth 5 points - you are NOT allowed to change the data * file! You are only allowed to change the code. * This code reads the description of events from a file and prints a reformatted summary. * The file entries are formatted with fields separated by semicolons * example: Free for all 5K;Come run a 5K!;Sunday;March 8;10:00;Outdoor Adventures Center * The output format should be: * name of event: abbreviated_day_of_week abbreviated_month day# at time, location * example: Free for all 5K: Sun Mar 8 at 10:00, Outdoor Adventures Center * If any fields are missing, print a "-" * example: Free for all 5K: - Mar 8 at 10:00, Outdoor Adventures Center */ /* try { f = new Scanner(new File("data/events.txt")); } catch (FileNotFoundException e) { e.printStackTrace(); } ArrayList while(f.hasNext()){ //get an event description from the file String[] event = f.nextLine().split(";"); //split on semicolons String name = event[0]; //event name String dayOfWeek = event[2].substring(0,3); //abbreviate the day of the week String time = event[3]; //event time String location = event[5]; //event location //break down month and day String[] date = event[3].split(" "); //split on spaces String month = date[0].substring(0,3); //abbreviate the month int dayNum = Integer.parseInt(date[1]); //day of the month //add reformatted event to arraylist String e = name+": "+dayOfWeek+" "+month+" "+dayNum+" at "+time+", "+location; events.add(e); } //print all events for(int i=0; i System.out.println(events.get(i)); } */ } /**A method that takes an image file and returns the 3-D pixels integer matrix * @param fileName * @return */ public static int[][][] get3DMatrix(String fileName){ int[][][] pixels = null; try{ /*load image from file*/ BufferedImage image = ImageIO.read(new File(fileName)); /*convert image to an int matrix*/ pixels = new int[image.getWidth()][image.getHeight()][4]; for (int x=0; x for(int y=0; y /*Default RGB color model (TYPE_INT_ARGB), 8-bits of precision for each color*/ int rgb = image.getRGB(x,y); /*Split into components */ pixels[x][y][3] = (rgb >> 24) & 0xFF; // "alpha"/opaqueness (0-255, 255 recommended) pixels[x][y][0] = (rgb >> 16) & 0xFF; // red pixels[x][y][1] = (rgb >> 8) & 0xFF; //green pixels[x][y][2] = (rgb) & 0xFF; //blue } } }catch(Exception e){ System.out.println("error loading the image"); } return pixels; } /**A method to convert the 3D pixel matrix into an image * @param pixels * @return */ private static BufferedImage getImage(int[][][] pixels){ int w = pixels.length; int h = pixels[0].length; BufferedImage image = new BufferedImage(w, h, BufferedImage.TYPE_INT_ARGB); for(int x=0; x for(int y=0; y int rgb = (pixels[x][y][3] << 24); rgb = rgb | ((pixels[x][y][0]) << 16 ); rgb = rgb | (pixels[x][y][1] << 8); rgb = rgb | pixels[x][y][2]; image.setRGB(x,y,rgb); } } return image; } /**A method to display the image described by the 3-D pixel matrix in a JFrame window. */ public static void showImage(int[][][] pixels){ /*convert int matrix to image*/ Image image = getImage(pixels); /*display image in JFrame window*/ JFrame frame = new JFrame("Image"); ImageIcon icon = new ImageIcon(image); JLabel label = new JLabel(icon); frame.setContentPane(label); frame.setVisible(true); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.pack(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
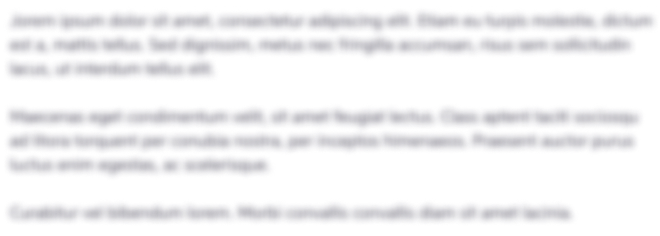
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started