Question
can anyone help me write this program write a java program to preform twos complement binary addition and subbstraction with 8 bit signed binary numbers.
can anyone help me write this program "write a java program to preform twos complement binary addition and subbstraction with 8 bit signed binary numbers. you are to write a menu driven program that gives the user three choices, 1) add 2) substract 3) quit. the input to your program will be two signed binary numbers whichh are to be read in as strings. You must write java methods with headers, public static String addBin(String x, String y) and public static String subBin(String x, String y) for addition and substraction. the arithmitic must be preformed in binary and the program should display the input/output numbers in binary as well as in decimal." I have pasted below a program whith methhods that must be used for this program
package lab3;
//Wissam Hammoud
//COSC 221
//Section 02
//lab 1
//Winter 2018
//This program gives the user three choices.
//They can either convert a decimal to a binary, a binary to a decimal, or exit from the program.
//This program has a method to convert to binary, a method to convert to decimal,
//and the main method.
import java.util.Scanner;
public class lab3 {
public static void main(String[] args) {
int choice;
String bin1, bin2;
Scanner sc = new Scanner(System.in);
System.out.println("enter bin1");
bin1 = sc.nextLine();
System.out.println("enter bin2");
bin2 = sc.nextLine();
while (true) {
System.out.println("~~~Select any one from below~~~ " // displays menu
+ "1. ADD " + "2. SUBSTRACT " + "3. QUIT ");
choice = sc.nextInt(); // stores the users input as a choice
if (choice == 1) {
System.out.println(addBin(bin1, bin2));
} else if (choice == 2) {
System.out.println(" SUBSTRACTING " + bin1 + " and " + bin2);
} else {
System.exit(0);
System.out.println(" END OF PROGRAM"); // program ends
break;
}
}
}
public static String addBin(String X, String Y) {
}
public static String subBin(String x, String y) {
}
public static String decToBin(int x) {
String bin = ""; // store the bin string
int rem;
int sign = 1;
if (x < 0) // check if x is negative
{
sign = -1; // change sign
x = -x; // make x positive
}
for (int i = 0; i < 8; i++) { // runs until you get the 8 bit
rem = x % 2; // rem is remainer of the dec / 2
x = x / 2; // x is the dec divided by 2
bin = rem + bin; // the binary number is the remainder and its stored in bin
}
/// if negative
if (sign == -1) {
String negBin = ""; // new string
// 1's complement
for (int i = 0; i < bin.length(); i++) {
if (bin.charAt(i) == '0')
negBin = negBin + '1';
else
negBin = negBin + '0';
}
// adding 1
rem = 1;
bin = "";
for (int i = negBin.length() - 1; i >= 0; i--) {
if (rem == 1) {
if (negBin.charAt(i) == '1') {
bin = '0' + bin;
} else {
bin = '1' + bin;
rem = 0;
}
} else {
bin = negBin.charAt(i) + bin;
}
}
}
return bin;
}
public static int binToDec(String s) {
int num = 0;
int sign = 1; // initialize sign
// if negative number
if (s.charAt(0) == '1') {
sign = -1; // change sign
String s1 = ""; // new string
// 1's complement
for (int i = 0; i < s.length(); i++) {
if (s.charAt(i) == '0')
s1 = s1 + '1';
else
s1 = s1 + '0';
}
// Adding 1
int rem = 1;
s = "";
for (int i = s1.length() - 1; i >= 0; i--) {
if (rem == 1) {
if (s1.charAt(i) == '1') {
s = '0' + s;
} else {
s = '1' + s;
rem = 0;
}
} else {
s = s1.charAt(i) + s;
}
}
}
for (int i = 7; i >= 0; i--) // i is the power
if (s.charAt(i) == '1') // if 1 appears, it takes 2^(7-i)
num = num + (int) Math.pow(2, 7 - i);
return sign * num;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
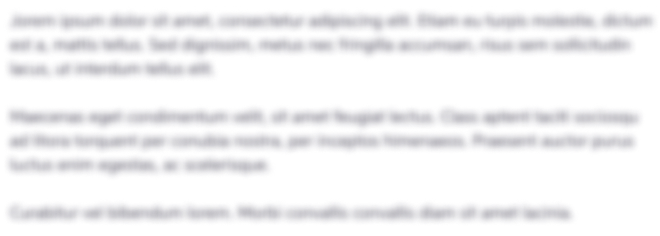
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started