Question
Can anyone try to help me write this java code in these two exercises? If someone could help that would be great! Thanks! In the
Can anyone try to help me write this java code in these two exercises? If someone could help that would be great! Thanks!
In the following exercise you are not premitted to "plink" values into the matrix with solitary assignment statements. You must always use a loop to initialize a row, col or diagonal in the matrix.
Exercise1.java
Do not change main
Just fill in these methods below main
zeros()
diagonal_1()
diagonal_2()
border()
Don't change any other code or write any other methods
The output of your program should look like this:
DIAGONAL_1 0 0 0 0 4 0 0 0 3 0 0 0 2 0 0 0 1 0 0 0 0 0 0 0 0 ZEROS 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 DIAGONAL_2 0 0 0 0 0 0 1 0 0 0 0 0 2 0 0 0 0 0 3 0 0 0 0 0 4 ZEROS 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 BORDER 0 1 2 3 4 1 0 0 0 3 2 0 0 0 2 3 0 0 0 1 4 3 2 1 0 |
In the following exercise you will implement Matrix Addition
Exercise2.java
Do not change main
Just fill in this method below main
addMatricies()
Don't change any other code or write any other methods
The output of your program should look like this:
MATRIX1 1 2 3 2 4 6 MATRIX2 4 6 8 6 9 12 MATRIX1 + MATRIX2 5 8 11 8 13 18 |
Files:
Exercise1.java:
/* Exercise1.java */ import java.io.*; import java.util.*; public class Exercise1 { public static void main( String[] args ) { try // We will learn about Exceptions soon { int[][]m = new int[5][5]; /* JAVA automatically initializes all the elements to 0. If printed will look like this: 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 */ fillDiagonal_1( m ); // You fill in code for method fillDiagonal_1 (below main) printMatrix( "DIAGONAL_1",m ); /* After fillDiagonal_1() the print of the matrix must look like this: 0 0 0 0 4 0 0 0 3 0 0 0 2 0 0 0 1 0 0 0 0 0 0 0 0 */ zeroMatrix( m ); // You fill in code for method zeroMatrix (below main) printMatrix( "ZEROS",m ); // should come out as all zeros fillDiagonal_2( m ); // You fill in code for method fillDiagonal_2 (below main) printMatrix( "DIAGONAL_2",m ); /* After fillDiagonal_2() the print of the matrix must look like this: 0 0 0 0 0 0 1 0 0 0 0 0 2 0 0 0 0 0 3 0 0 0 0 0 4 */ zeroMatrix( m ); // You fill in code for method zeroMatrix (below main) printMatrix( "ZEROS",m ); // should come out all zeros again fillBorder( m ); // You fill in code for method fillBorder (below main) printMatrix( "BORDER",m ); /* After fillBorder() the print of the matrix must look like this: 0 1 2 3 4 1 0 0 0 3 2 0 0 0 2 3 0 0 0 1 4 3 2 1 0 */ } catch( Exception e ) { System.out.println("EXCEPTION CAUGHT: " + e ); System.exit(0); } } // END MAIN // - - - - - - - - - - Y O U W R I T E T H E S E F O U R M E T H O D S ----------- // You must use only one for loop // You are NOT allowed to use a hardcoded literal 4 or 5 for row col length // You must always use expressions like matrix.length or matrix.length-1 // or matrix[row].length -1 etc. // Do not use a literal number except the number "1" as in length-1 etc private static void fillDiagonal_1( int[][] matrix ) { // YOUR CODE HERE } // You must use only one for loop // You are NOT allowed to use a hardcoded literal 4 or 5 for row col length etc // You must always use expressions like matrix.length or matrix[row].length etc. private static void fillDiagonal_2( int[][] matrix ) { // YOUR CODE HERE } // You may use as many as 4 (four) for loops // You are NOT allowed to use a hardcoded literal 4 or 5 for row col length etc // You must always use expressions like matrix.length or matrix[row].length etc. private static void fillBorder( int[][] matrix ) { // YOUR CODE HERE } // Use a nested for loop (for loop in a for loop) to set every elemnt to zero // HINT: Look at the code in printMatrix - it's identical to what you need here except // instead of printing matrix[row][col], you assign it a 0. You may use literal 0 of course here. private static void zeroMatrix( int[][] matrix ) { // YOUR CODE HERE } // - - - - - - - - - - D O N O T M O D I F Y. U S E A S I S ---------------- // IMPORTANT: matrix.length produces the number of rows. The num of rows IS the length of matrix // IMPORTANT: matrix[i].length produces the number of columns in the i'th row private static void printMatrix( String label, int[][] matrix ) { System.out.println(label); for (int row=0 ; rowExercise2.java:
/* Exercise2.java */ import java.io.*; import java.util.*; public class Exercise2 { public static void main( String[] args ) { try // We wil learn about Exceptions soon { int[][]m1 = new int[2][3]; int[][]m2 = new int[2][3]; int[][]m3 = new int[2][3]; // put some numbers in m1 for (int r=0; r
Step by Step Solution
There are 3 Steps involved in it
Step: 1
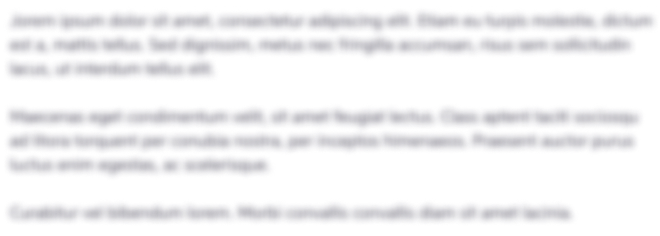
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started