Question
Can someobody help me this project? Please see the bottom for codes that is already given. This assignment asks you to write a Python interpreter
Can someobody help me this project? Please see the bottom for codes that is already given.
This assignment asks you to write a Python interpreter for a "Little" language, Though we've learned about four phases in building a compiler: lexical analysis, building parse trees, semantic analysis (code generation) and optimization, you will not need to do all of that for this small language.
You will also need to turn in a short write up explaining why your program is much easier than a true compiler. This must be in paragraph form and include at least 3 things that you didn't need to implement that a compiler does need to do.
The Little language is defined by the following BNF:
digit ::= 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 int ::=
This means that the language only supports positive integers and only has 8 operations: double, triple, square, cube, add, sub, mult and div. In addition, this language only allows one expression per line, doesn't have variables, and doesn't allow for "chaining" expressions.
The 8 operations perform the following:
double: Takes a single integer operand and prints the double of it (i.e. multiply by 2)
triple: Takes a single integer operand and prints the triple of it (i.e. multiply by 3)
square: Takes a single integer operand and prints the square (i.e. the operand times itself)
cube: Takes a single integer operand and prints the cube (i.e. raised to the 3rd power)
add: Takes two or more operands. The printed result is all the operands added together.
sub: Takes two operands. The printed result is the second operand subtracted from the first operand
mult: Takes two or more operands. The printed result is all the operands multiplied together
div: Takes two operands. The printed result is the first operand divided by the second operand
Your program must:
Your program must prompt for an input file name
Your program must process each line of the file, and produce correct output for each line. This output can simply be written to the Python Shell window.
The processing for each line is as follows:
Lines containing only white space or beginning with a comment (defined as a # in Little) are ignored
For other lines, the line (minus the newline character) must be printed, followed by the value produced by executing the line. Note: the value produced could be an error message.
Run your program on the attached TestAll.little file.
You may define and use your own functions, but it is not required.
I have included an outline of the program for you to start from.
Start with the included outline, run it, and continue fixing it/adding functionality until you have everything working.
The outline provided includes some functions for you to use. You will need to make modifications to some of the provided functions. This may mean additional code or changes to existing code - the functions which need modification have # TODO comments for you to find and modify/fix. Make sure you look at what the function is returning and make sure it makes sense with your changes.
The main body of the program also contains a # TODO comment reminding you to implement the operations that I didn't.
I suggest you proceed in an incremental fashion - work on one thing and get it working, then proceed to the next thing - this is called iterative development. DO NOT TRY TO WRITE THE ENTIRE PROGRAM AND THEN HOPE IT RUNS!!! Make SURE you are testing what you have periodically. It is MUCH easier to debug what might be wrong if you've only written 2 or 3 lines since the last time you tested the code. You may also want to save working copies periodically so you have something working to go back to in case you make a mistake you can't figure out.
Also - Remember that you can use the # to comment out lines in your TestAll.little file. This may be helpful if you start to get errors while you are working on something else. Just remember to remove the # comments later and make sure everything is working.
You might want to refer to the Python Chapter and the Python slides (specifically Python Strings, Python6 Working with Files and Python7 Functions) to help you.
Be sure to run your program on the provided TestAll.little. The correct output is shown below. There are 56 output lines. To receive full credit, your completed program should produce the same results.
Grading Breakdown:
GENERAL (10 points)
You must turn in a Python program as a .py file to receive credit.
1 point for a comment which including your name
3 points for comments for new functionality and removing unneeded TODOs
3 points for turning in example output. You only need to run the program once.
3 points for appropriate use of loops
MAIN PROGRAM (40 points)
2 points for prompting for the filename (and using the one the user enters)
2 points for properly opening the file (given)
2 points for properly closing the file (given)
3 points for skipping lines starting with a comment
4 points for implementing triple
4 points for implementing square
4 points for implementing cube
6 points for implementing sub
6 points for implementing div
6 points for implementing mult
1 point for not breaking double and add (given)
FUNCTIONS (5 points)
5 points for correctly fixing the isBinaryOp and isListOp functions
ERRORS (15 points)
15 points for correctly checking for errors
- 2 points for correct number of operands for unary operations
- 2 points for correct number of operands for binary operations (given)
- 2 points for correct number of operands for list operations
- 2 points for checking whether the operation is valid (given)
- 2 points for checking whether there are any operands
- 3 points for checking whether there is a divide by zero error
- 2 points for making sure operands are numeric (given)
OUTPUT (20 points)
1 point for printing a reasonable error message when an operation doesn't exit
(For example: Parsing error: no such operation)
This should be printed 5 times
1 point for printing a reasonable error message when multiple operands are expected
(For example: Parsing error: multiple operands expected)
This should be printed twice
1 point for printing a reasonable error message when there is a binary operator without exactly two operands
(For example: Parsing error: binary operator requires two operands)
This should be printed twice
1 point for printing a reasonable error message when there is a unary operator with more than 1 operand
(For example: Parsing error: unary operator requires one operand)
This should be printed three times
1 point for printing a reasonable error message when there is a correct operation, but no operands
(For example: Parsing error: no operands)
This should be printed three times
1 point for printing a reasonable error message when there are the correct number of operands, but one or more of them are not integers (given)
(For example: Parsing error: integer expected)
This should be printed 4 times
1 point for printing a reasonable error message when there is an attempt to divide by zero
(For example: Divide by zero error)
This should be printed once
4 points for the math being correct when printing double, triple, square and cube operations
2 points for the math being correct when printing sub and div operations
2 points for the math being correct when printing add operations
2 points for the math being correct when printing mult operations
1 point for not printing anything for blank lines (given)
2 points for not printing anything for lines that start with comment
WRITEUP (10 points)
Your write up in paragraph form explaining why your program is easier to write than a true compiler. Put another way, what sorts of things does a compiler worry about that you didn't need to? You should include at least 3 things that you didn't need to implement that a compiler does.
----------------------------------------------------------------------
Test All Little
# Test file for little language
double 5 double 10
triple 7 triple 2
square 2 square 5
cube 3 cube 5
sub 5 4 sub 8 4
add 2 3 add 1 2 3 add 1 2 3 4 5 add 2 3 3 add 4 5 6 7 8 9 10 2 3 4 add 4 2 3 2 4 4 5 div 10 5 div 8 2 div 16 4
mult 4 5 mult 1 2 3 mult 1 2 3 4 5 mult 2 3 4 5 mult 6 7 5 3 2 1 2 3 4 5
# Mix them up square 3 double 6 mult 5 2 sub 9 3 add 3 4 2 sub 12 2 cube 8 add 5 6 mult 8 2 1 2 triple 4
# Test spaces square 7 add 3 2
# The following cases should produce errors
error null no_op add 4 double 3 8 sub 2 sub 3 4 5 sub mult 3 hi triple cube cube 6 7 halve 8 square 4 5 double trouble add hello world add 4 5 xyz sub 3 abc div 3 0
----------------------------------------------------------------------
# This program interprets lines in a .little file and computes them. # The little language is defined by the following BNF: # # digit ::= 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 # int ::=
# Returns true if the operation only takes one operand def isUnaryOp (op): if op == "double" or op == "triple" or op == "square" or op == "cube": return True
# Returns true if the operation takes exactly two operands def isBinaryOp (op): # TODO return False
# Returns true if the operation takes 2 or more operands def isListOp (op): # TODO return False
# Returns true if the operation is valid def isValidOp (op): return isUnaryOp(op) or isBinaryOp(op) or isListOp(op)
# Returns true if the string passed in is an integer def isValidInt (num): try: int(num) return True except ValueError: return False
# Returns True if there is an error found in the expression def found_errors (op, numOperands, expression, line):
# if the operation is not valid if (not isValidOp (op)): print line + ":\tParsing error: no such operation" return True # if there are no operands # TODO # if operator is unary, then there should be one operand # TODO # if operator is binary, then there should be two operands if (isBinaryOp (op) and not numOperands == 2): print line + ":\tParsing error: binary operator requires two operands" return True
# if operator is a list op, then there should be more than one operand # TODO # Can't divide by zero # TODO # Check to make sure all the operands are numeric: i = 1 while i
######################################## ### main program starts here ### ########################################
# Ask the user for the name of the file: # TODO
filename = "TestAll.little"
# Open the file: print "Reading file: " + filename infile = open (filename, "r")
# Loop through each line in the file and process it: for i in infile:
# Get rid of leading and trailing spaces, including the newline character line = i.strip()
# Split line into a list expression = line.split()
# Number of operands = the number of elements in the line -1 (the operand) numOperands = len (expression) - 1 # check for whitespace or comments # If the line is a comment or is blank, we can skip it. # We could do this with an if...else block and indent all the rest of the code. # But in this case, continue will make the rest of the code easier to read. # That's not always the case with break and continue - which is why we need # to be careful when we choose to use them
# This line checks for empty lines if numOperands == -1: continue
# Check for comments - lines which start with a hashtag # TODO - For help, See Python Strings in Week 4
# find operator # Operator is the first element in the line. op = expression[0] error_found = found_errors (op, numOperands, expression, line) if not error_found:
# double operation has one operand and prints the value of the operand multiplied by 2 if (op == "double"): print line + ":\t", int(expression[1]) * 2
# add operation has many operands and prints the total of all of them if (op == "add"): total = 0 i = 1 while (i
# TODO: Implement triple, square, cube, sub, div and mult infile.close()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
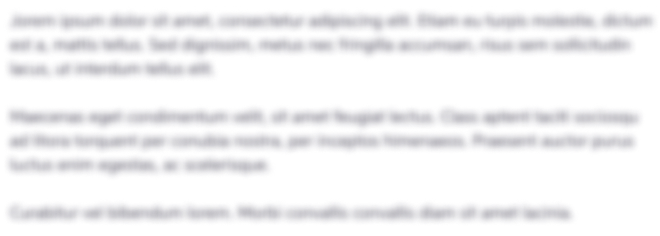
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started