Question
Can someone check my code and help me fix or add things? # import all necessary modules def match_chars(s0, s1): Returns the number of times
Can someone check my code and help me fix or add things?
# import all necessary modules
def match_chars(s0, s1):
"""Returns the number of times s0 and s1 contain the same character in
the same index
Args:
s0: a string
s1: a string with the same length as s1
Return:
Count of same character at same index
"""
length = min(len(s0), len(s1))
count = 0
for i in range(length):
if (s0[i] == s1[i]):
count += 1
return count
def create_pattern1(ch, num):
""" Returns the string that stores following pattern with given
character ch
for example:
for create_pattern('*', 4) will return string that contains:
*
**
***
****
Args:
ch: character to be printed in pattern
num: number of times it should be printed
Return: string with pattern
Hint: Use for loop and range function
"""
ls = []
for i in range(num):
ls.append(ch * (i + 1))
string = ' '.join([str(elem) for elem in ls])
return string
def create_pattern2(ch, num):
""" Same as create_pattern1.
Complete this function using while loop
"""
ls = []
i = 0
while i < num:
ls.append(ch * (i + 1))
i += 1
string = ' '.join([str(elem) for elem in ls])
return string
def is_balanced(dictionary):
""" Return True if and only if values in a dictionary add to 1.
For instance, output of is_balanced({'R': 0.5, 'G': 0.4, 'B': 0.7})
should be False
output of is_balanced({'R': 0.3, 'G': 0.5, 'B': 0.2})
should be True
Args:
dictionary
Return:
True or False
"""
summ = 0
for i, j in dictionary.items():
summ += j
if (1.0 == summ):
return True
else:
return False
def count_duplicates(dictionary):
""" Return the number of duplicate values in dictionary.
for instance,
count_duplicates({'Red': 1, 'Green': 2, 'Blue': 2, 'Yellow': 1,
'Purple': 3})
the return of this function should be 2
Args:
dictionary
Return: duplicates
"""
# initialize variable that stores number of duplicates
# convert dictionary values into a list using function list()
# store this list in a new variable
# wtite a for loop on the list of values
# if an item appears at least 2 times, it is a duplicate
# use .count() function to count items in list
# increment variable duplicate by 1
# Work is not done yet!!
# You also need to be sure that item has been removed from
# the list to avoid counting it again
# To do this, first create a variable to store number of occurences
# create a for loop to loop over for i in range(num_occurrences)
# and remove it from the list using .remove()
# return duplicates
dict_list = list(dictionary.values())
duplicate_count = 0
for ele in dict_list:
count_ele = dict_list.count(ele)
if count_ele > 1:
for i in range(count_ele - 1):
dict_list.remove(ele)
duplicate_count += 1
return duplicate_count
def backup_file():
"""Makes a backup of a file. Your program should
prompt the user for the name of the file to copy and then write a new file
with the same contents but with .bak as the file extension
Args: None
Return: None
This should create another file in your folder
You can use alkaline.txt to create backup alkaline.txt.bak
"""
# take input from the user asking which file would they like to backup?
# store the name of file in new variable, filename
# create new filename with .bak extension by adding filename + '.bak'
# Open new filename in 'w' mode
# loop over file you would like to backup
# use .write() to write each line in old file into new backup file
# close the file
pass
def reverse_file(f):
"""Returns a reverse of the file in a new list called reverse_list.
Args:
f: a freshly opened file in the format of the file alkaline.txt
Return:
list in reverse order
Hint:
Use split() to create a list of each line
Create another list to store the list in reverse order.
Traverse the first list using for loop from end of this list
Append each list item into new list
"""
# create empty list
# Use for loop and .split() to append each line of .txt file into
# the list
# create another empty list to store reverse of this list
# create variables to store the variables such as length of
# your list using function len()
# traverse the list using for loop in reverse order using length
# of the listappend each element of the list to new list.
# Decrement variable
# at the end, return the list
my_list = []
reverse_list = []
file = open(alkaline.txt)
content = file.read()
my_list = content.split(' ')
length = len(my_list)
for i in range(length-1, -1, -1):
reverse_list.append(my_list[i])
file.close()
return reverse_list
if __name__ == '__main__':
# function calls/sanity checks
# Sanity check for match_char
s0 = 'Golden'
s1 = 'Silver'
count = match_chars(s0, s1)
print('the number of times the two strings contain the same character in '
'the same index is', count)
s0 = 'aaccggggtgtttta'
s1 = 'ccaattttgaaaaaa'
count = match_chars(s0, s1)
print('the number of times the two strings contain the same character in '
'the same index is', count)
print('-----------------------------------------------------------')
# Sanity check for creating patterns
print(create_pattern1('*', 4))
print(create_pattern2('+', 5))
print('-----------------------------------------------------------')
# profile your functions create_pattern1 and create_pattern2
# for large number of characters
# write code to time your function create_pattern1('*', 1000)
print('The code took {:.2f}ms'.format((t2 - t1) * 1000.))
# write code to time your function create_pattern2('*', 1000)
print('The code took {:.2f}ms'.format((t2 - t1) * 1000.))
print('-----------------------------------------------------------')
# Sanity check for is_balanced
dictionary = {'R': 0.3, 'G': 0.5, 'B': 0.2}
print('The dictionary is balanced: ', is_balanced(dictionary))
print('-----------------------------------------------------------')
# Sanity check for counting duplicate values in a dictionary
dictionary = {'Red': 1, 'Green': 2, 'Blue': 2, 'Yellow': 1, 'Purple': 3}
print('counting duplicates: ', count_duplicates(dictionary))
dictionary = {'R': 0.3, 'G': 0.5, 'B': 0.2}
print('counting duplicates: ', count_duplicates(dictionary))
print('-----------------------------------------------------------')
# Working with files
filename = 'alkaline.txt'
# Sanity check for backup_file()
# should create a file with extension .bak in same folder
backup_file()
print('-----------------------------------------------------------')
# Sanity check for reverse_file()
with open(filename) as f:
print('File in reverse order is: ', reverse_file(f))
print('-----------------------------------------------------------')
Step by Step Solution
There are 3 Steps involved in it
Step: 1
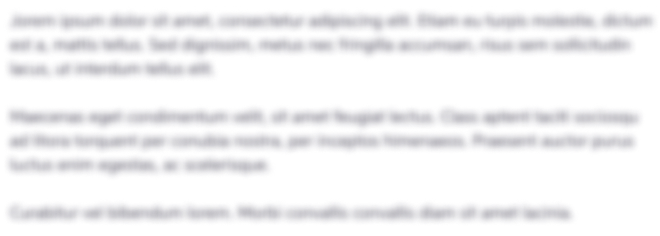
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started