Question
Can Someone check my code for my CheckoutLanes class: What I am supposed to do is Create a CheckoutLanes class that has some express lines
Can Someone check my code for my CheckoutLanes class:
What I am supposed to do is Create a CheckoutLanes class that has some express lines and regular lines. The class should look something like:
public class CheckoutLanes implements ICheckoutLanes { ... public CheckoutLanes(int numExpress, int numRegular) { ... } public void enterLane(int laneNumber, Shopper shopper) { ... } public List<Shopper> simulateCheckout() { ... } ... }
The constructor should create a CircularArrayQueuess for each express and regular lane. Store the queues in one or two arrays. You can use two arrays.
There must be at least one regular lane.
The void enterLane(int laneNumber, Shopper shopper) method adds the shopper to the given checkout lane. Express lanes come before regular lanes. If you had 2 express lanes and 4 regular lanes, lanes 0 and 1 are express and 2 - 5 are regular. This method does not check the shoppers number of items.
The List simulateCheckout() method should loop until all checkout lanes are empty.
Each time through the outer loop, the method should process the shopper at the head of the lane. The simulation should start at lane 0 and go to lane N - 1 processing one shopper at a time.
For express lanes, if the shopper has more than 10 items they are placed at the end of a regular lane. Print out Express lane shopper with items moved to lane . Else the method should print out Express Lane , shopper had items indicating the shopper has checked out.
For regular lanes, the method should print out Regular Lane , shopper had items indicating that the shopper has checked out.
When the shopper checks out they are added to the List of shoppers that simulateCheckout returns.
Here is the ICheckOutLane interface
import java.util.List; public interface ICheckoutLanes { /** * Adds the shopper to the checkout lane. * * @param laneNumber the checkout lane number. * @param shopper the shopper. */ void enterLane(int laneNumber, Shopper shopper); /** * Simulates the checkout process. * * @return A list of shoppers in the order they are finished. */ List simulateCheckout(); }
Code to be checked:
import java.util.ArrayList;
import java.util.List;
public class CheckoutLanes implements ICheckoutLanes { private CircularArrayQueue expressLanes; private CircularArrayQueue regularLanes; private CircularArrayQueue[] lines; private int numExpressLanes; private int numRegularLanes;
public CheckoutLanes(int numExpress, int numRegular) { if (numRegular <= 0) { throw new IllegalStateException(); } for (int i = 0; i < numExpress; i++) { expressLanes = new CircularArrayQueue(); } for (int i = 0; i < numRegular; i++) { regularLanes = new CircularArrayQueue(); } lines = new CircularArrayQueue[numRegular + numExpress]; if (numExpress != 0) { for (int i = 0; i < numExpress; i++) { lines[i] = expressLanes; } } for (int i = numExpress; i < numRegular; i++) { lines[i] = regularLanes; } numExpressLanes = numExpress; numRegularLanes = numRegular; }
public void enterLane(int laneNumber, Shopper shopper) { if (numExpressLanes != 0) { for (int i = 0; i < numExpressLanes; i++) { expressLanes.add(shopper); } } for (int i = numExpressLanes; i < numRegularLanes; i++) { regularLanes.add(shopper); } }
public List simulateCheckout() { List checkOut = new ArrayList(); int lengthOfExpressLane = 0; for (int i = 0; i < lines.length; i++) { //iterate for the array of queues for (int j = 0; j < numExpressLanes; j++) { //iterate over the express lanes Shopper customer = expressLanes.element(); if (customer.getNumberOfItems() > 10) { //possibly could use enter lane method regularLanes.add(customer); System.out.println("Express lane shopper with " + customer.getNumberOfItems() + " items moved to lane " + lines.length); expressLanes.remove(); } else { System.out.println("Express Lane " + j + " shopper had " + customer.getNumberOfItems() + " items"); checkOut.add(customer); expressLanes.remove(); } lengthOfExpressLane++; } for (int e = lengthOfExpressLane; e < lines.length; e++) { //iterate over regular lanes Shopper customer = regularLanes.element(); System.out.println("Regular Lane " + e + " shopper had " + customer.getNumberOfItems() + " items"); checkOut.add(customer); regularLanes.remove(); } } return checkOut; }
public static void main(String[] args) {
}
}
Here is my CircularArrayQueue class
import java.util.NoSuchElementException;
public class CircularArrayQueue implements Queue211 {
private int front;
private int rear;
private int size;
private int capacity;
private static final int DEFAULT_CAPACITY = 10;
private E[] data;
public CircularArrayQueue() {
@SuppressWarnings("unchecked")
public CircularArrayQueue(int defaultCapacity) {
capacity = defaultCapacity;
data = (E[]) new Object[capacity];
front = 0;
rear = capacity - 1;
size = 0;
}
@Override
public boolean add(E e) {
if (size == capacity) {
reallocate();
}
size++;
rear = (rear + 1) % capacity;
data[rear] = e;
return true;
}
@SuppressWarnings("unchecked")
private void reallocate() {
int newCapacity = 2 * capacity;
E[] newData = (E[]) new Object[newCapacity];
int j = front;
for (int i = 0; i < size; i++) {
newData[i] = data[j];
j = (j + 1) % capacity;
}
front = 0;
rear = size - 1;
capacity = newCapacity;
data = newData;
}
@Override
public E element() {
if (size == 0) {
throw new NoSuchElementException();
}
return data[front];
}
@Override
public boolean offer(E e) {
try {
return add(e);
} catch (IllegalStateException ise) {
return false;
}
}
@Override
public E peek() {
try {
return element();
} catch (NoSuchElementException nse) {
return null;
}
}
@Override
public E poll() {
try {
return remove();
} catch (NoSuchElementException nse) {
return null;
}
}
@Override
public E remove() {
if (size == 0) {
throw new NoSuchElementException();
}
E result = data[front];
front = (front + 1) % capacity;
size--;
return result;
}
@Override
public int size() {
return size;
}
}
Here is the Shopper class
public class Shopper { private static int count = 0; private int numberOfItems; private int shopperNumber; public Shopper(int numItems) { this.shopperNumber = count++; this.numberOfItems = numItems; } public final int getNumberOfItems() { return numberOfItems; } public final int getShopperNumber() { return shopperNumber; } }
test file for checkoutLanes
import static org.junit.Assert.assertEquals; import static org.junit.Assert.assertTrue; import java.util.List; import org.junit.Test; public class CheckoutLanesTest { /** * Test no express lane. */ @Test public void testNoExpress() { System.out.println(" Test No Express"); CheckoutLanes lanes = new CheckoutLanes(0, 1); lanes.enterLane(0, new Shopper(5)); lanes.enterLane(0, new Shopper(10)); lanes.enterLane(0, new Shopper(15)); List shoppers = lanes.simulateCheckout(); assertTrue(shoppers.get(0).getNumberOfItems() == 5); assertTrue(shoppers.get(1).getNumberOfItems() == 10); assertTrue(shoppers.get(2).getNumberOfItems() == 15); } /** * Test one express lane. */ @Test public void testOneExpress() { System.out.println(" Test One Express"); CheckoutLanes lanes = new CheckoutLanes(1, 1); lanes.enterLane(0, new Shopper(15)); lanes.enterLane(0, new Shopper(10)); lanes.enterLane(0, new Shopper(5)); lanes.enterLane(1, new Shopper(25)); List shoppers = lanes.simulateCheckout(); assertEquals("Wrong number of shoppers", 4, shoppers.size()); assertTrue(shoppers.get(0).getNumberOfItems() == 25); assertTrue(shoppers.get(1).getNumberOfItems() == 10); assertTrue(shoppers.get(2).getNumberOfItems() == 15); assertTrue(shoppers.get(3).getNumberOfItems() == 5); } /** * One express two regular. */ @Test public void testOneTwo() { System.out.println(" Test One Express, Two Regular"); CheckoutLanes checkout = new CheckoutLanes(1, 2); checkout.enterLane(0, new Shopper(15)); checkout.enterLane(0, new Shopper(3)); checkout.enterLane(1, new Shopper(20)); checkout.enterLane(2, new Shopper(17)); List shoppers = checkout.simulateCheckout(); assertEquals("Wrong number of shoppers", 4, shoppers.size()); assertTrue(shoppers.get(0).getNumberOfItems() == 20); assertTrue(shoppers.get(1).getNumberOfItems() == 17); assertTrue(shoppers.get(2).getNumberOfItems() == 3); assertTrue(shoppers.get(3).getNumberOfItems() == 15); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
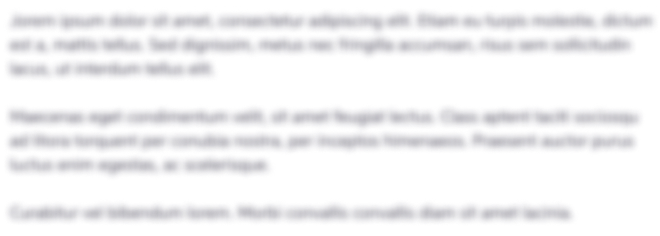
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started