Question
Can someone help me correct this code? It has many errors. Please only answer if you can provide the answer. import java.util.Scanner; public class ProgProject{
Can someone help me correct this code? It has many errors.
Please only answer if you can provide the answer.
import java.util.Scanner; public class ProgProject{ static Scanner read = new Scanner(System.in); //main method public static void main(String[]args){ //declaration and initialization int select; char exit='\0'; do{ //display main menu System.out.println(); System.out.println("Welcome to The Burgers"); System.out.println("-----------------MENU-----------------"); System.out.println("| 1. About us |"); System.out.println("| 2. Food Menu |"); System.out.println("| 3. Add Food Order |"); System.out.println("| 4. Delete Record |"); System.out.println("| 5. Search |"); System.out.println("| 0. EXIT |"); System.out.println("--------------------------------------"); //ask user to input System.out.print("Enter your choice (1-5) or 0 to Exit: "); select = read.nextInt(); switch(select){ case 1 : showAboutUs();break;//method call showAboutUs() case 2 : showFoodMenu();break;//method call showFoodMenu() case 3 : showFoodOrder();break;//method call showFoodOrder() case 4 : showDailyRecords();break;//method call showDailyRecords() case 5 : showSearch();break;//method call showSearch() case 0 : System.out.print(" Do you want to exit(Y/N)?: ");//exit exit = read.next().charAt(0);break; default: System.out.println("Invalid choice.(1-5) or 0 ONLY!!");break;//invalid choice }//end switch if(exit=='Y'||exit=='y'){ System.out.println(" --Thank you. Have a nice day.--");}//end if }while(select!=0||exit=='n'||exit=='N');//end do while }//end main
//method showAboutUs() public static void showAboutUs() { //display About Us System.out.println(); System.out.println(" ABOUT US"); System.out.println("--------------------------------------------------------------------------"); System.out.println("Welcome to The Bowls. Here we provide service of food ordering" + "\and satisfy your cravings." + " We exists to connect local Malaysians to each other through great-tasting bowl noodle + " while also supporting local farmers and utilizing the freshest ingredients." ); System.out.println("------------------------------------------------- -------------------------"); }//end method showAboutUs()
//method showFoodMenu()
public static void showFoodMenu() { //declaration and initialization String foodmenu []= {"Burger","Fries","Soda"} ;//store food menu String food [] = new String [3]; food[0] = (" Burger:"+ " 1. A hamburger consisting of one or more cooked pattiesusually ground meat beefplaced inside a bun."+ " UsThe patty is grilled. A hamburger topped with cheese."); food[1] = (" Fries:"+ " 1. A thin strip of potato, usually cut 3 to 4 inches in length. "+ " 2. That are deep fried until they are golden brown"+ " 3. Crisp textured on the outside while remaining white and soft on the inside"); food[2] = (" Soda:"+ " 1. The base is made with a puree of roasted fruit"+ " which caramelizes the fresh fruit and really intensifies the flavor. "+ " 2. Added some fresh mint and cold club soda );
int choice; char Continue='\0'; do{ //display code and food System.out.println(); System.out.println(" Food Menu"); System.out.println("----------------------------"); System.out.println("Code Food"); System.out.println("----------------------------"); System.out.println(" 1 " + food[0]); System.out.println(" 2 " + food[1]); System.out.println(" 3 " + food[2]); System.out.println("----------------------------"); //ask user to input System.out.print("Choose a choice between(1-3): "); choice = read.nextInt(); //display food switch(choice){ case 1 : System.out.print(food[choice - 1]);break; case 2 : System.out.print(food[choice - 1]);break; case 3 : System.out.print(food[choice - 1]);break;
default: System.out.println("Invalid choice. (1-3) ONLY!!");break;//invalid code }//end switch(choice) if(choice==1||choice==2||choice==3){ System.out.println(); System.out.print(" Do you want to continue(Y/N)?: "); Continue = read.next().charAt(0);}//end if
}while(choice!=1&&choice!=2&&choice!=3||Continue=='Y'||Continue=='y');// end do while loop }//end method Food Menu()
//method showAddFoodOrder() public static void showAddFoodOrder() { //declaration and initialization
public double subTotal; public static double runningTotal; private static double itemPrice; static boolean ordering = true; static Scanner input = new Scanner(System.in);
public static void menu() { System.out.println("Welcome 1. Burger ($2.00) 2. Fries ($1.50) 3. Soda ($1.00) 4. Done"); }
public static double itemPrice(int foodItem) { if (foodItem == 1) { // burger= $2.00 System.out.println("You've ordered a burger"); itemPrice = 2.00; } if (foodItem == 2) { // fries = $1.50 System.out.println("You've ordered fries"); itemPrice = 1.50; } if (foodItem == 3) { // soda = $1.00 System.out.println("You've ordered a soda"); itemPrice = 1.00; } quantity(); return itemPrice; }
public static double quantity() { System.out.println("Enter quantity"); double quantity = input.nextDouble(); subTotal(quantity, itemPrice); return quantity; }
public static double subTotal(double quantity, double itemPrice) { double subTotal = quantity * itemPrice; System.out.println("Subtotal: " + subTotal); return subTotal; }
public static void done(double runningTotal) { ordering = false; System.out.println(runningTotal); System.out.println("Enjoy your meal"); }
public static void main(String[] args) { int menuOption; int foodItem = 0; input = new Scanner(System.in); do { double runningTotal = 0; menu(); menuOption = input.nextInt(); switch (menuOption) { case 1: foodItem = 1; itemPrice(foodItem); break; case 2: foodItem = 2; itemPrice(foodItem); break; case 3: foodItem = 3; itemPrice(foodItem); break; case 4: done(runningTotal); break; default: System.out.println("Invalid option."); } } while (ordering); { subTotal(quantity(), itemPrice(foodItem)); runningTotal = runningTotal + subTotal(quantity(), itemPrice(foodItem)); } } }}//end method showAddFoodOrder()
//method showDeleteRecord()
public static void showDeleteRecord(String[] args){ int i,j; boolean delete=false; String [] ordermenu = {"burger","fries","soda"}; int arrayLength = ordermenu.length; System.out.print("Enter the order that you want to delete:"); String deleteName = input.next(); for (i=0; i < arrayLength; i++){ if(ordermenu[i].equals(deleteName)){ for(j=i;j< arrayLength-1;j++) ordermenu[j]=ordermenu[j+1]; delete=true; break; }//if }//for if (delete==false) System.out.println("The menu is not in the list."); }//end showDelete Record() //method showSearch() public static void showSearch() { //declaration and initialization int tip; String food; char more='\0',Continue1,Continue2; String food [] = new String [3]; food[0] = (" Burger:"+ " 1. A hamburger consisting of one or more cooked pattiesusually ground meat beefplaced inside a bun."+ " UsThe patty is grilled. A hamburger topped with cheese."); food[1] = (" Fries:"+ " 1. A thin strip of potato, usually cut 3 to 4 inches in length. "+ " 2. That are deep fried until they are golden brown"+ " 3. Crisp textured on the outside while remaining white and soft on the inside"); food[2] = (" Soda:"+ " 1. The base is made with a puree of roasted fruit"+ " which caramelizes the fresh fruit and really intensifies the flavor. "+ " 2. Added some fresh mint and cold club soda );
do{ //ask user to input System.out.println(); System.out.print("Which food you want to search?: "); food = read.nextInt(); switch(food){ case : do{ System.out.println(); read.nextLine(); System.out.print("Search food: ");//input search food = read.nextLine();
//display food switch(food){ case "BURGER" : case "burger" : System.out.print(food[0]);break; case "FRIES" : case "fries" : System.out.print(food[1]);break; case "SODA" : case "soda" : System.out.print(food[2]);break;
default : System.out.print(" No search found");break;//no search found }//end switch
System.out.println(""); System.out.print(" Do you want to continue (Y/N)?: "); Continue1 = read.next().charAt(0);
}while(Continue2=='Y'||Continue2=='y');//end do while loop break; default: System.out.println("Invalid choice. (1-2) ONLY!!");break;//invalid choice }//end switch if(food==1||food==2){ System.out.println(); System.out.print("Do you want to search more(Y/N)?: "); more = read.next().charAt(0);} }while(food!=1&&food!=2||more=='Y'||more=='y');//end do while loop
}//end method showSearch() }//end class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
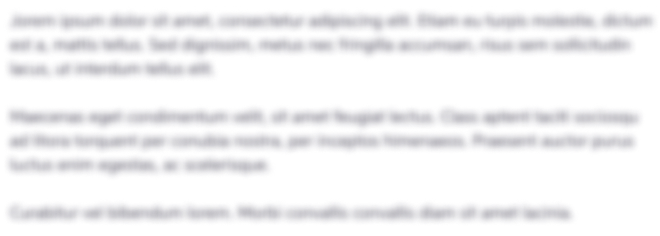
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started