Question
Can someone help me create a JAVA code calledDecimalComparator? It needs to contain the following three public static methods sameIntegralPart - This method takes in
Can someone help me create a JAVA code calledDecimalComparator?
It needs to contain the following three public static methods
- sameIntegralPart- This method takes in two double parameters and returns a boolean: true if the integer part of these two double values are the same, and false otherwise. For example:
sameIntegralPart(12.34, 12.4234) --> true
sameIntegralPart(0.34, 1.423434) --> false
- areEqualWithinPrecision- This method takes in two double parameters and one (precision) integer parameter (say x). It also returns a boolean. The method returns true if the two double arguments are identical UP TO x decimal places, and false otherwise. For example:
areEqualWithinPrecision(23.2345, 23.2346, 3) --> true areEqualWithinPrecision(23.2345, 23.2346, 4) --> false
**Notes:**If the precision argument x is zero, this method boils down to usingsameIntegralPart. If the precision argument x is negative or over 10, the method should return false.
- An overloaded version ofareEqualWithinPrecision. This overloaded version also takes two double parameters, but no integer (precision) parameter. This method behaves like the method in (2), with the precision set to 3 by default. For example:
areEqualWithinPrecision(23.2345, 23.2346) --> true areEqualWithinPrecision(23.2345, 23.2361) --> false
Hints:
- UsesameIntegralPartto codeareEqualWithinPrecision, and use one of the overloaded versions ofareEqualWithinPrecisionto code the other.
- BE CAREFUL:The integer part of a double can be larger than what anintcan accommodate.
- The API methodMath.powshould be useful here. Look it up!
**************************************************************************************
You should copy the skeleton sample program fromDecimalComparator code
public class DecimalComparator {
// Write your 3 methods here
// Use the main method to test your code (optional)
public static void main(String[] args) {
// System.out.println(sameIntegralPart(22.34, 22.37867)); // true
// System.out.println(sameIntegralPart(141457105122.34667, 141457105111.34667)); // false
// System.out.println(areEqualWithinPrecision(-1341.235, -1341.23534667, 3)); // true
// System.out.println(areEqualWithinPrecision(1341.2357, 1341.235346, 4)); // false
// System.out.println(areEqualWithinPrecision(0.2357, 0.235346, 4)); // false
// System.out.println(areEqualWithinPrecision(0.2357, 0.235346)); // true
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
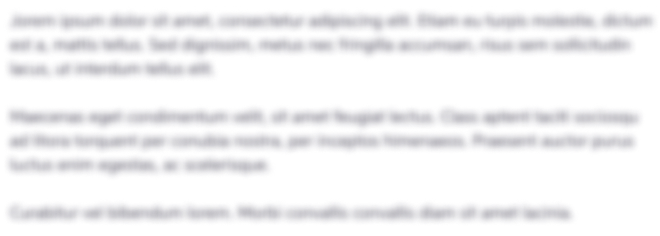
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started