Question
Can someone help me figure out what's wrong with my code? I'm not technically getting any errors but when I run the code and hit
Can someone help me figure out what's wrong with my code? I'm not technically getting any errors but when I run the code and hit Infinite Games it's supposed to ask me how many games I want to play and then I put in my draw for however many times I put in. It's for a rock, paper, scissors game. Here's the code I have:
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package my.rockpaperscissorsgame;
import java.io.FileInputStream; import java.io.InputStream; import javax.swing.ImageIcon; import javax.swing.JOptionPane; import sun.audio.AudioPlayer; import sun.audio.AudioStream;
/** * * @author me */ public class RockPaperScissorsGameGUI extends javax.swing.JFrame { private int totalTime; private int JOption;
/** * Creates new form RockPaperScissorsGameUI */ public RockPaperScissorsGameGUI() { initComponents(); timesLabel.setVisible(false); timesTextField.setVisible(false); }
/** * This method is called from within the constructor to initialize the form. * WARNING: Do NOT modify this code. The content of this method is always * regenerated by the Form Editor. */ @SuppressWarnings("unchecked") // private void initComponents() {
yourFace = new javax.swing.JLabel(); computerFace = new javax.swing.JLabel(); startGameButton = new javax.swing.JButton(); timesTextField = new javax.swing.JTextField(); timesLabel = new javax.swing.JLabel(); computerLabel = new javax.swing.JLabel(); youLabel = new javax.swing.JLabel(); compStatus = new javax.swing.JLabel(); gameOptions = new javax.swing.JComboBox(); yourStatus = new javax.swing.JLabel(); titleLabel = new javax.swing.JLabel();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
yourFace.setIcon(new javax.swing.ImageIcon(getClass().getResource("/my/rockpaperscissorsgame/NormalFace1.png"))); // NOI18N yourFace.addMouseListener(new java.awt.event.MouseAdapter() { public void mouseEntered(java.awt.event.MouseEvent evt) { yourFaceMouseEntered(evt); } public void mouseExited(java.awt.event.MouseEvent evt) { yourFaceMouseExited(evt); } });
computerFace.setIcon(new javax.swing.ImageIcon(getClass().getResource("/my/rockpaperscissorsgame/NormalFace1.png"))); // NOI18N computerFace.addMouseListener(new java.awt.event.MouseAdapter() { public void mouseEntered(java.awt.event.MouseEvent evt) { computerFaceMouseEntered(evt); } public void mouseExited(java.awt.event.MouseEvent evt) { computerFaceMouseExited(evt); } });
startGameButton.setText("Start Play"); startGameButton.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { startGameButtonActionPerformed(evt); } });
timesTextField.setText("times");
timesLabel.setText("Times");
computerLabel.setText("COMPUTER");
youLabel.setText("YOU");
compStatus.setText("Computer Status");
gameOptions.setModel(new javax.swing.DefaultComboBoxModel(new String[] { "One Game", "Five Games", "Infinite Games" })); gameOptions.addItemListener(new java.awt.event.ItemListener() { public void itemStateChanged(java.awt.event.ItemEvent evt) { gameOptionsItemStateChanged(evt); } }); gameOptions.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { gameOptionsActionPerformed(evt); } });
yourStatus.setText("Your Status");
titleLabel.setFont(new java.awt.Font("Tahoma", 1, 24)); // NOI18N titleLabel.setText("Rock, Paper, Scissors Game");
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(101, 101, 101) .addComponent(yourStatus) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) .addComponent(compStatus) .addGap(119, 119, 119)) .addGroup(layout.createSequentialGroup() .addGap(0, 39, Short.MAX_VALUE) .addComponent(yourFace, javax.swing.GroupLayout.PREFERRED_SIZE, 255, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED) .addComponent(startGameButton) .addGap(50, 50, 50) .addComponent(computerFace, javax.swing.GroupLayout.PREFERRED_SIZE, 258, javax.swing.GroupLayout.PREFERRED_SIZE) .addContainerGap()) .addGroup(layout.createSequentialGroup() .addGap(289, 289, 289) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false) .addGroup(layout.createSequentialGroup() .addComponent(timesLabel) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) .addComponent(timesTextField, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addComponent(gameOptions, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(0, 0, Short.MAX_VALUE)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) .addComponent(titleLabel) .addGap(170, 170, 170)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addGap(88, 88, 88) .addComponent(youLabel) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) .addComponent(computerLabel) .addGap(175, 175, 175)) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addContainerGap() .addComponent(titleLabel) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED) .addComponent(gameOptions, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(timesLabel) .addComponent(timesTextField, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(29, 29, 29) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(yourStatus) .addComponent(compStatus)) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(startGameButton) .addComponent(computerFace, javax.swing.GroupLayout.PREFERRED_SIZE, 258, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(yourFace, javax.swing.GroupLayout.PREFERRED_SIZE, 258, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(18, 18, 18) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(computerLabel, javax.swing.GroupLayout.PREFERRED_SIZE, 14, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(youLabel)) .addContainerGap(876, Short.MAX_VALUE)) );
pack(); }//
private void startGameButtonActionPerformed(java.awt.event.ActionEvent evt) { int index = gameOptions.getSelectedIndex(); switch(index) { case 0: theFinalWinner = playOneGame(); changeFaces(theFinalWinner); break; case 1: theFinalWinner = playFiveGames(); changeFaces(theFinalWinner); case 2: theFinalWinner = playInfiniteGames(); timesTextField.setText(strTimes); changeFaces(theFinalWinner); break; } }
private void yourFaceMouseEntered(java.awt.event.MouseEvent evt) { try{ if(theFinalWinner.equals("YOU")) in = new FileInputStream(applauseFile); else if (theFinalWinner.equals("COMPUTER")) in = new FileInputStream(booFile); audioStream = new AudioStream(in); AudioPlayer.player.start(audioStream); } catch (Exception e) { System.out.println("Audio file couldn't play!"); } } private void computerFaceMouseEntered(java.awt.event.MouseEvent evt) { try{ if(theFinalWinner.equals("YOU")) in = new FileInputStream(booFile); else if (theFinalWinner.equals("COMPUTER")) in = new FileInputStream(applauseFile); audioStream = new AudioStream(in); AudioPlayer.player.start(audioStream); } catch (Exception e) { System.out.println("Audio file couldn't play!"); } } private void gameOptionsItemStateChanged(java.awt.event.ItemEvent evt) { changeFaces("TIE"); yourStatus.setText("Your Status"); compStatus.setText("Computer Status"); int index = gameOptions.getSelectedIndex(); switch(index) { case 0: timesLabel.setVisible(false); timesTextField.setVisible(false); break; case 1: timesLabel.setVisible(false); timesTextField.setVisible(false); break; case 2: timesLabel.setVisible(true); timesTextField.setVisible(true); break; } }
private void yourFaceMouseExited(java.awt.event.MouseEvent evt) { AudioPlayer.player.stop(audioStream); }
private void computerFaceMouseExited(java.awt.event.MouseEvent evt) { AudioPlayer.player.stop(audioStream); }
private void gameOptionsActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: }
/** * @param args the command line arguments */ public static void main(String args[]) { java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new RockPaperScissorsGameGUI().setVisible(true); } }); } private String playOneGame() { String theWinner; String yourHand = (String)JOptionPane.showInputDialog(null, "What's your Hand? ", "Please Choose Your Hand", JOptionPane.PLAIN_MESSAGE,null,yourChoice,yourChoice[0]); int compIndex = ((int)(Math.random() * 100) % 3); theWinner = whoIsTheWinner(yourHand, computerHand[compIndex]); return theWinner; } private String playFiveGames() { String theWinner = "TIE"; String yourHand; String compHand; int timesYouWon=0, timesComputerWon=0; for(int index = 0; index<5; ++index) { int compIndex = ((int)(Math.random() * 100) % 3); yourHand = (String)JOptionPane.showInputDialog(null, "What's your Hand? ", "Please Choose Your Hand", JOptionPane.PLAIN_MESSAGE,null,yourChoice,yourChoice[0]); compHand = computerHand[compIndex]; theWinner = whoIsTheWinner(yourHand,compHand); if(theWinner.equals("YOU")) timesYouWon++; else if(theWinner.equals("COMPUTER")) timesComputerWon++; } if (timesYouWon > timesComputerWon) theWinner = "YOU"; else if(timesComputerWon > timesYouWon) theWinner = "COMPUTER"; yourStatus.setText("You won " + timesYouWon +" times"); compStatus.setText("Computer won "+ timesComputerWon + " times"); return theWinner; } private String playInfiniteGames() { String myHand, compHand, theWinner="TIE"; int response = JOptionPane.YES_OPTION; int timesYouWon=0, timesComputerWon=0; int totalTimes=0; while(response == JOptionPane.YES_OPTION) { myHand = (String)JOptionPane.showInputDialog(null, "What's your Hand? ", "Please Choose Your Hand", JOptionPane.PLAIN_MESSAGE,null,yourChoice,yourChoice[0]); int compIndex = ((int)(Math.random() * 100) % 3); compHand = computerHand[compIndex]; theWinner = whoIsTheWinner(myHand, compHand); if(theWinner.equals("YOU")) timesYouWon++; else if(theWinner.equals("COMPUTER")) timesComputerWon++; totalTime++; response=JOptionPane.showConfirmDialog(null, "Keep Playing", "Computer is asking you...", JOption); } strTimes = "" + totalTimes; if (timesYouWon > timesComputerWon) theWinner = "YOU"; else if(timesComputerWon > timesYouWon) theWinner = "COMPUTER"; return theWinner; } private String whoIsTheWinner(String myHand, String compHand) { yourStatus.setText(myHand); compStatus.setText(compHand); String theWinner = "TIE"; switch (myHand) { case "Rock": if(compHand.equals("Paper")) theWinner="COMPUTER"; else if (compHand.equals("Scissors")) theWinner="YOU"; break; case "Paper": if(compHand.equals("Rock")) theWinner="YOU"; else if (compHand.equals("Scissors")) theWinner="COMPUTER"; break; case "Scissors": if(compHand.equals("Rock")) theWinner="COMPUTER"; else if (compHand.equals("Paper")) theWinner="YOU"; break; } return theWinner; } private void changeFaces(String fWinner) { switch (fWinner) { case "YOU": yourFace.setIcon(happyImg); computerFace.setIcon(sadImg); break; case "COMPUTER": yourFace.setIcon(sadImg); computerFace.setIcon(happyImg); break; case "TIE": yourFace.setIcon(normalImg); computerFace.setIcon(normalImg); } } String[] computerHand = {"Rock", "Paper", "Scissors"}; String[] yourChoice = {"Rock", "Paper", "Scissors"}; String theFinalWinner = ""; ImageIcon sadImg = new ImageIcon("my path file"); ImageIcon happyImg = new ImageIcon("my path file"); ImageIcon normalImg = new ImageIcon("my path file"); String booFile = "my path file"; String applauseFile = "my path file"; AudioStream audioStream; InputStream in; String strTimes="";
// Variables declaration - do not modify private javax.swing.JLabel compStatus; private javax.swing.JLabel computerFace; private javax.swing.JLabel computerLabel; private javax.swing.JComboBox gameOptions; private javax.swing.JButton startGameButton; private javax.swing.JLabel timesLabel; private javax.swing.JTextField timesTextField; private javax.swing.JLabel titleLabel; private javax.swing.JLabel youLabel; private javax.swing.JLabel yourFace; private javax.swing.JLabel yourStatus; // End of variables declaration
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
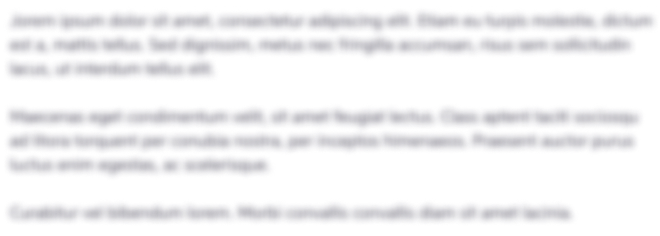
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started