Question
Can someone help me on how/ why this will NOTadd object to the list when entered in text boxes but will diplay them fine when
Can someone help me on how/ why this will NOTadd object to the list when entered in text boxes but will diplay them fine when they are added manually. And how would i go about adding them. thanks.
package scoreboardapp;
public class Scoreboard {
private GameEntry firstNode;
public Scoreboard() { this.firstNode = null; }
public GameEntry getFirstNode() { return firstNode; }
public void setFirstNode(GameEntry firstNode) { this.firstNode = firstNode; }
public GameEntry addTolist(String name, int score) { GameEntry newNode; GameEntry currentNode;
newNode = new GameEntry(name, score);
if (this.firstNode == null) { this.firstNode = newNode; } else { currentNode = this.firstNode; while (currentNode.nextElement != null) { currentNode = currentNode.nextElement; } currentNode.nextElement = newNode; } return newNode; }//END ADDTOLIST()
public GameEntry printInfo() { GameEntry currentNode = null;
if (this.firstNode == null) { return null; } else { currentNode = this.firstNode; while (currentNode != null) {
// System.out.println(currentNode); currentNode = currentNode.nextElement; } } return currentNode.nextElement;
}//END PRINT ELEMENTS()
}//END SCOREBOARD CLASS
package scoreboardapp;
public class GameEntry {
public String gamersName; public int gamersScore; GameEntry nextElement;
public GameEntry() { this.gamersName = null; this.gamersScore = 0; nextElement = null; }
public GameEntry(String gamersName, int gamersScore) { this.gamersName = gamersName; this.gamersScore = gamersScore; }
public String getGamersName() { return gamersName; }
public void setGamersName(String gamersName) { this.gamersName = gamersName; }
public int getGamersScore() { return gamersScore; }
public void setGamersScore(int gamersScore) { this.gamersScore = gamersScore; }
public GameEntry getNextElement() { return nextElement; }
public void setNextElement(GameEntry nextElement) { this.nextElement = nextElement; }
@Override public String toString() { return ("Gamers Name: " + this.gamersName + " " + "Gamers Score: " + this.gamersScore); }
}//End Game Entry Class
package scoreboardapp;
import java.awt.GraphicsConfiguration; import java.util.Collections; import java.util.LinkedList;
public class TheBoard extends javax.swing.JFrame { String gamersName; int gamersScore; int temp = 0; // To store the store temporary for compare purpose int position = 0; // To store the position of the highest score student
GameEntry myGameEntry = new GameEntry(); Scoreboard myScoreboard = new Scoreboard(); ScoreCheckComp myScoreCheckComp = new ScoreCheckComp(); public TheBoard(GraphicsConfiguration gc) { super(gc); }
/** * Creates new form TheBoard */ public TheBoard() { initComponents(); }
/** * This method is called from within the constructor to initialize the form. * WARNING: Do NOT modify this code. The content of this method is always * regenerated by the Form Editor. */ @SuppressWarnings("unchecked") //
jLabel1 = new javax.swing.JLabel(); txtGamersName = new javax.swing.JTextField(); txtGamersScore = new javax.swing.JTextField(); btnAddScore = new javax.swing.JButton(); btnExit = new javax.swing.JButton(); lstPrintOut = new java.awt.List(); lblTopScores = new javax.swing.JLabel(); btnClear = new javax.swing.JButton(); btnCalculate = new javax.swing.JButton();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel1.setText("The Top Scores for the Game Board");
txtGamersName.setBorder(javax.swing.BorderFactory.createTitledBorder("Enter Gamers Name:")); txtGamersName.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { txtGamersNameActionPerformed(evt); } });
txtGamersScore.setBorder(javax.swing.BorderFactory.createTitledBorder("Enter Game Score:"));
btnAddScore.setText("Add Score"); btnAddScore.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { btnAddScoreActionPerformed(evt); } });
btnExit.setText("Exit"); btnExit.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { btnExitActionPerformed(evt); } });
lstPrintOut.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { lstPrintOutActionPerformed(evt); } });
lblTopScores.setBorder(javax.swing.BorderFactory.createTitledBorder("Gamers Name and Top Score"));
btnClear.setText("Clear"); btnClear.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { btnClearActionPerformed(evt); } });
btnCalculate.setText("Calculate"); btnCalculate.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { btnCalculateActionPerformed(evt); } });
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addContainerGap() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addComponent(jLabel1) .addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addComponent(txtGamersName, javax.swing.GroupLayout.PREFERRED_SIZE, 199, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(txtGamersScore, javax.swing.GroupLayout.PREFERRED_SIZE, 187, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGroup(layout.createSequentialGroup() .addComponent(lstPrintOut, javax.swing.GroupLayout.PREFERRED_SIZE, 345, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(85, 85, 85) .addComponent(lblTopScores, javax.swing.GroupLayout.PREFERRED_SIZE, 294, javax.swing.GroupLayout.PREFERRED_SIZE))) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(35, 35, 35) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(btnAddScore, javax.swing.GroupLayout.Alignment.TRAILING) .addComponent(btnExit, javax.swing.GroupLayout.Alignment.TRAILING)) .addComponent(btnClear))) .addGroup(layout.createSequentialGroup() .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(btnCalculate))) .addGap(41, 41, 41)))) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addComponent(jLabel1) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(43, 43, 43) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(txtGamersName, javax.swing.GroupLayout.PREFERRED_SIZE, 80, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(txtGamersScore, javax.swing.GroupLayout.PREFERRED_SIZE, 80, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(11, 11, 11) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 27, Short.MAX_VALUE) .addComponent(lstPrintOut, javax.swing.GroupLayout.PREFERRED_SIZE, 296, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(18, 18, 18)) .addGroup(layout.createSequentialGroup() .addGap(214, 214, 214) .addComponent(btnCalculate) .addGap(0, 0, Short.MAX_VALUE)))) .addGroup(layout.createSequentialGroup() .addGap(64, 64, 64) .addComponent(btnAddScore) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED) .addComponent(btnClear) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) .addComponent(btnExit)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) .addComponent(lblTopScores, javax.swing.GroupLayout.PREFERRED_SIZE, 252, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(0, 0, Short.MAX_VALUE))) .addGap(35, 35, 35)) );
pack(); }//
private void btnExitActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: System.exit(0); }
private void btnAddScoreActionPerformed(java.awt.event.ActionEvent evt) {
///////////////////// Add Score Button////////////////////////////////////////// //READ THE USERS' INPUT gamersName = txtGamersName.getText(); gamersScore = Integer.parseInt(txtGamersScore.getText());
myGameEntry.setGamersName(gamersName); myGameEntry.setGamersScore(gamersScore); lstPrintOut.add(myGameEntry.toString()); }
private void txtGamersNameActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: }
private void btnClearActionPerformed(java.awt.event.ActionEvent evt) { //clear txtGamersName.setText(""); txtGamersScore.setText(""); }
private void lstPrintOutActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: }
private void btnCalculateActionPerformed(java.awt.event.ActionEvent evt) { // CALCULATE BUTTON LinkedList
}
/** * @param args the command line arguments */ public static void main(String args[]) { /* Set the Nimbus look and feel */ //
/* Create and display the form */ java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new TheBoard().setVisible(true); } }); }
// Variables declaration - do not modify private javax.swing.JButton btnAddScore; private javax.swing.JButton btnCalculate; private javax.swing.JButton btnClear; private javax.swing.JButton btnExit; private javax.swing.JLabel jLabel1; private javax.swing.JLabel lblTopScores; private java.awt.List lstPrintOut; private javax.swing.JTextField txtGamersName; private javax.swing.JTextField txtGamersScore; // End of variables declaration }
package scoreboardapp; import java.util.Comparator;
public class ScoreCheckComp implements Comparator
@Override public int compare(GameEntry gamer1, GameEntry gamer2) {
if (gamer1.getGamersScore() < gamer2.getGamersScore()) { return 1;//object bigger; } else { return -1;//object smaller; }
}//END CHECK }//END CLASS
Step by Step Solution
There are 3 Steps involved in it
Step: 1
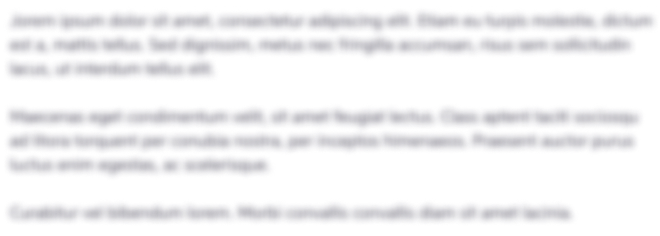
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started