Question
Can someone help me with the following project Playfair, Vigenere, Caesar and Substitution. All they require a special string key which is used in the
Can someone help me with the following project
Playfair, Vigenere, Caesar and Substitution. All they require a special string key which is used in the encrypting process. This key should be specified in the constructor for all of these classes. You may also want to include a setKey(String key) method as well.
The classes Coder and KeyCoder should contain any and all common elds and methods of the subclasses; specifically, Coder should contain two abstract methods public abstract String encrypt(String s) and public abstract String decrypt(String s). Both Coder and KeyCoder will be abstract classes.
Driver Program : Your driver program P1XXX.java will have two main components. The first component will get input from the user which specifies: 1. the series of encryption schemes to use to encrypt/decrypt. 2. whether or not to encryt or decrypt. You should allow the user to specify up to 10 ciphers. For example, the user might first choose to use array encryption, following by a substitution encryption using the key "Westminster", followed by another array encryption, and ending with a Caesar encryption. The second component of the driver program will them perform the encryption/decryption. Read in a single line of text, pass it through whatever series of ciphers the user speci ed, and then output the nal result. The series of ciphers should be stored in an array of Coders. This array should be declared as follows: Coder encryptionSeries[]; encryptionSeries[0] should be set to the rst cipher specifed, encryptionSeries[1] to the second, and so on. The general code for encryption should look something like the following: initialize encryptionSeries[] base on user input read in a line for(i=0; i import java.util.Objects; public class ArrayCoder extends Coder { // main method to test the two encryption classes public static void main(String args[]) { ArrayCoder p1 = new ArrayCoder(); String cipher = p1.encrypt("Send more monkeys!my name is albert Einstein what do you want from me"); String decipher = p1.decrypt(cipher); System.out.println("encrypted: "+cipher); System.out.println("decrypted: "+decipher); } Character key[][]; /** * Constructor */ public ArrayCoder() { key = new Character[5][5]; } public String encrypt(String plaintext) { plaintext = refine(plaintext); // remove all special characters form plaintext StringBuilder ct = new StringBuilder(); // output int strt = 0; // start of the plaintext int end = plaintext.length(); // end of the plaintext // when the plain text is more than 25 characters in length // then we have to encrypt in blocks of 25 while(strt String chunck = plaintext.substring(strt, Math.min(strt+25, end)); // creat a block of 25 characters buildMatrix(chunck); // encrypt it ct.append(parseMatrix()).append(' '); // add the encrypted block to the output strt += 25; // increment the pointer } return ct.toString(); } public String decrypt(String ciphertext) { ciphertext = refine(ciphertext); // remove all special characters form ciphertext StringBuilder pt = new StringBuilder(); // output int strt = 0; // start of the ciphertext int end = ciphertext.length(); // start of the ciphertext // when the ciphe text is more than 25 characters in length // then we have to encrypt in blocks of 25 while(strt String chunck = ciphertext.substring(strt, Math.min(strt+25, end)); buildMatrix(chunck); pt.append(refine(parseMatrix())); strt += 25; } return pt.toString(); } /** * This method takes one character at a time and add it to the key matrix * in row major order */ private void buildMatrix(String s) { int i = 0; int j = 0; for(Character c : s.toCharArray()) { key[i][j++] = c; // add it to the key at location [i,j] if(j == 5) { j = 0; i++; } } int k =0; while(!(i == 5 && j == 0 )) { key[i][j++] = (char)(65+(k++)); if(j == 5) { j = 0; i++; } } } // this method removes all special characters from the // string and returns a uppder case of that string // add some more special characters if you want private String refine(String s) { return s.replaceAll(" ", ""). replaceAll(",", ""). replaceAll(":", ""). replaceAll("!", ""). replaceAll(";", ""). toUpperCase(); } /** * This method traverses the key matrix in column major order * To generate the cipher text */ private String parseMatrix() { StringBuilder parser = new StringBuilder(); for(int i = 0; i for(int j = 0; j parser.append(key[j][i]); } parser.append(' '); } return parser.toString(); } } ////////////////////// public class VigenereCoder { public static void main(String[] args) { String key = "WAX"; String message = "WESTMINSTER"; String encodedMessage = encodeMessage(message, key); System.out.println("String: " + message); System.out.println("Encoded message: " + encodedMessage); System.out.println("Decoded message: " + decodeMesssage(encodedMessage, key)); } private static String decodeMesssage(String encodedMessage, String key) { String res = ""; encodedMessage = encodedMessage.toUpperCase(); for (int i = 0, j = 0; i = 'A' && c private static String encodeMessage(String message, String key) { String res = ""; message = message.toUpperCase(); for (int i = 0, j = 0; i = 'A' && c } ////////////////////////////////// import java.util.Scanner; public class SubstitutionCoder extends KeyCoder{ public static void main(String[] args) { Scanner input = new Scanner(System.in); String strKey, letters = "ZYXWVUTSRQPONMLKJIHGFEDCBA"; String key = ""; String stringToEncrypt, encryptedString = ""; //prompt and read the read from the user System.out.print("Enter the key: "); strKey = input.nextLine(); //prompt and read the string to be encrypted System.out.print("Enter the string to be encrypted: "); stringToEncrypt = input.nextLine(); input.close(); //convert the letters in key to upper case strKey = strKey.toUpperCase(); //run a loop through the letters in the key entered by user for(int i = 0; i { //add each character to key removing the duplicates if(!key.contains(strKey.charAt(i)+"")) key = key + strKey.charAt(i); } //add the rest of the letters in reverse order to the key for(int i = 0 ; i { if(!key.contains(letters.charAt(i)+"")) key = key + letters.charAt(i); } int index; //convert the string to be encrypted to upper case stringToEncrypt = stringToEncrypt.toUpperCase(); for(int i = 0; i { //get the index of each letter in string to be encrypted index = (int)stringToEncrypt.charAt(i) - 65; //get the encrypted letter and append it to encrypted string encryptedString = encryptedString + key.charAt(index); } //display the encrypted string System.out.println(" The encrypted string is: " + encryptedString); } } //////////////////////////////////////////////////// import java.util.*; public class PlayfairCoder extends KeyCoder { char [][] grid = new char[5][5]; int [] rowVal = new int[26]; int [] colVal = new int[26]; public void buildGrid(String key) { key = clean(key); // build Playfair grid grid[0][0] = key.charAt(0); int r=0, c=1; for(int i=1; i char ch = key.charAt(i); if (ch == 'J') // (skipping any J's) continue; if (key.substring(0,i).indexOf(ch) == -1) { grid[r][c] = ch; rowVal[ch-'A'] = r; // save location of each letter in grid colVal[ch-'A'] = c; if (++c == 5) { c = 0; r++; } } } for(char ch = 'Z'; ch >= 'A'; ch--) { // then use remaining unused letters if (ch == 'J') continue; if (key.indexOf(ch) == -1) { grid[r][c] = ch; rowVal[ch-'A'] = r; colVal[ch-'A'] = c; if (++c == 5) { c = 0; r++; } } } rowVal[9] = rowVal[8]; // set J location to I loction colVal[9] = colVal[8]; } public String clean(String s) { String ans = ""; for(int i=0; i if (Character.isLetter(s.charAt(i))) ans += s.charAt(i); } ans = ans.toUpperCase(); return ans; } public String encrypt(String plaintext, String key) { buildGrid(key); String cyphertext = ""; plaintext = clean(plaintext); if (plaintext.length()%2 == 1) plaintext += 'X'; for(int i=0; i int ch1 = plaintext.charAt(i) - 'A'; int ch2 = plaintext.charAt(i+1) - 'A'; if (rowVal[ch1] == rowVal[ch2]) { cyphertext += grid[rowVal[ch1]][(colVal[ch1]+1)%5]; cyphertext += grid[rowVal[ch2]][(colVal[ch2]+1)%5]; } else if (colVal[ch1] == colVal[ch2]) { cyphertext += grid[(rowVal[ch1]+1)%5][colVal[ch1]]; cyphertext += grid[(rowVal[ch2]+1)%5][colVal[ch2]]; } else { cyphertext += grid[rowVal[ch1]][colVal[ch2]]; cyphertext += grid[rowVal[ch2]][colVal[ch1]]; } } return cyphertext; } public String decrypt(String plaintext, String key) { buildGrid(key); String cyphertext = ""; plaintext = clean(plaintext); if (plaintext.length()%2 == 1) plaintext += 'X'; for(int i=0; i int ch1 = plaintext.charAt(i) - 'A'; int ch2 = plaintext.charAt(i+1) - 'A'; if (rowVal[ch1] == rowVal[ch2]) { cyphertext += grid[rowVal[ch1]][(colVal[ch1]+4)%5]; cyphertext += grid[rowVal[ch2]][(colVal[ch2]+4)%5]; } else if (colVal[ch1] == colVal[ch2]) { cyphertext += grid[(rowVal[ch1]+4)%5][colVal[ch1]]; cyphertext += grid[(rowVal[ch2]+4)%5][colVal[ch2]]; } else { cyphertext += grid[rowVal[ch1]][colVal[ch2]]; cyphertext += grid[rowVal[ch2]][colVal[ch1]]; } } return cyphertext; } public static void main(String [] args) { Scanner in = new Scanner(System.in); Playfair coder = new Playfair(); String plaintext; System.out.print("Enter text to encrypt "); plaintext = in.nextLine(); String cyphertext = coder.encrypt(plaintext, "red hot chili peppers"); System.out.println("Encrypted text = " + cyphertext); plaintext = coder.decrypt(cyphertext, "red hot chili peppers"); System.out.println("Decrypted text = " + plaintext); } } //////////////////////////////// class Caesar extends VigenereCoder { // Encrypts text using a shift od s public static StringBuffer encrypt(String text, int s) { StringBuffer result = new StringBuffer(); text = text.toUpperCase(); for (int i = 0; i char ch = (char) (((int) text.charAt(i) + s - 65) % 26 + 65); result.append(ch); } return result; } // Driver code public static void main(String[] args) { String text = "westminster"; int s = 3; System.out.println("Text : " + text); System.out.println("Shift : " + s); System.out.println("Cipher: " + encrypt(text, s)); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
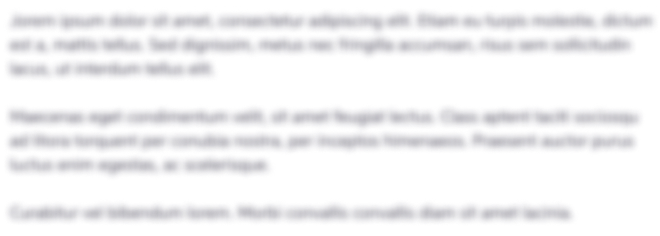
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started