Question
Can someone helps me with this python HW? Implement a class called Card to represent a playing card. The class should have the following methods
Can someone helps me with this python HW?
Implement a class called Card to represent a playing card. The class should have the following methods & attributes:
__init__(self, rank, suite) - creates a new card and sets private attributes.
rankis an integer in the range 1 - 13 indicating the ranks from Ace (1) to King (13).
suite is a single character - 'd', 'c', 'h', or 's' - indicating the suit (diamonds, clubs, hearts, or spades)
rank - returns the card's rank
suite - returns the full name of the card's suit
value - returns the card's Blackjack value (Ace = 1, face cards = 10)
__repr__(self) - returns the card's full name (e.g. "Ace of Spades" or "Three of Hearts")
- Save your class in a file called card.py
- Other programs should be able to import your class and create a card like this:
from card import Card c = Card(1,"s") print(c.rank) # should print 1 print(c.suite) # should print 'Spades' print(c.value) # should print 10 for face cards & card rank for others print(c) # should print 'Ace of Spades'
Hint
Several of your class attributes will need to be defined as @property methods. See the class notes on using @property and private attributes.
Several of your methods will need to match card rank and suit values with full names. Consider whether a list or a dictionary is better for each type of information.
Extra Credit
Write a function in card.py, but not part of the Card class, that asks the user whether to draw a card. If the user agrees, the function should create a new card with randomly selected value and suit. The program should display the combined total of Blackjack values after each draw, and prompt the user to draw another card until the total exceeds 21.
I have the program figured out. I only need help with extra credit. Thank you!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
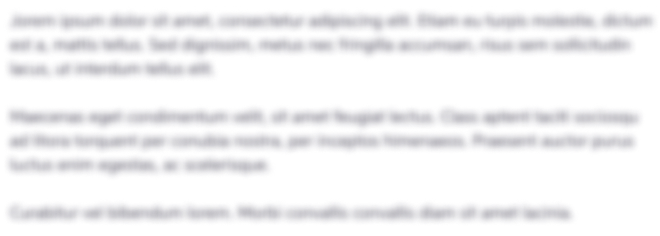
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started