Question
Can someone please assist me with the program question below. it has to do with Binary search trees and finding a maxkey(); any help will
Can someone please assist me with the program question below. it has to do with Binary search trees and finding a maxkey();
any help will be appreciated. below i attached the CPP file
#pragma once
#include
using namespace std;
template
struct Node
{
Node() : parent_(0), left_(0), right_(0) { }
V operator*() { return(value_); }
bool IsRoot() const { return(parent_ == NULL); }
bool IsExternal() const { return((left_ == NULL) && (right_ == NULL)); }
void Show(ostream& stream);
K key_;
Node* left_;
Node* parent_;
Node* right_;
V value_;
};
template
class BinarySearchTree
{
public:
class Iterator
{
public:
Iterator() { }
Iterator MaxKey();
Iterator(Node
const Node
Node
bool operator==(const Iterator& p) const
{ return(node_ == p.node_); }
bool operator!=(const Iterator& p) const
{ return(node_ != p.node_); }
Iterator& operator++();
private:
Node
friend class BinarySearchTree;
};
BinarySearchTree();
Iterator Find(const K& key);
Iterator Insert(const K& key, const V& value);
bool Erase(const K& key);
void Erase(const Iterator& i);
void Show(ostream& stream);
int Size() const;
bool Empty() const;
Iterator Begin();
Iterator End();
private:
Node
void ExpandExternal(Node
Node
Node
Node
Node
int size_;
Node
};
template
BinarySearchTree
{
size_ = 0;
// Create the super root and make its left child the logical root of the tree.
superRoot_ = new Node
ExpandExternal(superRoot_);
root_ = superRoot_->left_;
}
template
typename BinarySearchTree
{
Node
if (!node->IsExternal()) {
return(Iterator(node));
}
else { // not found, return end iterator
return(End());
}
}
template
Node
{
if (node->IsExternal()) {
return(node);
}
if (key == node->key_) {
return(node);
}
else if (key key_) {
return(Finder(key, node->left_));
}
else {
return(Finder(key, node->right_));
}
}
template
void BinarySearchTree
{
root_->Show(stream);
return;
}
template
void Node
{
if (!IsExternal()) {
left_->Show(stream);
stream
right_->Show(stream);
}
return;
}
template
typename BinarySearchTree
BinarySearchTree
{
Node
return(Iterator(node));
}
template
Node
{
Node
while (!node->IsExternal()) {
node = Finder(key, node->right_);
}
ExpandExternal(node);
node->key_ = key;
node->value_ = value;
++size_;
return(node);
}
template
bool BinarySearchTree
{
Node
if (!node->IsExternal()) {
Eraser(node);
return(true);
}
else {
return(false);
}
}
template
void BinarySearchTree
{
Eraser(i.node_);
}
template
Node
{
Node
// Is the node's left child an external node?
if (node->left_->IsExternal()) {
remove = node->left_;
}
else if (node->right_->IsExternal()) {
remove = node->right_;
}
else {
// Both children are internal nodes. Find the node's logical successor by
// going down to the right child subtree and then all the way down to the
// bottom left corner of that subtree.
remove = node->right_;
do {
remove = remove->left_;
} while (!remove->IsExternal());
Node
node->key_ = removeParent->key_;
node->value_ = removeParent->value_;
}
--size_;
return(RemoveAboveExternal(remove));
}
template
void BinarySearchTree
{
node->left_ = new Node
node->left_->parent_ = node;
node->right_ = new Node
node->right_->parent_ = node;
size_ += 2;
}
template
Node
{
Node
Node
Node
if (parent == root_) {
root_ = sibling;
sibling->parent_ = NULL;
}
else {
Node
if (parent == grandParent->left_) {
grandParent->left_ = sibling;
}
else {
grandParent->right_ = sibling;
}
sibling->parent_ = grandParent;
}
delete child;
delete parent;
size_ -= 2;
return(sibling);
}
template
int BinarySearchTree
{
return(size_);
}
template
bool BinarySearchTree
{
return(size_ == 0);
}
template
typename BinarySearchTree
{
Node
// Travel down the left side of the tree to the lower-left external node.
while (!node->IsExternal()) {
node = node->left_;
}
// Return an iterator to the lower-leftmost internal node.
return(Iterator(node->parent_));
}
template
typename BinarySearchTree
{
return(Iterator(superRoot_));
}
template
typename BinarySearchTree
{
// Is there a right subtree?
Node
if (!next->IsExternal()) {
// Yes, go down to its lowest node.
do {
node_ = next;
next = next->left_;
} while (!next->IsExternal());
}
else {
// No, go up until we see the parent of a left subtree.
next = node_->parent_;
while (node_ == next->right_) {
node_ = next;
next = next->parent_;
}
node_ = next;
}
return(*this);
}
Add a public member function to class BinarySearchTree that returns an iterator to the node with the largest key. Do not change the contents of the search tree. Your function should have the declaration: Hint, Iterator MaxKey ): Study the two functions Iterator Find (const K& key) and its helper function Node
Step by Step Solution
There are 3 Steps involved in it
Step: 1
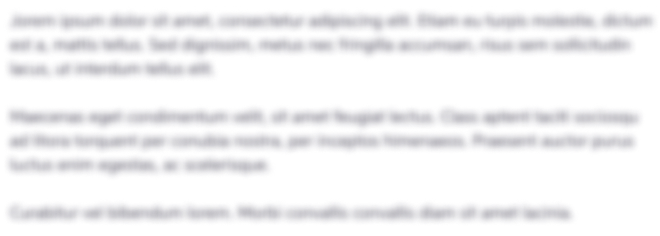
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started