Question
Can someone please check why my code isn't giving the necessary outputs. I have written it in NodeJS with JavaScript as a Command Line Application.
Can someone please check why my code isn't giving the necessary outputs. I have written it in NodeJS with JavaScript as a Command Line Application. It is intended to perform the following operations: Here's what I need: implement a simple Toy Robot game. The game initialises with an empty 5 x 5 board with its own coordinate system: the bottom left of the board is (1, 1) (row 1, column 1), and the top right corner of the board is (5, 5). When the game starts, it responds to the following user commands: * PLACE_ROBOT ROW,COL,FACING (This command places a robot at a given coordinate with an initial Facing direction) * PLACE_WALL ROW,COL This command places a wall at the given coordinate. If the target location is empty, then it adds a wall to it. The user can add as many walls as they like until the board is filled. If the target location is occupied (by the robot, or another wall), then this command is ignored. Invalid coordinates are ignored. *REPORT The game prints out the current location and facing direction of the robot. If there are no robots on the board, this command is ignored. *MOVE The MOVE command moves the robot 1 space forward in the direction it is currently facing. If there are no robots on the board, this command is ignored. If there is a wall in front of the robot, this command is ignored. If the robot has already reached the edge of the board, a MOVE command towards the edge warps the robot to the opposite of the board. *LEFT / RIGHT The turn commands LEFT and RIGHT, turns the robot 90 degrees to its current left or right. If there are no robots on the board, this command is ignored. Test Data Here are some examples of test data: PLACE_ROBOT 2,3,NORTH PLACE_WALL 3,5 MOVE MOVE RIGHT MOVE MOVE MOVE REPORT # the app should print: 1,4,EAST PLACE_ROBOT 2,2,WEST PLACE_WALL 1,1 PLACE_WALL 2,2 PLACE_WALL 1,3 LEFT LEFT MOVE REPORT # the app should print: 3,2,EAST I have written this code:
// Import necessary libraries
const readline = require('readline');
// Initialize board and robot variables
let board = [];
let robot = null;
console.log('Starting program...');
// your program code here
// Initialize readline interface for user input
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
// Function to handle user commands
function handleCommand(input) {
console.log(`User input: ${input}`);
const command = input.split(',');
switch (command[0]) {
case 'PLACE_ROBOT':
const row = parseInt(command[1]);
const col = parseInt(command[2]);
const facing = command[3];
placeRobot(row, col, facing);
break;
case 'PLACE_WALL':
const wallRow = parseInt(command[1]);
const wallCol = parseInt(command[2]);
placeWall(wallRow, wallCol);
break;
case 'REPORT':
report();
break;
case 'MOVE':
moveRobot();
break;
case 'LEFT':
turnRobot(-1);
break;
case 'RIGHT':
turnRobot(1);
break;
default:
console.log(`Invalid command: ${input}`);
}
}
// Function to place robot on board
function placeRobot(row, col, facing) {
if (isValidCoord(row, col) && isValidFacing(facing)) {
robot = {
row: row,
col: col,
facing: facing
};
}
}
// Function to place wall on board
function placeWall(row, col) {
if (isValidCoord(row, col) && isEmpty(row, col)) {
board[row-1][col-1] = 'X';
}
}
// Function to move robot forward
function moveRobot() {
if (robot) {
let newRow = robot.row;
let newCol = robot.col;
switch (robot.facing) {
case 'NORTH':
newRow++;
break;
case 'SOUTH':
newRow--;
break;
case 'EAST':
newCol++;
break;
case 'WEST':
newCol--;
break;
}
if (isValidCoord(newRow, newCol) && isEmpty(newRow, newCol)) {
robot.row = newRow;
robot.col = newCol;
} else {
wrapRobot();
}
}
}
// Function to turn robot left or right
function turnRobot(direction) {
if (robot) {
const facings = ['NORTH', 'EAST', 'SOUTH', 'WEST'];
const index = facings.indexOf(robot.facing);
const newIndex = (index + direction + facings.length) % facings.length;
robot.facing = facings[newIndex];
}
}
// Function to report current robot position and facing direction
function report() {
if (robot) {
console.log(`${robot.row},${robot.col},${robot.facing}`);
}
}
// Function to check if coordinates are valid
function isValidCoord(row, col) {
return row >= 1 && row <= 5 && col >= 1 && col <= 5;
}
// Function to check if facing direction is valid
function isValidFacing(facing) {
const validFacings = ['NORTH', 'SOUTH', 'EAST', 'WEST'];
return validFacings.includes(facing);
}
// Function to check if board location is empty
function isEmpty(row, col) {
return board[row-1][col-1] === undefined;
}
// Function to wrap robot to other side of board if it moves off the edge
function wrapRobot() {
let newRow = robot.row;
let newCol = robot.col;
switch (robot.facing) {
case 'NORTH':
newRow = 1;
break;
case 'SOUTH':
newRow = 5;
break;
case 'EAST':
newCol = 1;
break;
case 'WEST':
newCol = 5;
break;
}
if (isEmpty(newRow, newCol)) {
robot.row = newRow;
robot.col = newCol;
}
}
// Function to initialize board with empty spaces
function initializeBoard() {
for (let i = 0; i < 5; i++) {
board[i] = [];
for (let j = 0; j < 5; j++) {
board[i][j] = undefined;
}
}
}
// Function to start the program
function start() {
initializeBoard();
rl.on('line', (input) => {
handleCommand(input);
rl.prompt();
});
rl.prompt();
}
rl.question('Enter a command: ', (input) => {
handleCommand(input);
rl.prompt();
});
Step by Step Solution
There are 3 Steps involved in it
Step: 1
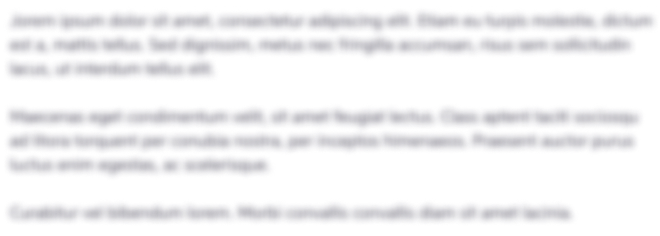
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started