Question
Can someone please help me on my C code. I have been working on this programming lab, but still stuck. I've appreciate it. The semester
Can someone please help me on my C code. I have been working on this programming lab, but still stuck. I've appreciate it.
The semester project Library Book Management System will be based on the data type struct book as defined below.
struct book{
int ID;
char Title[50];
char Author[50];
int SubjectCode;
int BorrowingAge;
char BorrowerName[50];
struct book *nextbook;
};
You will need to download 6 files they are prj.h, prj1.h, prj2.h, proj_main.c, prj1.c and library.c. The main is located in proj_main.c, it does not need to be modified. All the code you are writing will need to be placed in prj1.c. In library.c, I have written a number of functions. The prototypes for my functions are in prj2.h.
Each book that is in the library list will have a ID, Title, Author Name, Subject Code, Borrowing Age (reflects the number of days passed after it has been borrowed from the library), name of the Borrower and a pointer nextBook which points to a struct book.
Subject code for different subjects as follows:
000 General works, Computer science and Information, 100 Philosophy and psychology, 200 Religion, 300 Social sciences, 400 Language, 500 Pure Science, 600 Technology, 700 Arts & recreation, 800 Literature, 900 History & geography
The following functions will be available and written by the instructor:
void assignData(struct book *);
struct book * genNewBook(struct book *);
struct book * sortByID (struct book * head);
You are to write the following functions
struct book * processBook (struct book * head);
void displayList (struct book * head);
struct book * findBookbyID (struct book * head, int ID);
struct book * findBookbyKeywords (struct book * head, char * keywords);
struct book * addNewbook (struct book * head);
struct book * deleteBook (struct book * head, int ID_to_go);
void sendReminder (struct book * head);
struct book * addReturnedBook(struct book * head, int return_book_ID);
struct book * sortByAlphabeticalOrder (struct book * head);
void subjectSummary (struct book * head);
void terminateWrite (struct book * head);
struct book * freeList(struct book * head);
Main() will prompt the user to enter a command. The command menu looks like the following:
P Process Book D Display List A Add New Book L Delete Book
R Send Reminder
B Add Returned Book O Sort list by Alphabetical Order
T Sort list by ID
J Subject Summary Q Terminate
Functional descriptions of the functions you need to write:
struct book * processBook (struct book * head);
Here you are to process a book when a user wants to borrow it. User can ask for a book either by the ID or by keywords. Ask the user which method he/she wants to use then call your findBookbyID or findBookbyKeywords functions accordingly. If that book is found in the library then you issue that book to that user. Ask for the user name and it should get stored in the Borrower field. Borrowing Age (reflects the number of days it has been with the user) field should be changed from the default 0 value to 1. If the book is not found in the library or it has been already borrowed by someone else tell user that particular reason. Finally display your updated list.
void displayList (struct book * head);
Here you are to print to the screen each book in the library list in an organized and formatted manner. Remember to print a header for each element.
struct book * findBookbyID (struct book * head, int ID);
In this function you are passed an ID and you return the address of the book with this ID. If the book is not in the list tell user about it and you return NULL.
struct book * findBookbyKeywords (struct book * head,char * keywords);
In this function you are passed one or two words from a book title and you return the address of the book which has these words in its title. If no book with a title having the keywords is found tell user about it and you return NULL. (Hint: Check for substring)
struct book * addNewbook (struct book * head);
Here you are to use malloc() to generate a struct book and call assignData that will assign all the info for this new book. You should add the book into the link list. New books should go into the list so that the list is sorted according to ID.
struct book * deleteBook (struct book * head, int ID_to_go);
In this function you are passed an ID, find the node book_to_go from the link list that corresponds to that ID, then delete that node in a manner that reattaches the necessary links to preserve the link list, then you free the memory for book _to_go. If the ID is invalid then display a message to the user that it is an invalid ID and go back to the main menu.
void sendReminder (struct book * head);
A book can remain with the borrower for maximum 14 days. On 10th day the borrower should get a reminder message. In this function you will look for the books with borrowing age 10 and should display a reminder message to its borrower. For example your display window should print a message like this: Jerry lee, you borrowed the book C programming 10 days ago. You have 4 days left to return it.
struct book * addReturnedBook(struct book * head, int return_book_ID);
In this function you are passed the ID of a previously borrowed book. Find the node from the link list that corresponds to that ID. You are to erase the borrower name and set the borrowed age back to zero for that book.
struct book * sortByAlphabeticalOrder (struct book * head);
Here you are to sort the book list according to the Title field (in increasing alphabetical order). You should return a pointer to the beginning of the link list.
void subjectSummary (struct book * head);
In this function you are to count and display the number of books you have in the library for different subjects (do this using the subject codes). The books borrowed by the users should not get included in the count.
void terminateWrite (struct book * head);
If the user enter this command you are to write all the books in the list to a file called Booklist.txt. All fields of the structure for each book should be written to an individual line. After writing you should call freeList and then the program will terminate.
struct book * freeList(struct book * head);
Here you should free all the elements of your list and return NULL to main.
Individually how much each function will be worth:
processBook 15
displayList 10
findBookbyID 12
findBookbyTitle 12
addNewbook 12
deleteBook 12
sendReminder 10
addReturnedBook 10
sortByAlphabeticalOrder 15
subjectSummary 12
terminateWrite 10
freeList 10
Total 140
...................................................................................................................................
(1)library.c #define _CRT_SECURE_NO_WARNINGS #include "prj2.h" #include#include #include "prj1.h" char *author_source[]={"Horace Greeley", "Sigourney Weaver", "Wendy Morse", "Cora Simmons", "Phil Donahue", "Dan Crane", "Willie Daniels", "Carl Lewis","Joe Crabb", "Buster Keaton", "Dawn Harris", "Rene Williamson", "Pat Lane", "Jose Richards", "Edward Morris", "Nathan Nevins", "John Doe", "Jane Eyre", "Wednesday Adams", "Annette Wendt", "Yannick Noah", "Christopher Columbus", "George Sims", "Anna Washington", "Marie Curie", "Rhonda White", "Susan Nash", "Mary Wright", "Lily Langely","John Thompson", "Ray Reynolds"}; char *title_source[]={ "Angel Of Eternity", "Baker Of The Stockades", "Dogs Of The Nation", "Aliens Of Perfection", "Wives And Giants", "Enemies And Companions", "Annihilation Of Gold", "Obliteration Of Water", "Signs In My Dreams", "Healing The Abyss", "Defender Of Moondust", "Creature With A UFO", "Droids Of Sunshine", "Armies Of The Moon", "Invaders And Rebels", "Creatures And Emperors", "Planet Of Aliens", "Element Of Moondust", "Hidden In Robotic Control", "Confused By The Immortals", "Freaks Of The Decade", "Children Per Continent", "Animals Of The Revolution", "Crustaceans Of The Caves", "Rats And Spices", "Men And Friends", "Cars Of The Jungle", "Working Of The Future", "Ancient New Earth", "Political Aliens"}; char *borrower_source[]={"Farhad Akbar", "Bassam Awad Alanazi", "NONE", "NONE", "Roger Cungtha Biak", "NONE", "Christian Curtis Collier", "NONE","NONE", "Tanner Durnil", "Melissa Gudeman", "NONE", "NONE", "Daniel Patrick Kobold Jr", "Travis Lee Leondis", "Cleopatrio Soares Neto", "Daniel Biaklian Sang", "NONE", "Bryant Bernard", "NONE", "Joaquin John Garcia", "NONE", "NONE", "Jacob Austin Shebesh", "D'Artagnan S. Engle", "NONE", "You Zhou", "SYAMIL ALI", "NONE","Joi Ashlyn Officer", "NONE"}; char * code_source[]={"000", "100", "200", "300", "400", "500", "600", "700","800", "900" }; char * age_source[]={ "10","4", "10", "11", "12", "10", "2", "10", "6", "10", "1", "13", "3", "4", "6", "5", "3", "4", "6", "5", "10", "10", "10", "10","10","10", "9", "2", "8", "13"}; void assignData(struct book * V) { static int done=0; int titleStrings, authorStrings, borrowerStrings ; int i,j; if(!done) { done =1; srand(time(NULL)); } titleStrings=sizeof(title_source)/sizeof(char*); authorStrings=sizeof(author_source)/sizeof(char*); borrowerStrings=sizeof(borrower_source)/sizeof(char*); strcpy(V->Title, title_source[rand()%titleStrings]); strcpy(V->Author, author_source[rand()%authorStrings]); strcpy(V->BorrowerName, borrower_source[rand()%borrowerStrings]); V->ID = rand() % 9000 + 1000; if ((strcmp (V->BorrowerName, "NONE")) == 0) V->BorrowingAge = 0 ; else { j=rand()%12; V->BorrowingAge = atoi (age_source[j]); } j=rand()%10; V->SubjectCode = atoi (code_source[j]); V->nextbook=NULL; } struct book * genNewBook(struct book * H) { static int xv=1; int num; static int size= 4; struct book *head=NULL; struct book *ptr, *temp; int i; if(xv) { xv=0; srand(xv); } num = size + rand()%size; size=2; head=(struct book *) malloc(sizeof(struct book)); assignData(head); ptr=head; for(i=1;i nextbook=temp; ptr=temp; } ptr=head; head = sortByID(head); return head; } struct book * sortByID (struct book * head) { struct book * ptr=head; int num=0; int i; struct book *tmp, *stmp; while(ptr!=NULL) { num++; ptr=ptr->nextbook; } ptr=head; for(i=0; i nextbook !=NULL; ) { if(ptr->ID > ptr->nextbook->ID) { if(ptr==head) { head=ptr->nextbook; tmp=head->nextbook; head->nextbook=ptr; ptr->nextbook=tmp; } else { tmp=head; while(tmp->nextbook !=ptr) tmp=tmp->nextbook; stmp=ptr->nextbook->nextbook; tmp->nextbook=ptr->nextbook; ptr->nextbook->nextbook=ptr; ptr->nextbook=stmp; } } else ptr=ptr->nextbook; } } return head; }
................................................................................................................
(2)prj1.c
#define _CRT_SECURE_NO_WARNINGS
#include "prj1.h" #include "prj2.h" struct book * processBook (struct book * head) { return head; } void displayList (struct book * head) { } struct book * findBookbyID (struct book * head, int ID) { return NULL; } struct book * findBookbyKeywords(struct book * head, char * title) { return NULL; } struct book * addNewbook (struct book * head) { return head; } struct book * deleteBook (struct book * head, int ID_to_go) { return head; } void sendReminder (struct book * head) { } struct book * addReturnedBook(struct book * head, int return_book_ID) { return head; } struct book * sortByAlphabeticalOrder (struct book * head) { return head; } void subjectSummary (struct book * head) { } void terminateWrite (struct book * head) { } struct book * freeList(struct book * head) { return NULL; }
-------------------------------------------------------------------------------------
(3)prj1.h
#include "prj.h" struct book * processBook (struct book * head); void displayList (struct book * head); struct book * findBookbyID (struct book * head, int ID); struct book * findBookbyKeywords(struct book * head, char * keywords); struct book * addNewbook (struct book * head); struct book * deleteBook (struct book * head, int ID_to_go); void sendReminder (struct book * head); struct book * addReturnedBook(struct book * head, int return_book_ID); struct book * sortByAlphabeticalOrder (struct book * head); void subjectSummary (struct book * head); void terminateWrite (struct book * head); struct book * freeList(struct book * head);
-----------------------------------------------------------------------------------------------------------------------------------------------------------
(4)prj2.h
#include "prj.h" void assignData(struct book *); struct book * genNewBook(struct book *); struct book * sortByID (struct book * head);
-------------------------------------------------------------------------------------------------------------------------------------------------
(5)prj.h
#include#include #include #include #ifndef __PRJ__ #define __PRJ__ #define MAXD 20 struct book { int ID; char Title[50]; char Author[50]; int SubjectCode; int BorrowingAge; char BorrowerName[50]; struct book *nextbook; }; #endif
-----------------------------------------------------------------------------------------------------------------------------------------------
(6)proj_main.c
#define _CRT_SECURE_NO_WARNINGS #include "prj.h" #include "prj1.h" #include "prj2.h" #includevoid printMainMenu(); struct book * processBook (struct book * head); void displayList (struct book * head); struct book * findBookbyID (struct book * head, int ID); struct book * findBookbyKeywords(struct book * head, char * keywords); struct book * addNewbook (struct book * head); struct book * deleteBook (struct book * head, int ID_to_go); void sendReminder (struct book * head); struct book * addReturnedBook(struct book * head, int return_book_ID); struct book * sortByAlphabeticalOrder (struct book * head); void subjectSummary (struct book * head); void terminateWrite (struct book * head); struct book * freeList(struct book * head); int main() { struct book *head=NULL; char buffer[75]; char X; int ID_to_go; int return_book_ID; head= genNewBook (head); while(head !=NULL) { printMainMenu(); printf(">>>>> Enter a command "); X=getchar (); fflush(stdin); X=toupper(X); switch(X){ case 'P': // Process book displayList(head); head=processBook (head); fflush(stdin); break; case 'D': // Display list displayList(head); fflush(stdin); break; case 'A': // Add new book head=addNewbook (head); displayList(head); fflush(stdin); break; case 'L': // Delete book displayList(head); printf(">>>>> Choose a book ID "); ID_to_go= atoi(gets(buffer)); head=deleteBook (head, ID_to_go); displayList(head); fflush(stdin); break; case 'R': // Send reminder displayList(head); sendReminder(head); fflush(stdin); break; case 'B': // Add returned book displayList(head); printf(">>>>> Choose a book ID "); return_book_ID= atoi(gets(buffer)); head=addReturnedBook (head, return_book_ID); displayList(head); fflush(stdin); break; case 'T': // Sort list by ID //completed by JG head=sortByID(head); displayList(head); fflush(stdin); break; case 'O': // Sort list by alphabetical order head=sortByAlphabeticalOrder (head); displayList(head); fflush(stdin); break; case 'J': // Subject summary displayList(head); subjectSummary (head); fflush(stdin); break; case 'Q': // Terminate terminateWrite (head); head = freeList(head); fflush(stdin); break; } } return 0; } void printMainMenu() { printf("******************************************************* "); printf("******************************************************* "); printf(" Main Menu "); printf("******************************************************* "); printf("\tP\t\tProcess Book "); printf("\tD\t\tDisplay List "); printf("\tA\t\tAdd New Book "); printf("\tL\t\tDelete Book "); printf("\tR\t\tSend Reminder "); printf("\tB\t\tAdd Returned Book "); printf("\tO\t\tSort list by Alphabetical Order "); printf("\tJ\t\tSubject Summary "); printf("\tT\t\tSort list by ID "); printf("\tQ\t\tTerminate "); printf("******************************************************* "); printf("******************************************************* "); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
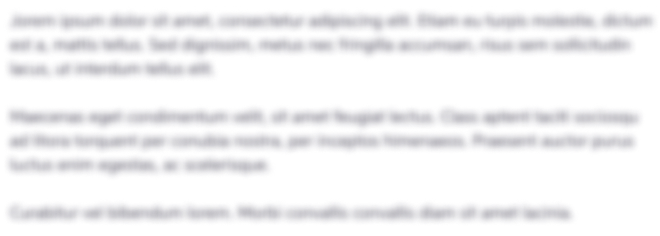
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started