Question
Can someone please help me? Please explain in /*comments*/. THANKS IN ADVANCE!!!! #include #include #include #include // included to check for memory leaks // #define
Can someone please help me? Please explain in /*comments*/. THANKS IN ADVANCE!!!!
#include
#include
#include
#include
// included to check for memory leaks
// #define CRTDBG_MAP_ALLOC // for Visual Studio Only
// #include
// #pragma warning(disable: 4996) // for Visual Studio Only
// global linked list 'list' contains the list of books
struct bookList {
struct book *book;
struct bookList *next;
} *list = NULL; // currently empty
// structure "book" contains the book's name, library's name and linked list of authors
struct book {
char name[30];
char library[30];
struct author *authors;
};
// linked list 'authors' contains name of authors
struct author {
char name[30];
struct author *next;
};
// forward declaration of functions that have already been implemented
// Do not modify these functions
void flushStdIn();
void actionOnList(char);
void helper(char);
void deleteDatabase(struct bookList*);
void displayList(struct bookList*);
// The following forward declarations are for functions that require implementation. Their empty definition is at the bottom of the file.
// Do not modify the return types and input parameter data types
// return type // name and parameters // points
void addBook(char*, char*); // 5
void addAuthor(char*, char*); // 10
struct book* searchBook(char*); // 5
void removeBook(char*); // 15
char* lastAuthor(char*); // 15
struct bookList* booksInThisLibrary(char*); // 25
struct bookList* sortByName(); // 25
// Total: 100 points
int main()
{
char ch = '\0';
printf(" CSE 220 project 5: Library database ");
do
{
printf(" Please select an option: ");
printf("\t a: Add a new book to the database ");
printf("\t c: Add an author for a book ");
printf("\t s: Search for a book in the database ");
printf("\t r: Remove a book from the database ");
printf("\t l: Display the last author added for a book ");
printf("\t b: Display the list of books available in a particular library ");
printf("\t n: Display the list of books sorted by name ");
printf("\t e: Exit ");
ch = tolower(getchar());
flushStdIn();
actionOnList(ch);
} while (ch != 'e');
deleteDatabase(list);
list = NULL;
// _CrtDumpMemoryLeaks(); // check for memory leaks (VS will let you know in output if they exist)
return 0;
}
// flush out ' ' characters
void flushStdIn()
{
int c;
do
c = getchar();
while (c != ' ' && c != EOF);
}
// branch to different tasks
void actionOnList(char c)
{
switch (c)
{
case 'a':
case 'c':
case 's':
case 'r':
case 'l':
case 'b':
case 'n': helper(c); break;
case 'e': break;
default: printf("Invalid input! ");
}
}
// This function asks the user for information depending on what option the user selects from the menu and displays the expected result.
// This function is already implemented completely. Read the function to understand how and what parameters are given as input to the functions that you will implement.
// It is always helpful to understand how the code works before implementing new features.
// Do not change anything in this function or you risk failing the automated test cases.
void helper(char c)
{
if (c == 'a')
{
char bookName[100];
char libraryOfBook[30];
printf(" Please enter the following info of the new book. ");
printf(" Book name: ");
fgets(bookName, sizeof(bookName), stdin);
bookName[strlen(bookName) - 1] = '\0'; // remove the trailing ' '
printf(" Library: ");
fgets(libraryOfBook, sizeof(libraryOfBook), stdin);
libraryOfBook[strlen(libraryOfBook) - 1] = '\0'; // remove the trailing ' '
struct book* result = searchBook(bookName);
if (result == NULL) // add book if not already present in database
{
addBook(bookName, libraryOfBook);
printf(" Book added to library database. ");
}
else
printf(" That book is already in the database. ");
}
else if (c == 's' || c == 'r' || c == 'c' || c == 'l')
{
// actions which are common to commands s,r,c,l
char bookName[30];
printf(" Please enter the book's name: ");
fgets(bookName, sizeof(bookName), stdin);
bookName[strlen(bookName) - 1] = '\0'; // remove the trailing ' '
struct book* result = searchBook(bookName);
if (result == NULL)
printf(" That book is not in the database. ");
else if (c == 's')
{
printf(" Library: %s ", result->library);
if (result->authors == NULL)
printf("No author in database for this book. ");
else
{
printf(" Authors: ");
// modified code start
struct author* tempAuthor = result->authors;
while (tempAuthor != NULL)
{
printf("%s ", tempAuthor->name);
tempAuthor = tempAuthor->next;
}
/* while(result->authors != NULL)
{
printf("%s ", result->authors->name);
result->authors = result->authors->next;
}
*/
// modified code end
}
}
// command specific actions below
else if (c == 'r')
{
removeBook(bookName);
printf(" Book removed from the library database ");
}
else if (c == 'c')
{
char authorName[30];
printf(" Please enter the name of the author: ");
fgets(authorName, sizeof(authorName), stdin);
authorName[strlen(authorName) - 1] = '\0'; // remove the trailing ' '
addAuthor(bookName, authorName);
printf(" Author added. ");
}
else if (c == 'l')
{
char* result = lastAuthor(bookName);
if (result == NULL)
printf(" No authors listed for this book. ");
else
printf(" Last author: %s ", result);
}
}
else if (c == 'b')
{
char libraryName[30];
printf(" Please enter the library name: ");
fgets(libraryName, sizeof(libraryName), stdin);
libraryName[strlen(libraryName) - 1] = '\0'; // remove the trailing '
struct bookList* result = booksInThisLibrary(libraryName);
printf(" List of books in %s library: ", libraryName);
displayList(result);
deleteDatabase(result);
result = NULL;
}
else // c = 'n'
{
struct bookList* result = sortByName();
printf(" List of books sorted by name: ");
displayList(result);
deleteDatabase(result);
result = NULL;
}
}
// This function recursively removes all books from the linked list.
// Notice that all of the authors for all of the books are removed as well
// This function is already called at the right places.
void deleteDatabase(struct bookList* books)
{
struct author* a1;
if (books != NULL)
{
deleteDatabase(books->next);
while (books->book->authors != NULL)
{
a1 = books->book->authors;
books->book->authors = books->book->authors->next;
free(a1); // delete authors linked list
}
free(books->book);
free(books); // delete book linked list
}
}
// This function prints the list of books for 'n: sort by name' and 'b: books in particular library' options.
// It may be useful to trace this code before you get started
void displayList(struct bookList* inputList)
{
if (inputList == NULL)
{
printf(" No books to list ! "); // check if list is empty
return;
}
while (inputList != NULL) // traverse the list to display
{
printf(" Book name: %s", inputList->book->name);
printf(" Library: %s ", inputList->book->library);
printf("Authors: ");
struct author* ptr = inputList->book->authors;
if (ptr == NULL) // check if authors are added for the book
{
printf("No authors in database. ");
}
else
{
while (ptr != NULL) // traverse list of authors to display
{
printf("%s ", ptr->name);
ptr = ptr->next;
}
}
inputList = inputList->next;
}
}
Please read instructions carefully (under Project Description). The codes that I pasted on here is what the professor provided. I need to add two void functions (void addBook and void addAuthor). Please help and explain. THANKS!
Project Description The following figure shows an instance of the linked list that is used in this project. The main node of the linked list is called bookList, which contains two members: 'book' and 'next'. The 'book' member is a pointer to a book node. The 'next' pointer points to the next book. The book node contains three members: name, library and authors. The 'authors' member is a pointer to author node. The 'author' node contains the author's name and a 'next' pointer, which points to next author node. The last 'next' pointer of all linked lists has to be terminated with NULL list bookList *book book *book next *next next null book name name name ibrary library authors author library authors authors null name name name next *next null next null You are given a partially completed program p05.c. Your job is to follow the instructions given in the C file to complete the functions so that the program executes properly. A user is given the option to perform few actions on the list/database. You will need to write the functions which implement these actions. The functions that are already implemented in the program take care of asking the user for the information that is needed to add to perform the action, displaying the result of the actions and calling the functions at appropriate places in the programStep by Step Solution
There are 3 Steps involved in it
Step: 1
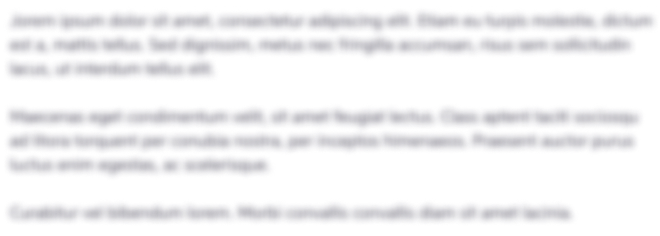
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started