Question
can you add a method to this code that checks if each integer input by user is zero or not so that the output includes
can you add a method to this code that checks if each integer input by user is zero or not so that the output includes a line like so:
import java.io.*;
import java.lang.*;
import java.util.Arrays;
class HugeInteger{
private int ArrayDifference[]=new int[40];
private int AddRes[]=new int[40];
private int SubRes[]=new int[40];
private boolean greaterThan;
private boolean lessThan;
private boolean equalTo;
public HugeInteger(){
}
public HugeInteger(int[] arrayDiff) {
ArrayDifference = arrayDiff;
}
public HugeInteger(boolean isLess, boolean isGreater, boolean isEqual) {
this.lessThan = isLess;
this.greaterThan = isGreater;
this.equalTo = isEqual;
}
public HugeInteger(int[] addRes, int[] subRes) {
AddRes = addRes;
SubRes = subRes;
}
public boolean isLess() {
return lessThan;
}
public boolean isGreater() {
return greaterThan;
}
public boolean isEqual() {
return equalTo;
}
public int[] getArrayDiff() {
return ArrayDifference;
}
public int[] getAddRes() {
return AddRes;
}
public int[] getSubRes() {
return SubRes;
}
public void setLess(boolean isLess) {
this.lessThan = isLess;
}
public void setGreater(boolean isGreater) {
this.greaterThan = isGreater;
}
public void setEqual(boolean isEqual) {
this.equalTo = isEqual;
}
public void setArrayDiff(int[] arrayDiff) {
ArrayDifference = arrayDiff;
}
public void setAddRes(int[] addRes) {
AddRes = addRes;
}
public void setSubRes(int[] subRes) {
SubRes = subRes;
}
public boolean EqualTo(int a[],int b[]){
equalTo=Arrays.equals(a,b);
return equalTo;
}
public boolean NotEqualTo(int a[],int b[]){
return (!Arrays.equals(a,b));
}
public boolean GreaterThan(int a[],int b[]){
for(int i=0;i
ArrayDifference[i]=a[i]-b[i];
}
for(int i=0;i
if(ArrayDifference[i]>0){
greaterThan=true;
return true;
}else if(ArrayDifference[i]==0){
continue;
}
else{
lessThan=true;
return false;
}
}
return false;
}
public boolean LessThan(int a[],int b[]){
for(int i=0;i
ArrayDifference[i]=a[i]-b[i];
}
for(int i=0;i
if(ArrayDifference[i]
lessThan=true;
return true;
}else if(ArrayDifference[i]==0){
continue;
}
else{
greaterThan=true;
return false;
}
}
return false;
}
public boolean GreaterThanOrEqualTo(int a[],int b[]){
if(greaterThan || equalTo)
return true;
else
return false;
}
public boolean LessThanOrEqualTo(int a[],int b[]){
if(lessThan || equalTo)
return true;
else
return false;
}
public String Add(int a[],int b[]){
int carry=0;
String str="";
for(int i=39;i>=0;i--){
AddRes[i]=(a[i]+b[i]+carry)%10;
carry=(a[i]+b[i])/10;
}
for(int i=0;i
str+=AddRes[i]+"";
return str;
}
public String Sub(int a[],int b[]){
String str="";
if(greaterThan){
int borrow=0;
str="";
for(int i=39;i>=0;i--){
if(a[i]
borrow=10;
a[i-1]--;
}
else {
borrow=0;
}
SubRes[i]=a[i]-b[i]+borrow;
}
}else{
int borrow=0;
str="-";
for(int i=39;i>=0;i--){
Step by Step Solution
There are 3 Steps involved in it
Step: 1
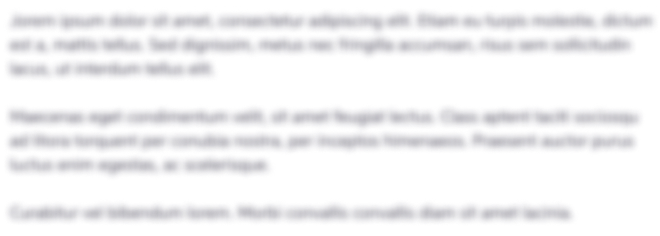
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started