Question
Can you add some comments to this code describing each part and how it works? Thanks public class ItemToPurchase { private String itemName; private int
Can you add some comments to this code describing each part and how it works? Thanks
public class ItemToPurchase {
private String itemName;
private int itemPrice;
private int itemQuantity;
public ItemToPurchase() {
itemName = "none";
itemPrice = 0;
itemQuantity = 0;
}
public String getName() {
return itemName;
}
public void setName(String itemName) {
this.itemName = itemName;
}
public int getPrice() {
return itemPrice;
}
public void setPrice(int itemPrice) {
this.itemPrice = itemPrice;
}
public int getQuantity() {
return itemQuantity;
}
public void setQuantity(int itemQuantity) {
this.itemQuantity = itemQuantity;
}
}
____________________________________________________________________________________________________________________
import java.util.Scanner;
public class ShoppingCartPrinter {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
ItemToPurchase item1 = new ItemToPurchase();
ItemToPurchase item2 = new ItemToPurchase();
System.out.println("Item 1");
System.out.println("Enter the item name:");
String name1 = scan.nextLine();
System.out.println("Enter the item price:");
int price1 = scan.nextInt();
System.out.println("Enter the item quantity:");
int quantity1 = scan.nextInt();
item1.setName(name1);
item1.setPrice(price1);
item1.setQuantity(quantity1);
scan.nextLine();
System.out.println();
System.out.println("Item 2");
System.out.println("Enter the item name:");
String name2 = scan.nextLine();
System.out.println("Enter the item price:");
int price2 = scan.nextInt();
System.out.println("Enter the item quantity:");
System.out.println();
int quantity2 = scan.nextInt();
item2.setName(name2);
item2.setPrice(price2);
item2.setQuantity(quantity2);
System.out.println("TOTAL COST");
int item1Total = item1.getPrice() * item1.getQuantity();
int item2Total = item2.getPrice() * item2.getQuantity();
System.out.println(item1.getName()+" "+item1.getQuantity()+" @ $"+item1.getPrice()+" = $"+item1Total);
System.out.println(item2.getName()+" "+item2.getQuantity()+" @ $"+item2.getPrice()+" = $"+item2Total);
System.out.println();
System.out.println("Total: $"+(item1Total + item2Total));
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
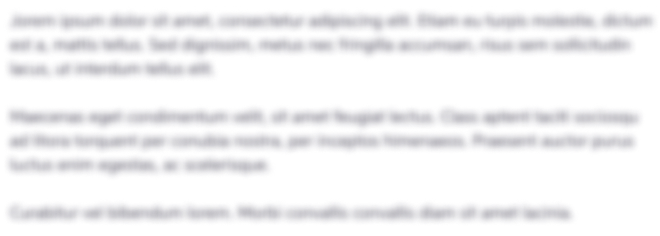
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started