Question
// Can you comment on what gets added? It would really help. package option1.stage3; import option1.stage1.Doctor; import option1.stage1.Patient; import option1.stage2.TimeSlot; public class Appointment { private
// Can you comment on what gets added? It would really help.
package option1.stage3;
import option1.stage1.Doctor;
import option1.stage1.Patient;
import option1.stage2.TimeSlot;
public class Appointment {
private Doctor doctor;
private Patient patient;
private TimeSlot timeSlot;
public Doctor getDoctor() {
return doctor;
}
public void setDoctor(Doctor doctor) {
this.doctor = doctor;
}
public Patient getPatient() {
return patient;
}
public void setPatient(Patient patient) {
this.patient = patient;
}
public TimeSlot getTimeSlot() {
return timeSlot;
}
public void setTimeSlot(TimeSlot timeSlot) {
this.timeSlot = timeSlot;
}
public Appointment(Doctor doctor, Patient patient, TimeSlot timeSlot) {
setDoctor(doctor);
setPatient(patient);
setTimeSlot(timeSlot);
}
public Appointment(Patient patient, TimeSlot timeSlot) {
setDoctor(null);
setPatient(patient);
setTimeSlot(timeSlot);
}
public String toString() {
if(doctor != null)
return patient+" with "+doctor+" for "+timeSlot;
else
return patient+" for "+timeSlot;
}
/**
*
* @param other
* @return true if calling object conflicts with parameter Appointment.
* we say two appointments conflict if they are with the same doctor
* and the timeSlots overlap.
* HINT: Use method equals from Doctor class and method overlapsWith from TimeSlot class
*/
public boolean conflictsWith(Appointment other) {
return false; //TO BE COMPLETED
}
}
//THE APPOINTMENTTEST.JAVA PART:
package option1.stage3;
import common.Graded;
import option1.stage1.*;
import option1.stage2.TimeSlot;
import static org.junit.Assert.*;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Date;
import java.text.SimpleDateFormat;
import org.junit.AfterClass;
import org.junit.Test;
public class AppointmentTest {
private static int score = 0;
private static String result = "";
@Test @Graded(marks=5, description="Appointment:conflictsWith(Appointment)")
public void testConflictsWith() {
Doctor d1 = new Doctor("Rachel", "Pediatrician");
Doctor d2 = new Doctor("James", "Oncologist");
Doctor d3 = new Doctor("Rachel", "General Practitioner");
Doctor d5 = new Doctor("rachel", "pediatrician");
Time a = new Time(12, 0);
Time b = new Time(12, 15);
Time c = new Time(12, 30);
Time d = new Time(12, 45);
Time e = new Time(13, 0);
TimeSlot slot1 = new TimeSlot(a, b);
TimeSlot slot3 = new TimeSlot(c, d);
TimeSlot slot4 = new TimeSlot(b, d);
TimeSlot slot7 = new TimeSlot(a, e);
Patient randomPatient = new Patient("Dummy");
Appointment a1 = new Appointment(d1, randomPatient , slot1);
Appointment a2 = new Appointment(d1, randomPatient , slot3);
assertFalse(a1.conflictsWith(a2));
Appointment a3 = new Appointment(d2, randomPatient , slot1);
assertFalse(a1.conflictsWith(a3));
Appointment a4 = new Appointment(d1, randomPatient , slot7);
assertTrue(a2.conflictsWith(a4));
Appointment a5 = new Appointment(d5, randomPatient , slot4);
assertFalse(a1.conflictsWith(a5));
Appointment a6 = new Appointment(d3, randomPatient , slot1);
assertFalse(a1.conflictsWith(a6));
Appointment a7 = new Appointment(d5, randomPatient , slot7);
assertTrue(a1.conflictsWith(a7));
score+=5;
result+="Appointment:conflictsWith(Appointment) passed ";
}
//log reporting
@AfterClass
public static void wrapUp() throws IOException {
System.out.println(result.substring(0,result.length()-1));
System.out.println("--------------------------------------------");
System.out.println("Total: "+score+" out of 5");
System.out.println("-------------------------------------------- ");
String timeStamp = new SimpleDateFormat("yyyy-MM-dd-HH-mm-ss").format(new Date());
File file = new File("log/Appointment"+timeStamp+".txt");
FileWriter writer = new FileWriter(file);
writer.write(result.substring(0,result.length()-1)+" ");
writer.write("-------------------------------------------- ");
writer.write(score+" ");
writer.flush();
writer.close();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
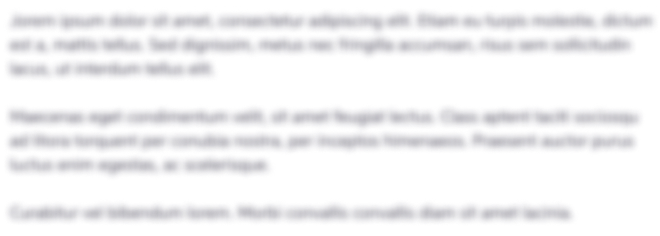
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started