Question
Can you convert this code into a C++ code? //////////////////////////////////////////// DVD.java package movie; public class DVD { private String movieTitle; private String movieStars[]; private String
Can you convert this code into a C++ code?
////////////////////////////////////////////
DVD.java
package movie;
public class DVD {
private String movieTitle; private String movieStars[]; private String movieDirector; private String movieProducer; private String movieProductionCo; private int numberOfCopies; DVD(String title, String[] stars, String director, String producer, String production, int copies) { movieTitle = title; movieStars = stars; movieDirector = director; movieProducer = producer; movieProductionCo = production; numberOfCopies = copies; }
public String getMovieTitle() { return movieTitle; }
public void setMovieTitle(String movieTitle) { this.movieTitle = movieTitle; }
public String[] getMovieStars() { return movieStars; }
public void setMovieStars(String[] movieStars) { this.movieStars = movieStars; }
public String getMovieDirector() { return movieDirector; }
public void setMovieDirector(String movieDirector) { this.movieDirector = movieDirector; }
public String getMovieProducer() { return movieProducer; }
public void setMovieProducer(String movieProducer) { this.movieProducer = movieProducer; }
public String getMovieProductionCo() { return movieProductionCo; }
public void setMovieProductionCo(String movieProductionCo) { this.movieProductionCo = movieProductionCo; }
public int getNumberOfCopies() { return numberOfCopies; }
public void setNumberOfCopies(int numberOfCopies) { this.numberOfCopies = numberOfCopies; } public void incrementCopies() { numberOfCopies++; } public boolean checkOut() { if(numberOfCopies>0) { numberOfCopies--; return true; } else return false; } public void checkIn() { numberOfCopies++; } @Override public String toString() { return "Movie: " + movieTitle + ", Stars: " + movieStars[0] + " and " + movieStars[1] + ", Director: " + movieDirector + ", Producer: " + movieProducer + ", Production Company: "+movieProductionCo; } }
PersonType.java
package movie;
public class PersonType { private String firstName; private String lastName; PersonType(String firstName, String lastName) { this.firstName = firstName; this.lastName = lastName; }
public String getFirstName() { return firstName; }
public void setFirstName(String firstName) { this.firstName = firstName; }
public String getLastName() { return lastName; }
public void setLastName(String lastName) { this.lastName = lastName; } }
Customer.java
package movie;
import java.util.ArrayList; import java.util.List;
public class Customer extends PersonType{ List
public int getAccountNumber() { return accountNumber; } public String getName() { return getFirstName() + " " + getLastName(); } public void setName(String firstName, String lastName) { setFirstName(firstName); setLastName(lastName); } public void rentDVD(DVD dvd) { for(int i=0; i
DVDStore.java
package movie;
import java.util.ArrayList; import java.util.List;
public class DVDStore { List DVDDriver.java package movie; import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class DVDDriver { public static void menu(DVDStore store) { System.out.println("1. Search a DVD"); System.out.println("2. Check out a DVD"); System.out.println("3. Check in a DVD"); System.out.println("4. Check whether a DVD is in stock"); System.out.println("5. Print all titles of DVD"); System.out.println("6. Print all the DvDs"); System.out.println("9. Exit"); System.out.println("Enter your choice: "); Scanner consoleInt = new Scanner(System.in); Scanner consoleString = new Scanner(System.in); int choice = consoleInt.nextInt(); int accNumber; DVD dvd = null; String title = ""; if(choice != 9 && choice != 5 && choice != 6) { System.out.println("Enter the movie title: "); title = consoleString.nextLine(); } switch(choice) { case 1: dvd = store.searchDVD(title); if(dvd != null) { System.out.println(dvd); } menu(store); break; case 2: System.out.println("Enter account number of Customer: "); accNumber = consoleInt.nextInt(); if(store.rentDVD(title, accNumber)) System.out.println("Movie rented !"); menu(store); break; case 3: System.out.println("Enter account number of Customer: "); accNumber = consoleInt.nextInt(); store.returnDVD(title, accNumber); System.out.println("Movie returned !"); menu(store); break; case 4: dvd = store.searchDVD(title); if(dvd != null) { if(dvd.getNumberOfCopies()>0) System.out.println(title + " is in stock"); } menu(store); break; case 5:store.printMovieTitles(); menu(store); break; case 6: store.printAllDVDs(); menu(store); break; case 9: return; } } public static void main(String[] args) { DVDStore store = new DVDStore(); try { Scanner filereader = new Scanner(new File("movies.txt")); while(filereader.hasNextLine()) { String title = filereader.nextLine().replace("DVD ", ""); String star1 = filereader.nextLine().replace("movie ", ""); String star2 = filereader.nextLine().replace("movie ", ""); String producer = filereader.nextLine().replace("movie ", ""); String director = filereader.nextLine().replace("movie ", ""); String production = filereader.nextLine().replace("movie ", ""); String copies = filereader.nextLine(); store.addDVD(new DVD(title, new String[]{star1, star2}, director, producer, production, Integer.valueOf(copies))); } } catch (FileNotFoundException e) { System.out.println("File format is wrong !"); e.printStackTrace(); } System.out.println("Enter a customer first name: "); Scanner consoleReader = new Scanner(System.in); String fName = consoleReader.next(); System.out.println("Enter a customer last name: "); String lname = consoleReader.next(); Customer customer = new Customer(fName, lname); store.addCustomer(customer); menu(store); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
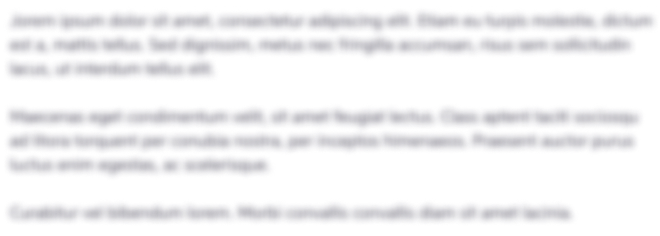
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started