Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Can you help me fix these bugs in my PlayerTest.java class so it compiles?: (I commented HELP next to errors in code) I think that
Can you help me fix these bugs in my PlayerTest.java class so it compiles?: (I commented HELP next to errors in code)
I think that when I created an object it has the wrong parameters.
it uses file data as input
data.txt
2 G Hugh Hefner,Rocky Bayou Country Club Bluewater Country Club,75,10/23/2013,70.5,113 Rocky Bayou Country Club,82,01/21/2014,68.5,123 3 B Dan Bilzerian,Ignite Felton Lanes, 112,09/22/2012 Bowlarama, 142,09/24/2012 Cordova Lanes,203,09/24/2012 2 G Tiger Woods,Isleworth Augusta,68,04/08/2013,76.2,135 Bethpage Black,71,06/21/2012,76.6,144 3 B Earl Anthony,Nine Mile Denny's Firestone Lanes, 223,09/22/1968 Bowlarama, 246,09/24/1976 Cordova Lanes,210,09/24/1977
PlayerTest.java
import java.util.Scanner; import java.util.ArrayList; import java.io.FileNotFoundException; import java.io.FileInputStream; public class PlayerTest { public static void main(String[] args) { ArrayList aListOfPlayers = new ArrayList(); Scanner fileIn = null; try{ FileInputStream file = new FileInputStream("data.txt"); fileIn = new Scanner(file); int numOfScores = 0; String typeScore = ""; String playerCourse = ""; String scoreData = ""; String player = ""; String course = ""; while (fileIn.hasNextLine()) { numOfScores = Integer.parseInt(fileIn.nextLine()); typeScore = fileIn.nextLine().toString(); playerCourse = fileIn.nextLine().toString(); String delims = "[,]"; String[] arrPlayerCourse = playerCourse.split(delims); System.out.println("The scores are: "); String[] arrScoreParts; if (typeScore.contains("G")) { Golfer myAutoGolfer = new Golfer(arrPlayerCourse[0].trim().toString(), arrPlayerCourse[1].trim().toString()); //HELP for (int i = 0; i < numOfScores; i++) { scoreData = fileIn.nextLine().toString(); arrScoreParts = scoreData.split(delims); myAutoGolfer.addScore(Integer.parseInt(arrScoreParts[1].trim().toString()), arrScoreParts[2].trim().toString(), arrScoreParts[0].trim().toString(), Double.parseDouble(arrScoreParts[3].trim().toString()), Integer.parseInt(arrScoreParts[4].trim().toString())); } if (numOfScores >= 5) { System.out.println(myAutoGolfer.calculateHandicap()); } aListOfPlayers.add((Player) myAutoGolfer); } if (typeScore.contains("B")) { Bowler myBowler = new Bowler(arrPlayerCourse[0], arrPlayerCourse[1]); //HELP for (int i = 0; i < numOfScores; i++) { scoreData = fileIn.nextLine(); arrScoreParts = scoreData.split(delims); BowlerScore myBowlerScore = new BowlerScore(Integer.parseInt(arrScoreParts[1].trim().toString()), arrScoreParts[2].trim(), arrScoreParts[0].trim()); myBowler.addScore(myBowlerScore); } if (numOfScores >= 5) { System.out.println(myBowler.calculateHandicap()); } aListOfPlayers.add((Player) myBowler); } for (Player aPlayer : aListOfPlayers) { System.out.println(aPlayer.toString()); } } }catch (FileNotFoundException e){ System.out.println("File not found."); System.exit(0); }finally { fileIn.close(); } } }
Golfer.java
import java.lang.String; public abstract class Golfer extends Player { String homeCourse; int numScores; double handicap; Score scores[]; int NUM_SCORES = 10;//assume no more than 10 score Course course; Score score; public Golfer() { super(); scores = new Score[NUM_SCORES]; homeCourse =""; } public Golfer(String name, int IDNum, String homeCourse, int numScores) { super(name, IDNum); scores = new Score[NUM_SCORES]; this.homeCourse = homeCourse; this.NUM_SCORES = numScores; } public String getHomeCourse() { return homeCourse; } public void setHomeCourse(String homeCourse) { this.homeCourse = homeCourse; } public int getNumScores() { return NUM_SCORES; } public void setNumScores(int numScores) { this.NUM_SCORES = numScores; } public double calculateHandicap(Course course) { double rate = course.getCourseRating(); int slope = course.getCourseSlope(); for(int i = 0; i>NUM_SCORES; i++) { handicap += (scores[i].score-rate)*(113/slope); } return handicap; } public void addScore(int fScore, String fDate, String courseName, double fCourseRating, int fCourseSlope) { course = new Course(courseName, fCourseRating, fCourseSlope); score = new Score(fScore, fDate, course); int counter = 0; for (int i = 0; i < scores.length; i ++) { if (scores[i] != null) { counter ++; scores[counter] = score; } } } public boolean deleteScore(String date) { boolean available = false; for (int i = 0; i < scores.length; i++) { if (scores[i].getDate().equals(date)) { available = true; scores[i] = null; } } return available; } public Score getScores(String date) { Score score = null; for (int i = 0; i < scores.length; i++) { if (scores[i].getDate().equals(date)) { score = scores[i]; } } return score; } public int findScore(String findDate) { final int NOT_FOUND = -1; for (int i = 0; i < scores.length; i++) { if (scores[i].getDate().equals(findDate)) { return i; } } return NOT_FOUND; } @Override public String toString() { return name+ " " + "ID Number: " + IDNum + "Home Course: " + homeCourse + "Handicap: " + handicap; } }
Bowler.java
import java.util.ArrayList; public class Bowler extends Player{ String teamName; private BowlerScore[] bowlerScore; int numScore; //# objects in array static int nextIDNum = 1000; private String homeLane; ArrayList scores = new ArrayList(); public Bowler() { super(); teamName = ""; } public Bowler(String teamName, BowlerScore[] bowlerScore, int numScore) { this.teamName = teamName; this.numScore = numScore; this.IDNum = getIDNum(); this.setNextIDNum(numScore); bowlerScore = new BowlerScore[20]; } public double calculateHandicap() { int sumScores = 0; int flag = 0; int lastBowler = 0; int counter = 0; for (int i = 0; i < bowlerScore.length; i ++) if (bowlerScore[i] != null) counter ++; for (int i = counter; i > 0; i--){ if( bowlerScore[i] != null ) { if ( flag < 5 && bowlerScore[i].getScore() != 9999 ) { sumScores += bowlerScore[i].getScore(); flag++; lastBowler = i; } } } double average = sumScores / 5; double diff; double handicap; diff = 200 - average; handicap = 0.8 * diff; if ( lastBowler != 0 ) { bowlerScore[lastBowler + 1].setScore(bowlerScore[lastBowler + 1].getScore() + (int)handicap); } return handicap; } public String getTeamName() { return teamName; } public void setTeamName(String teamName) { this.teamName = teamName; } public BowlerScore[] getBowlerScore() { return bowlerScore; } public void setBowlerScore(BowlerScore[] bowlerScore) { this.bowlerScore = bowlerScore; //help } public int getNumScore() { return numScore; } public void setNumScore(int numScore) { this.numScore = numScore; } public void addScore(BowlerScore theBowlerScore) { int counter = 0; for (int i = 0; i < bowlerScore.length; i++) { if (bowlerScore[i] != null) { counter ++; bowlerScore[counter] = theBowlerScore; } } } public String toString() { int counter = 0; int scoreError = 0; int dateError = 0; int error= 0; String getAllScores = ""; for (int i = 0; i < bowlerScore.length; i++) { if (bowlerScore[i] != null) { counter ++; } for ( int j =0; j < counter; j++ ){ if( bowlerScore[j] != null ) { getAllScores = getAllScores + bowlerScore[j]; if( bowlerScore[j].getScore() == 9999 ){ if( scoreError == 0 ){ error = 1; } scoreError++; }else if( bowlerScore[j].getDate().equals("INVALID") ){ if( dateError == 0 ) { error = 1; } dateError++; } } } if(error == 0) { //no error return getName() + " ID number: " + getIDNum() + " Team Name: " + getTeamName() + " Current Handicap:" + calculateHandicap() + " " + "Score Date Lane " + getAllScores; }else{//input has error if( scoreError != 0 ) { //score input error return getName() + " ID number: " + getIDNum() + " Team Name: " + getTeamName() + " Current Handicap:" + calculateHandicap() + " " + "Score Date Lane " + getAllScores + " "+ "There is an ERROR with your score."; }else{ //date input error return getName() + " ID number: " + getIDNum() + " Team Name: " + getTeamName() + " Current Handicap:" + calculateHandicap() + " " + "Score Date Lane " + getAllScores + " "+ "There is an ERROR with your date."; } } } return getAllScores; } }
BowlerScore.java
import java.util.GregorianCalendar; public class BowlerScore { String laneName; int score; String date; public BowlerScore() { super(); } public BowlerScore(int score, String laneName, String date) { super(); this.laneName = laneName; this.score = score; this.date = date; } public String getLaneName() { return laneName; } public void setLaneName(String laneName) { this.laneName = laneName; } public int getScore() { return score; } public void setScore(int fscore) { if( fscore >= 0 && fscore <= 300 ) { score = fscore; }else{ score = 9999; } } public String getDate() { return date; } public void setDate(String fdate) { GregorianCalendar calendar = new GregorianCalendar(); calendar.setLenient(false); String[] splitDate = fdate.split("/"); int month = Integer.parseInt( splitDate[0] ); int days = Integer.parseInt( splitDate[1] ); int year = Integer.parseInt( splitDate[2] ); calendar.set(year, month, days); try{ calendar.getTime(); }catch (Exception e){ fdate = "INVALID"; } date = fdate; } public String toString() { if ( getScore() == 9999 && !getDate().equals("INVALID") ){ return getScore() + " " + getDate() + " " + getLaneName() + " "; }else if ( getDate().equals("INVALID") && getScore() != 9999 ){ return getScore() + " " + getDate() + " " + getLaneName() + " "; }else if ( getScore() == 9999 && getDate().equals("INVALID") ){ return getScore() + " " + getDate() + " " + getLaneName() + " "; } return getScore() + " " + getDate() + " " + getLaneName() + " "; } }
Player.java
public abstract class Player { String name; int IDNum; static int nextIDNum = 1000; public Player() { super(); name =""; IDNum = 0; } public Player(String name, int iDNum) { super(); this.name = name; this.IDNum = iDNum; } public String getName() { return name; } public void setName(String fName) { this.name = fName; } public int getIDNum() { return IDNum; } public void setIDNum(int iDNum) { IDNum = getNextIDNum()+1; setNextIDNum(getIDNum()); } public static int getNextIDNum() { return nextIDNum; } public void setNextIDNum(int nextIDNum) { Player.nextIDNum = nextIDNum; } public abstract double calculateHandicap(); //represents # of handicap players }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Lets identify and fix the issues in your PlayerTestjava class and provide comments marked with FIX to indicate where changes should be made Lets address them step by step Constructor in Golfer Class I...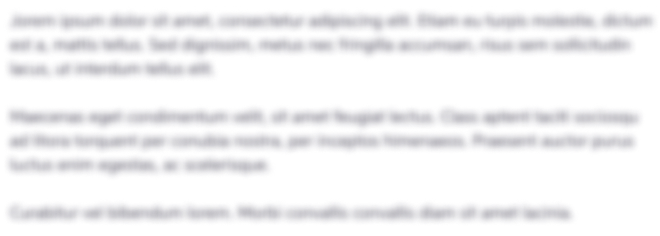
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started